Merge/flatten an array of arrays
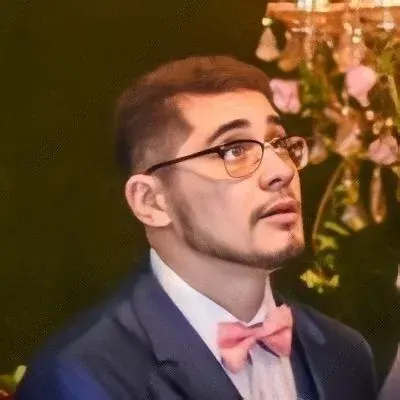
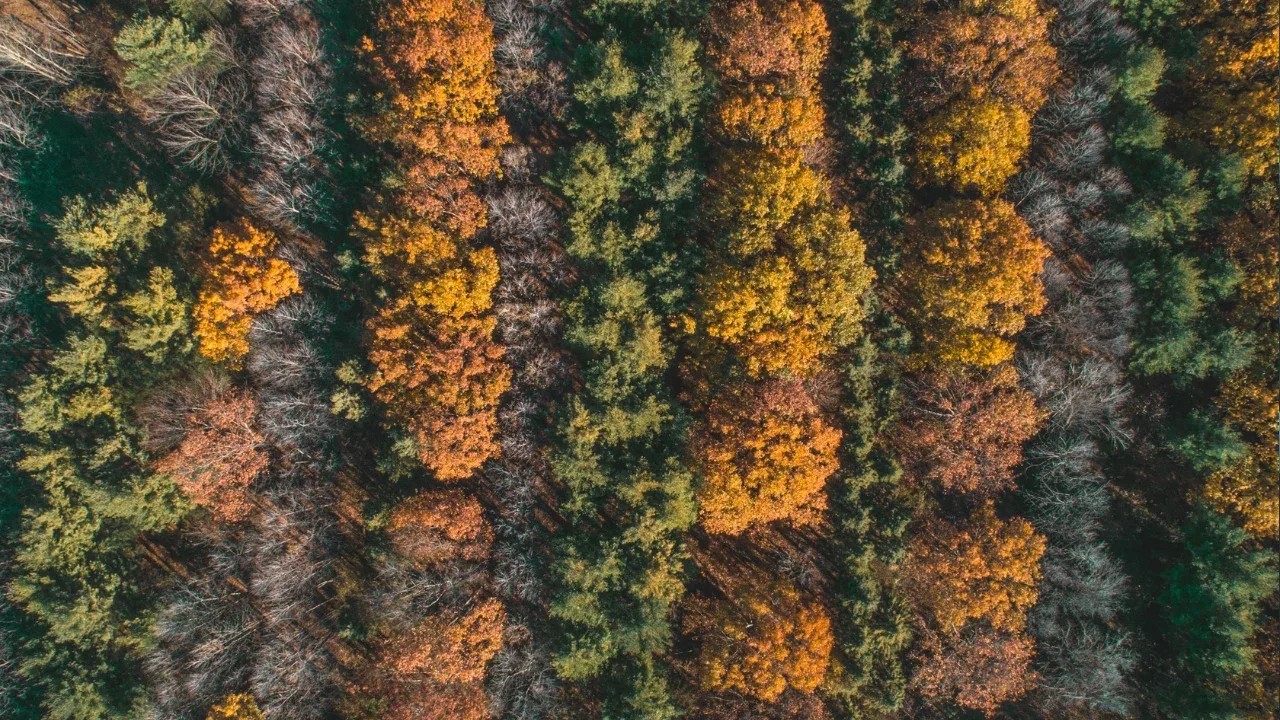
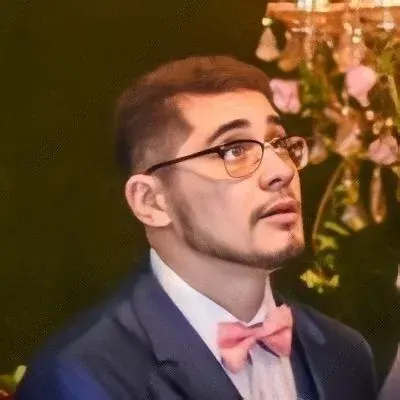
📝 Title: "Merge and Flatten an Array of Arrays in JavaScript: Simplified Guide with Easy Solutions! 💪"
Introduction: Hey there, tech enthusiasts! 🤓 Welcome to another exciting blog post where we unravel the mysteries of JavaScript. Today, we are going to address a common issue programmers encounter: merging and flattening an array of arrays. 🤔 Fear not, fellow coders, as we have easy solutions that will make your coding life a breeze! Let's dive right into it! 🚀
The Problem: So, you have an array of arrays, huh? Here's an example to illustrate the issue:
[["$6"], ["$12"], ["$25"], ["$25"], ["$18"], ["$22"], ["$10"]]
Your goal is to merge the separate inner arrays into one, like this:
["$6", "$12", "$25", ...]
Don't worry; we've got you covered! 🛡️
Solution 1: The concat
Method 🗂️
The easiest way to merge and flatten an array of arrays is to use the trusty concat
method available in JavaScript. Let me show you how it works:
const arrayOfArrays = [["$6"], ["$12"], ["$25"], ["$25"], ["$18"], ["$22"], ["$10"]];
const flattenedArray = [].concat(...arrayOfArrays);
console.log(flattenedArray); // Output: ["$6", "$12", "$25", ...]
The ...
spread operator allows us to spread the inner arrays inside the concat
method, resulting in a single, merged array. Voilà! 🌟
Solution 2: The reduce
Method 📊
For those seeking a more elegant and functional approach, the reduce
method comes to the rescue! Here's how you can use it:
const arrayOfArrays = [["$6"], ["$12"], ["$25"], ["$25"], ["$18"], ["$22"], ["$10"]];
const flattenedArray = arrayOfArrays.reduce((accumulator, currentArray) => accumulator.concat(currentArray), []);
console.log(flattenedArray); // Output: ["$6", "$12", "$25", ...]
By using reduce
, we iterate over each inner array and merge them into the accumulator, starting with an empty array []
. The result is our desired flattened array! 🎉
Call-to-Action:
There you have it, folks! Two simple solutions to merge and flatten an array of arrays in JavaScript. Try them out in your next project and save yourself time and headaches. 😎
If you found this blog post helpful, share it with your fellow developers 🌍 to spread the knowledge. 💡 Also, don't hesitate to leave a comment below if you have any questions or suggestions for future topics. Happy coding! 💻🔥