Most efficient way to convert an HTMLCollection to an Array
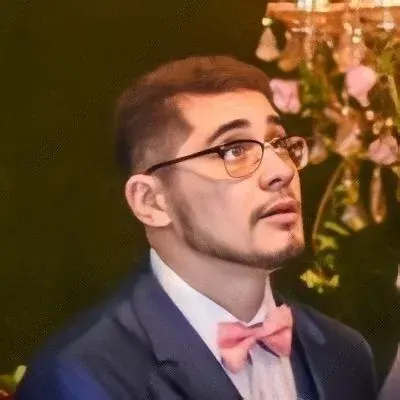
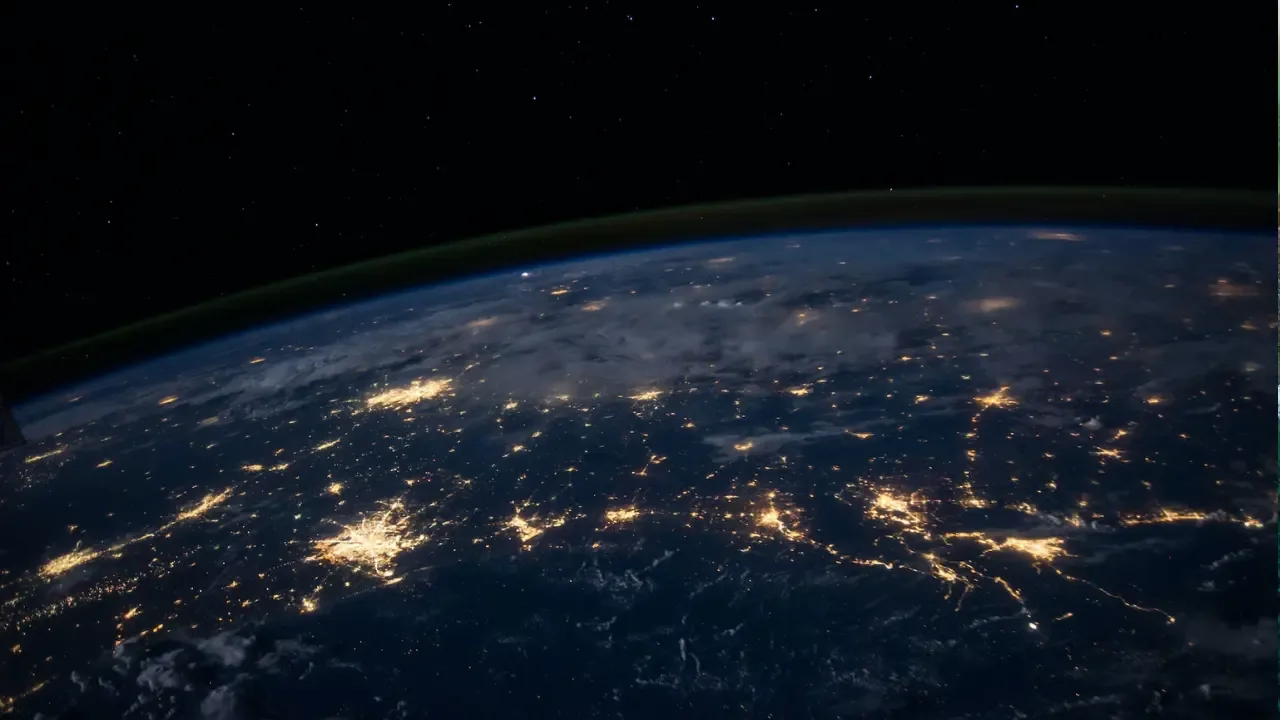
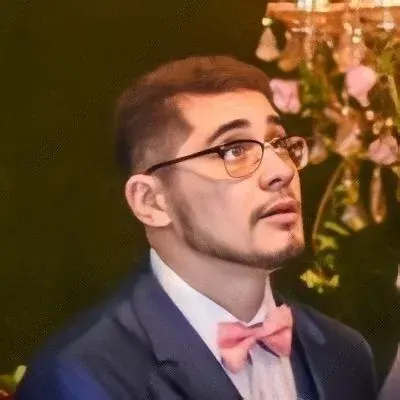
📝 Blog Post: Converting an HTMLCollection to an Array? Let's Do It Efficiently! 🚀
Hey there, tech enthusiasts! 👋 Have you ever wondered if there's a more efficient way to convert an HTMLCollection to an Array? 🤔 You're not alone! Many developers face this challenge when dealing with DOM manipulation. Well, sit back, relax, and let me show you some cool ways to tackle this problem! 🤓🚀
🔍 The Problem: Manual Iteration 😩
So, the common approach to converting an HTMLCollection to an Array is by manually iterating through the collection and pushing each item into the array. It works, but it can be a tedious and error-prone task, especially if the collection has a large number of items. 😫
✨ The Solution: Array.from() Method 🌟
Fear not, my friend! JavaScript provides us with a magical method called Array.from()
. 🪄 This method allows us to effortlessly convert an iterable object, like an HTMLCollection, into an Array in just one line of code. Let's see it in action:
const htmlCollection = document.getElementsByClassName('myClass'); // Replace 'myClass' with your desired CSS class
const myArray = Array.from(htmlCollection);
console.log(myArray); // Bask in the glory of your newly converted Array! 🎉
🎯 Instant Conversion, No Loops! 🔄
With Array.from()
, you'll get an Array filled with the contents of your HTMLCollection, without the need for any manual looping or pushing. It's a clean and efficient solution that saves you both time and frustration. 😌
💡 The Power of Spread Operator (...) 💪
If you prefer an even shorter syntax, you can use the spread operator (...
) in combination with Array.from()
. This technique spreads the iterable object into individual elements, creating a new Array:
const htmlCollection = document.getElementsByClassName('myClass');
const myArray = [...htmlCollection];
😎 How about Performance? ⚡
You might be wondering about the performance impact of these conversion methods. Well, worry not! Both Array.from()
and the spread operator leverage JavaScript's built-in optimizations, making them highly efficient. 🏎️💨
💥 Conclusion: Say Goodbye to Manual Conversion! 👋
There you have it, fellow devs! Converting an HTMLCollection to an Array can be a breeze with the power of Array.from()
or the spread operator. No more tedious loops or manual pushing! ⌛️⛏️
Now it's your turn! Give these methods a try, and let us know how they work for you. Do you have any other cool tricks up your sleeve? Share them in the comments below! Let's make our code more efficient together! 💪💬
Keep coding, keep innovating, and stay tuned for more helpful tips on our tech blog! 🌟✨