Move an array element from one array position to another
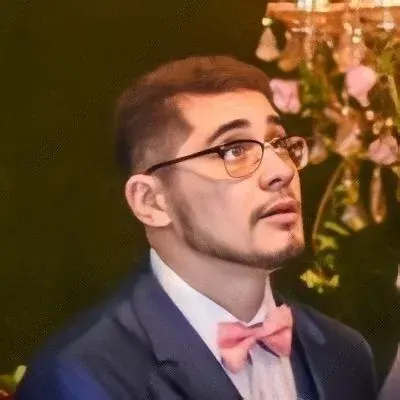
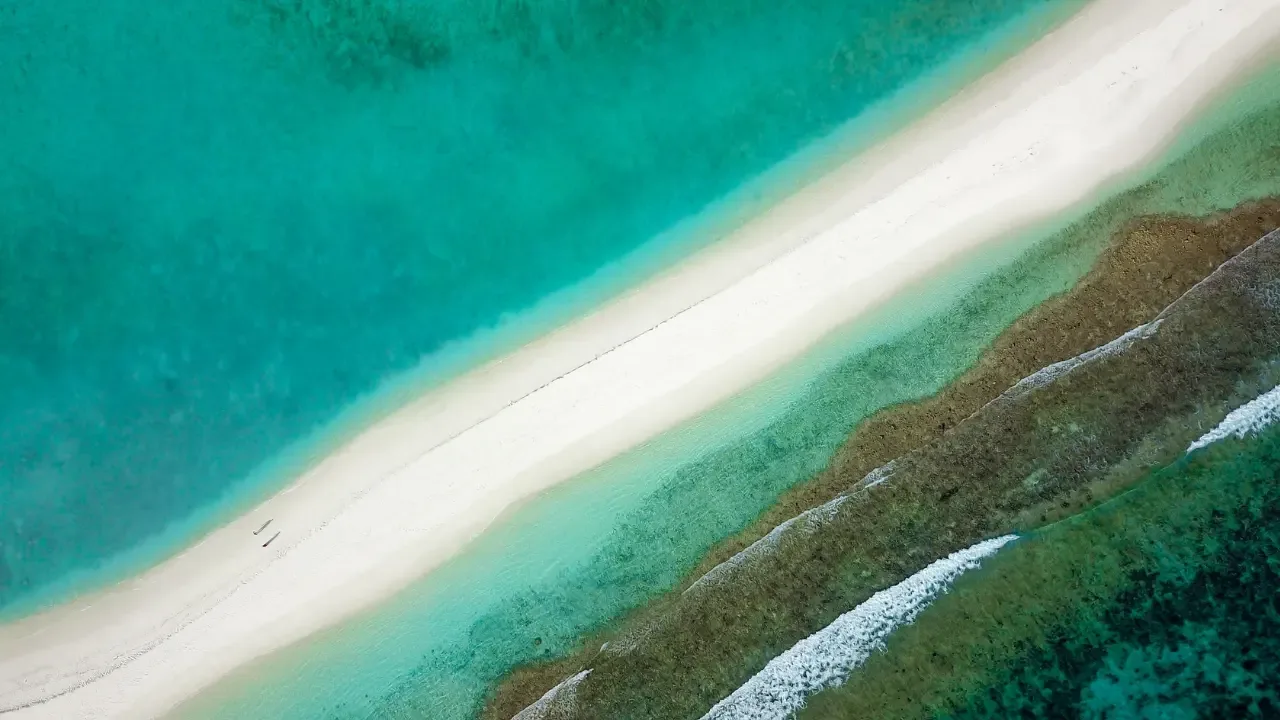
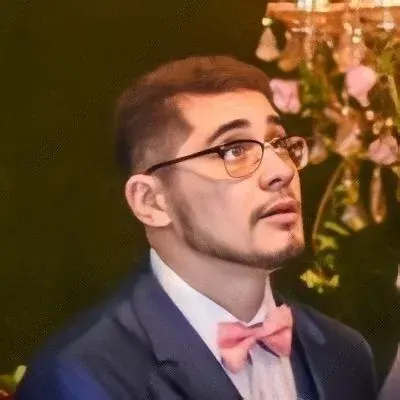
Moving Array Elements Like a Pro 💪
Are you ready to level up your programming skills and become a master of manipulating arrays? 🚀 In this blog post, we'll delve into the art of moving array elements from one position to another, effortlessly. 🎯
The Dilemma 💭
We empathize with you, fellow developer, when it comes to the struggles of moving array elements. 🤔 The scenario you described seems straightforward at first, but it can quickly become a head-scratcher. Thankfully, we're here to lend a helping hand. 💪
The Challenge 🎯
Let's start by acknowledging the problem: you need to move elements within an array, such as moving 'd'
to the left of 'b'
or shifting 'a'
to the right of 'c'
in your given array:
var array = ['a', 'b', 'c', 'd', 'e'];
Your desired outcome is a modified array where the element positions are updated accordingly:
array = ['a', 'd', 'b', 'c', 'e'];
Sounds tricky, right? But fear not! We have some nifty solutions for you. 🎉
The Solutions 💡
Solution 1: Good old-fashioned splice()
🙌
One way to tackle this challenge is by using the trusty splice()
function, which allows you to remove and insert elements in an array. Here's how you can apply it to move 'd'
to the left of 'b'
:
var indexOfD = array.indexOf('d');
var indexOfB = array.indexOf('b');
if (indexOfD !== -1 && indexOfB !== -1) {
array.splice(indexOfD, 1); // Remove 'd'
array.splice(indexOfB - 1, 0, 'd'); // Insert 'd' at the desired position
}
This code uses the indexOf()
function to find the positions of 'd'
and 'b'
within the array. Then, it removes 'd'
with splice()
, followed by inserting it into the desired position using another splice()
operation.
Solution 2: The concise reduce()
approach 🙌
If you're a fan of compact code, you might appreciate this elegant solution using the reduce()
function:
var moveElement = (arr, element, newPosition) => {
const index = arr.indexOf(element);
return arr.reduce(
(acc, curr, i) => acc.concat(i === newPosition ? [element, curr] : i === index ? [] : curr), []
);
}
array = moveElement(array, 'd', 1);
In this approach, we define a moveElement()
function that takes three parameters: the array, the element to move, and the new position. Using reduce()
, we iterate over the array and construct a new array with the desired element moved to the specified position.
Your Turn! ✨
Congrats on making it this far! Now it's time to put your newfound knowledge into practice. 🎉 Try applying these solutions to move other elements within an array, experiment, and share your results with the world. 🌍
Stay Curious 🤓
Moving array elements is just one of the many skills you can add to your programming toolbox. Keep exploring, stay curious, and never stop learning. If you have any questions or awesome ideas to share, feel free to leave a comment below. Let's grow together! 🌱
Happy coding! 🚀