Moment js date time comparison
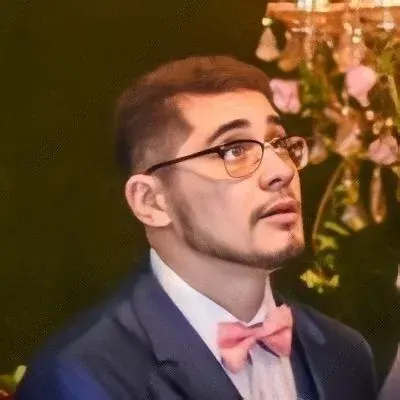
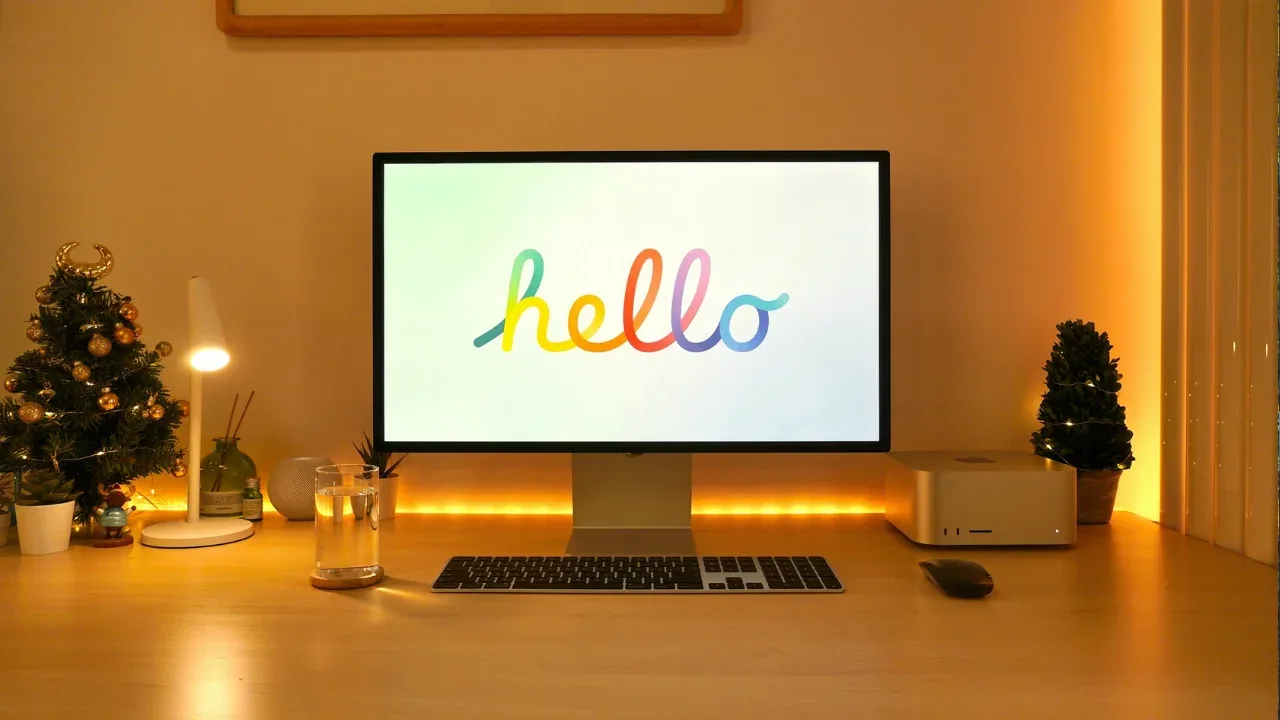
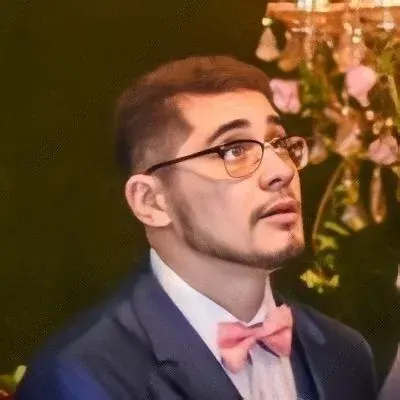
Comparing Date and Time using Moment.js
If you're working with date and time in JavaScript and want to compare them, Moment.js is a popular library that offers extensive functionality for parsing, manipulating, and formatting dates and times. However, when it comes to comparing dates and times with Moment.js, you might encounter some challenges.
The Problem
In the given code snippet, the user is trying to compare two date-time values using Moment.js. They are expecting the first date-time to be greater than the second one. However, the code always goes to the else
condition, suggesting that the comparison is not working as expected.
The Solution
To correctly compare date and time in Moment.js, you can use the isAfter()
function. This function checks if a given date is after another date.
Here's an example of how to modify the code to achieve the desired comparison:
var date_time = req.body.date + 'T' + req.body.time + req.body.timezone;
var utc_input_time = moment(date_time).utc();
console.log('utc converted date_time', moment(date_time).utc().format("YYYY-MM-DDTHH:mm:SSS"));
var isafter = moment(utc_input_time).isAfter(moment('2014-03-24T01:14:000'));
if(isafter === true){
console.log('is after true');
} else {
console.log('is after is false');
}
In this updated code, we are using the isAfter()
function to compare the utc_input_time
with the specified date and time.
Explanation
The issue in the original code might be due to improper formatting or incorrect usage of the isAfter()
function. By comparing the utc_input_time
with the specified date and time in the right format, you can now accurately determine if the first date-time is after the second one.
Your Turn
Now it's your turn to apply this knowledge to your projects and start comparing date and time using Moment.js. Make sure to follow the correct formatting for your dates and use the appropriate comparison functions like isAfter()
to get accurate results.
Feel free to share your experiences or any further questions in the comments section below! Happy coding! 💻🎉📅