"Mixed content blocked" when running an HTTP AJAX operation in an HTTPS page
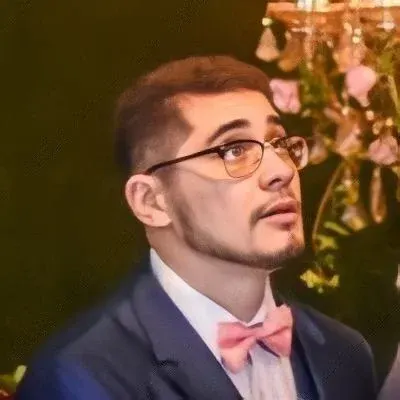
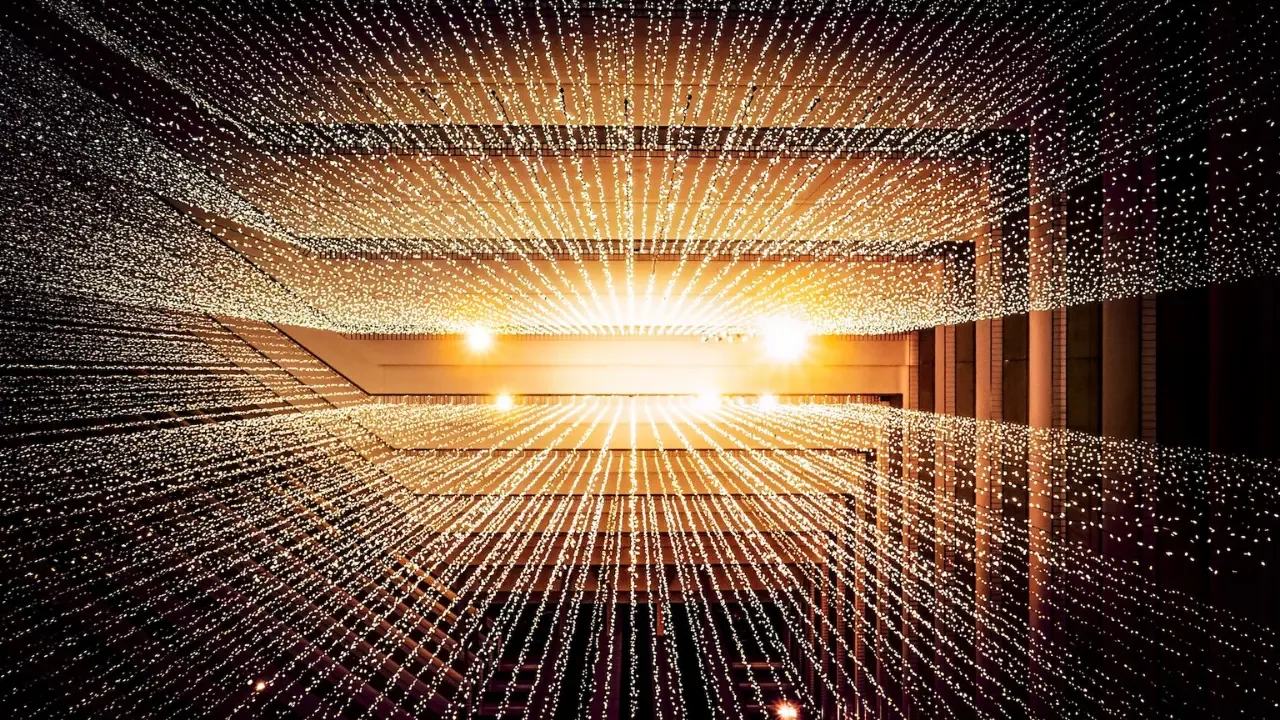
💡 Solving the "Mixed content blocked" problem when running an HTTP AJAX operation in an HTTPS page
Have you ever come across the pesky "Mixed content blocked" error while trying to run an HTTP AJAX operation in an HTTPS page? Trust me, you're not alone! 🙅♂️
Picture this: You have a form on your website, and upon submission, you want to display a thank-you page. Simple, right? But what if you encounter the dreaded "Mixed content blocked" error? 😱
Understanding the "Mixed content blocked" error
The error message generally looks something like this:
Mixed Content: The page at 'https://page.com' was loaded over HTTPS, but requested an insecure XMLHttpRequest endpoint 'http://XX.XXX.XX.XXX/vicidial/non_agent_api.php?queries=query=data'. This request has been blocked; the content must be served over HTTPS.
🔎 The root cause of this issue is mixed content: loading a secure webpage (HTTPS) that attempts to retrieve content from an insecure source (HTTP).
The AJAX script conundrum
Let's take a closer look at the AJAX script mentioned in the context of the problem:
<script>
SubmitFormClickToCall = function(){
jQuery.ajax({
url: "http://XX.XXX.XX.XX/vicidial/non_agent_api.php",
data: jQuery("#form-click-to-call").serialize(),
type: "GET",
processData: false,
contentType: false,
success: function(data){
window.location.href = "https://www.example.com/thank-you";
}
});
}
</script>
The issue here is that the API (http://XX.XXX.XX.XX/vicidial/non_agent_api.php
) is being called over HTTP, while your page is loaded over HTTPS, resulting in a mixed content block.
Easy solution to the mixed content problem
Now, if you can't change the API to HTTPS (which is often the case), we can work around this issue:
Instead of using AJAX to submit the form, let's create a server-side PHP file (let's call it
submit_form.php
) that receives the submitted data.In
submit_form.php
, we can send the data to the API using a server-side mechanism like cURL. Don't forget to sanitize and validate the data before sending it!Here's an example of how we can use cURL to send a GET request to the API:
<?php $apiUrl = "http://XX.XXX.XX.XX/vicidial/non_agent_api.php"; $queryParams = $_GET; // Assuming your form data is sent via GET // Create the cURL request $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $apiUrl . '?' . http_build_query($queryParams)); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // Execute the request and fetch the response $response = curl_exec($ch); curl_close($ch); // Redirect the user to the thank-you page header("Location: https://www.example.com/thank-you"); exit(); ?>
Modify your JavaScript code to use the
submit_form.php
file instead of making a direct AJAX call. Here's how:<script> SubmitFormClickToCall = function(){ var form = jQuery("#form-click-to-call"); var formData = new FormData(form[0]); // Submit the form to submit_form.php using AJAX jQuery.ajax({ url: "/path/to/submit_form.php", data : formData, type : "GET", processData: false, contentType: false, success: function(data){ window.location.href = "https://www.example.com/thank-you"; } }); } </script>
Voila! With this approach, your AJAX request is made to a secure (HTTPS) endpoint (
submit_form.php
) rather than the insecure API, avoiding the mixed content block!
🚀 Let's tackle this problem together!
If you're facing the "Mixed content blocked" error while running an HTTP AJAX operation in an HTTPS page, fear not! Simply follow the steps outlined above, and your form submission and redirect to the thank-you page will work seamlessly. 🎉
So, what are you waiting for? Get that form submission working flawlessly and make your users feel appreciated with a fancy thank-you page!
If you found this guide helpful or have any questions, feel free to leave a comment below and let's discuss! Happy coding, amigos! ✌️
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
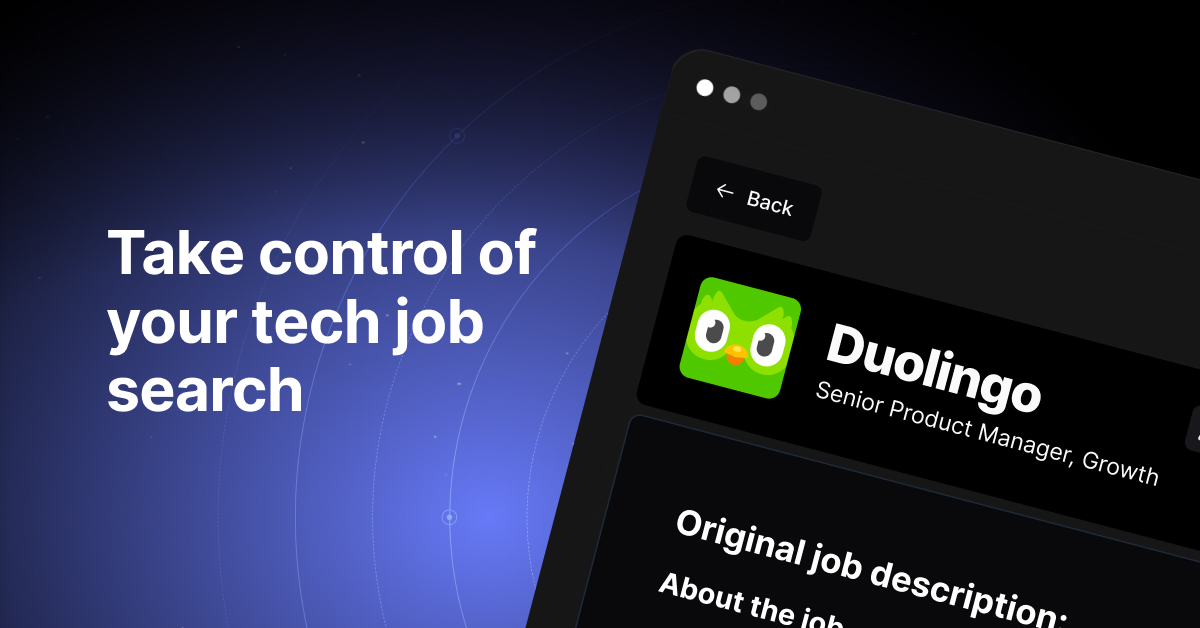