Most efficient method to groupby on an array of objects
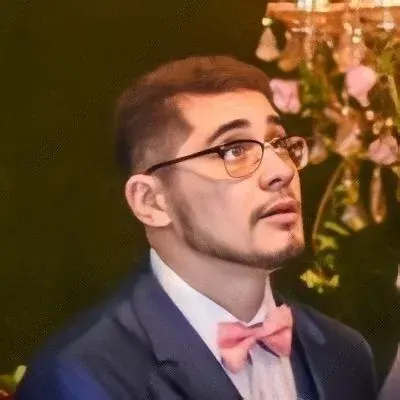
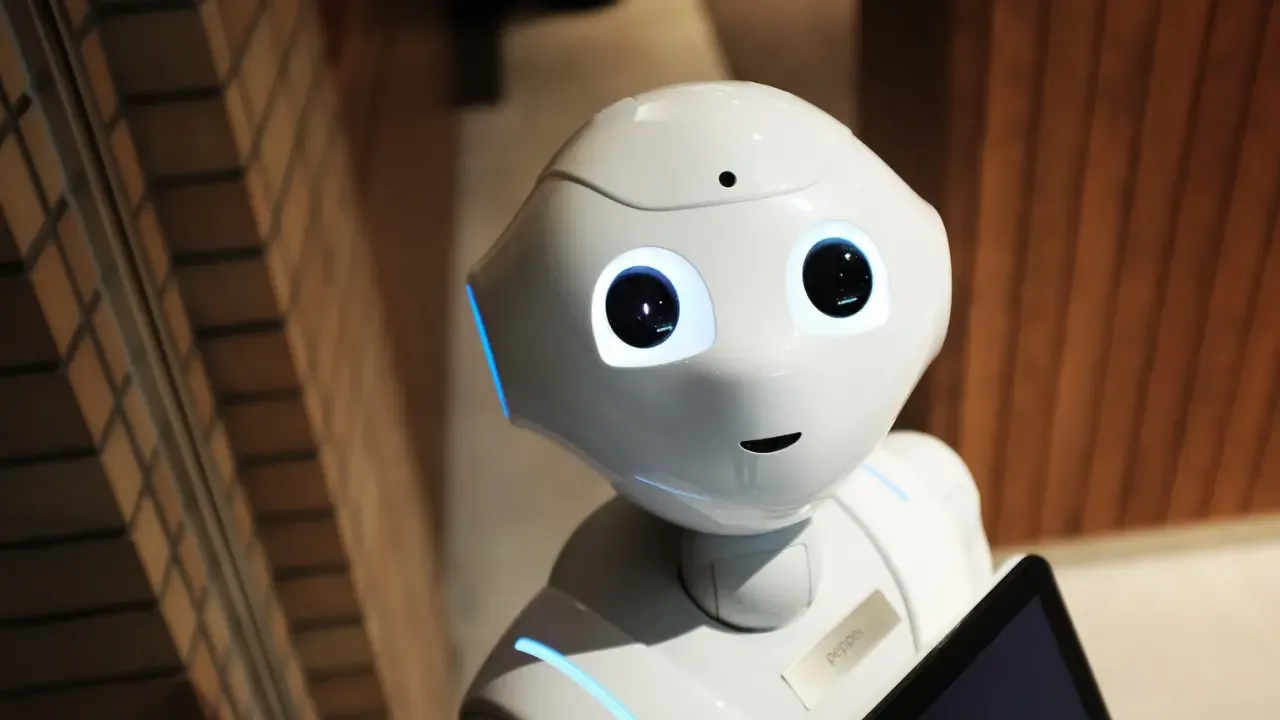
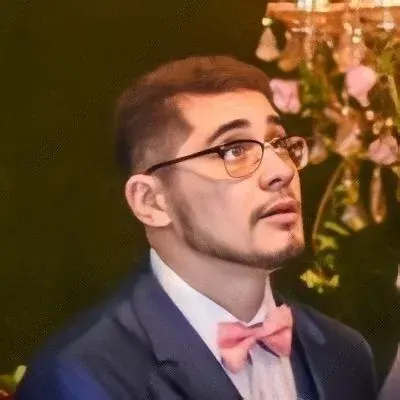
The Most Efficient Method to Groupby on an Array of Objects
Are you struggling with grouping objects in an array and summing their values efficiently? Look no further! In this blog post, we'll explore a common issue faced by developers and provide you with easy solutions to tackle it.
The Challenge
Consider the following array of objects:
[
{ Phase: "Phase 1", Step: "Step 1", Task: "Task 1", Value: "5" },
{ Phase: "Phase 1", Step: "Step 1", Task: "Task 2", Value: "10" },
{ Phase: "Phase 1", Step: "Step 2", Task: "Task 1", Value: "15" },
{ Phase: "Phase 1", Step: "Step 2", Task: "Task 2", Value: "20" },
{ Phase: "Phase 2", Step: "Step 1", Task: "Task 1", Value: "25" },
{ Phase: "Phase 2", Step: "Step 1", Task: "Task 2", Value: "30" },
{ Phase: "Phase 2", Step: "Step 2", Task: "Task 1", Value: "35" },
{ Phase: "Phase 2", Step: "Step 2", Task: "Task 2", Value: "40" }
]
The task at hand is to apply the groupby
operation to this array while aggregating the values for each group.
The Desired Output
Let's say we want to group the objects by the Phase
key, and we expect the following result:
[
{ Phase: "Phase 1", Value: 50 },
{ Phase: "Phase 2", Value: 130 }
]
If we want to group by both Phase
and Step
, the expected output would be:
[
{ Phase: "Phase 1", Step: "Step 1", Value: 15 },
{ Phase: "Phase 1", Step: "Step 2", Value: 35 },
{ Phase: "Phase 2", Step: "Step 1", Value: 55 },
{ Phase: "Phase 2", Step: "Step 2", Value: 75 }
]
The Solution
While Underscore.js offers a groupby
function, it doesn't provide an out-of-the-box solution to perform the desired aggregation. However, fear not! We've got you covered with an efficient solution using JavaScript and Underscore.js.
const array = [
// Array of objects
];
const result = _(array)
.groupBy('Phase')
.map((group, Phase) => {
return {
Phase: Phase,
Value: _.sumBy(group, 'Value')
};
})
.value();
In the above code snippet, we first use groupBy
to group the objects based on the Phase
key. Then, using map
, we iterate over each group, calculate the sum of the Value
property using sumBy
, and finally map it to the desired output format.
Taking It Further
If you require additional levels of grouping, such as by both Phase
and Step
, you can modify the code accordingly:
const result = _(array)
.groupBy(obj => obj.Phase + obj.Step)
.map((group, key) => {
const [Phase, Step] = key.split(' ');
return {
Phase: Phase,
Step: Step,
Value: _.sumBy(group, 'Value')
};
})
.value();
Your Call-to-Action
Now that you have a solution at your fingertips, give it a try and optimize your code for efficient grouping and aggregation of objects in an array! Share your experience or any alternative solutions in the comments below. Happy coding! 💻🚀
Note: This blog post provides a specific solution using Underscore.js, but alternative libraries like Lodash can also be used with similar approaches.