module.exports vs exports in Node.js
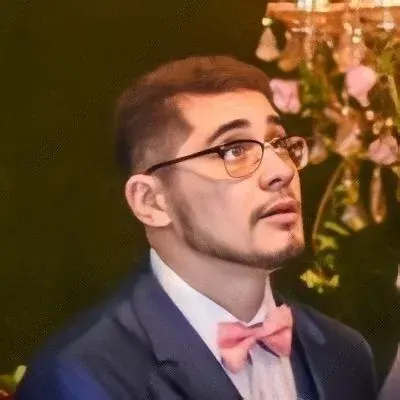
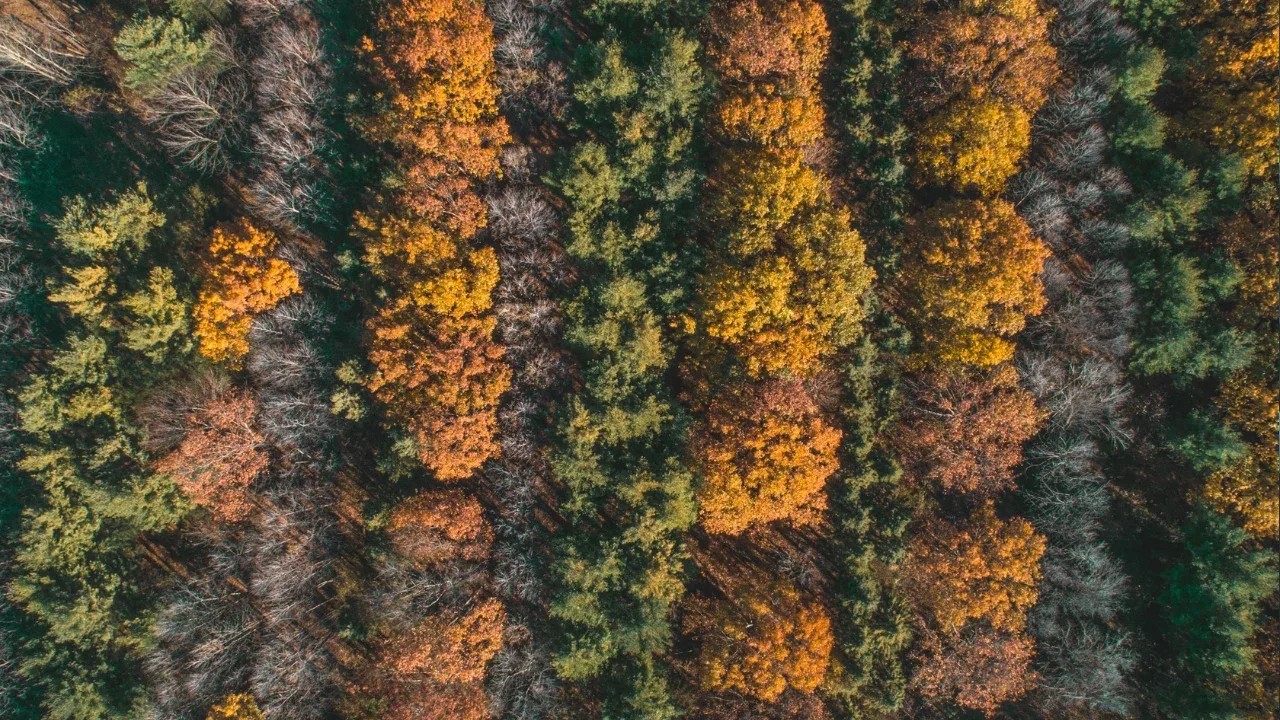
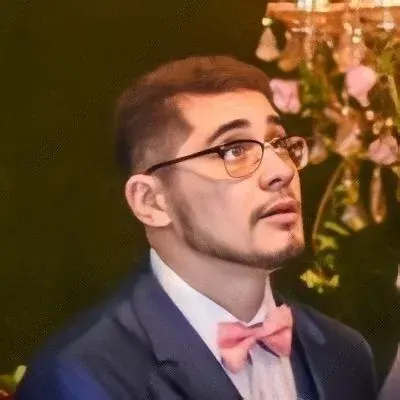
Understanding module.exports vs exports in Node.js 📦🤔
When working with Node.js modules, you may come across the terms module.exports
and exports
. 🤷♂️ While they both serve a similar purpose of exporting values from a module, there are some key differences that you need to be aware of. Let's dive into the details! 🚀
The Basics of Exporting in Node.js 📨📨
In Node.js, modules play a crucial role in organizing and sharing code. Exporting values from a module allows other parts of your application to access and use those values. By default, each module has an exports
object, which is initially an empty object.
Here's a simple example to illustrate this:
// math.js
exports.add = function(a, b) {
return a + b;
};
exports.multiply = function(a, b) {
return a * b;
};
// app.js
const math = require('./math.js');
console.log(math.add(2, 3)); // Output: 5
console.log(math.multiply(2, 3)); // Output: 6
In the above example, we are exporting two functions (add
and multiply
) from the math.js
module. In the app.js
file, we require the math.js
module using the require
function and can then access the exported functions using the math
object.
Understanding module.exports 📥
While exports
provides a convenient way to export values, it has its limitations. If you attempt to assign a new value directly to exports
, it will break the reference between the module and the original exports
object. 😱 This means any properties you add to exports
after that assignment will not be accessible to other modules.
This is where module.exports
comes into play. Think of module.exports
as the actual object that is exported from the module. By default, module.exports
points to the same object as exports
. However, you can reassign module.exports
to a different value or object.
Let's take a look at an example illustrating the difference:
// greetings.js
module.exports = {
sayHello: function() {
return "Hello!";
}
};
// app.js
const greetings = require('./greetings.js');
console.log(greetings.sayHello()); // Output: Hello!
console.log(greetings.howAreYou()); // TypeError: greetings.howAreYou is not a function
In the above example, we export an object with the sayHello
method using module.exports
. If we had used exports
instead, the sayHello
method would have been inaccessible.
Combining module.exports and exports in Advanced Scenarios 🔄
Occasionally, you may encounter scenarios in which you want to export an object with several properties while also assigning a single function or variable to be the main export. In such cases, you can use a combination of module.exports
and exports
.
Take a look at this example:
// utils.js
exports.isValid = function(str) {
// implementation...
};
module.exports = function specialFunction() {
// implementation...
};
// app.js
const utils = require('./utils.js');
console.log(utils.isValid("Hello")); // Output: true
console.log(utils("Something")); // Output: Special function invoked!
By combining module.exports
and exports
, we can export both the isValid
function and the specialFunction
as separate entities that can be used in other modules.
Conclusion and Your Call-to-Action! 🏁📣
Understanding the differences between module.exports
and exports
in Node.js is essential to optimize your code organization and module exports. Remember:
exports
is a reference tomodule.exports
and allows adding properties to be exported.module.exports
is the actual object that is exported from the module. It can be reassigned to a different value or object.
Now that you have a better understanding of module.exports
vs exports
, put this knowledge into practice in your Node.js projects! 🚀 Feel free to share your thoughts and experiences in the comments below. Happy coding! 💻❤️