Maximum call stack size exceeded error
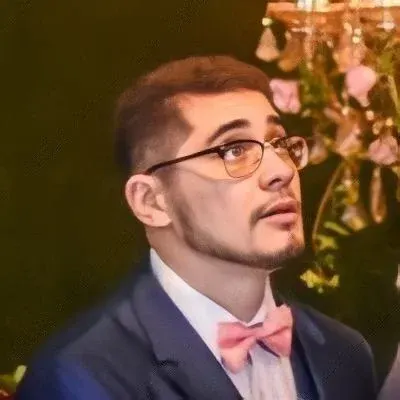
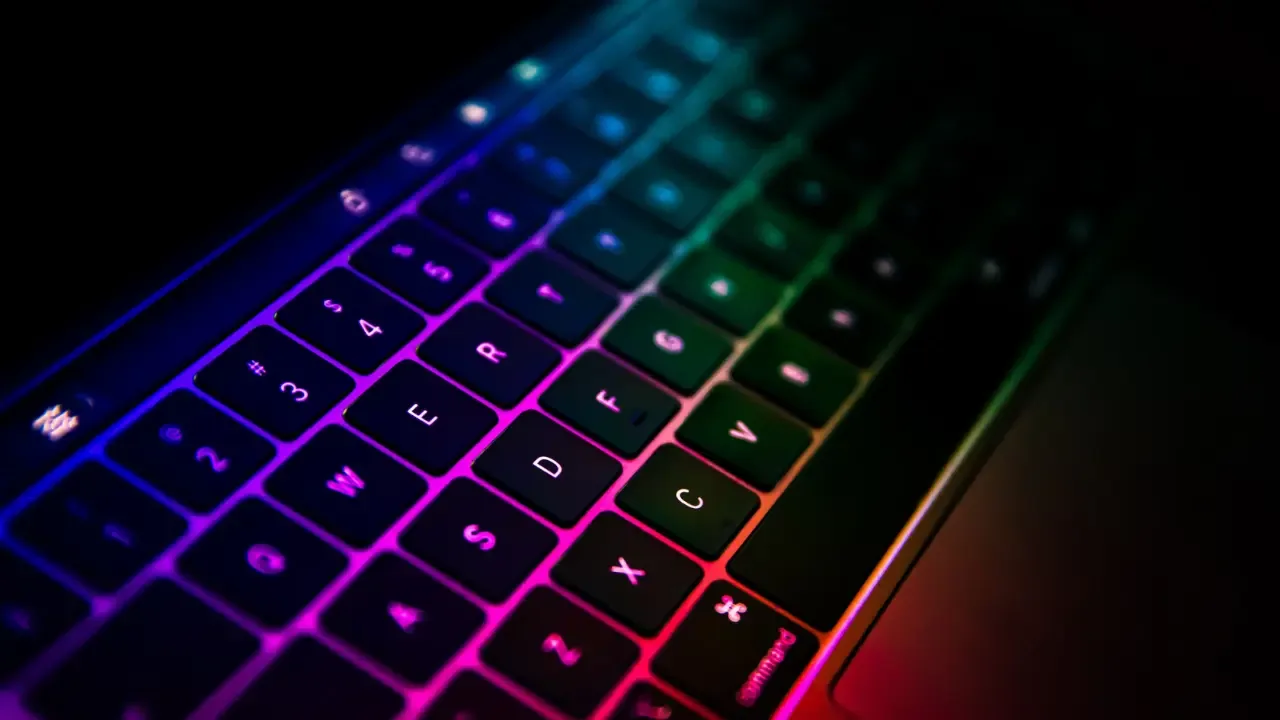
Understanding the "Maximum call stack size exceeded" Error đđĨ
Have you ever encountered the infamous "Maximum call stack size exceeded" error while working with JavaScript? đ¤ If you have, you know how frustrating and perplexing it can be. But fear not! In this blog post, we'll unravel the mysteries behind this error and provide you with easy-to-implement solutions. So, let's dive right in! đââī¸đ
What does this error mean? â
This error message is thrown when a recursive function or loop consumes more stack space than what is available. The call stack is a data structure used by the JavaScript engine to keep track of function calls. Each time a function is called, a new frame is added to the call stack. When a function completes execution, its frame is removed from the stack.
However, if a function repeatedly calls itself or another function without terminating, the call stack keeps growing until it exceeds its maximum limit. At this point, JavaScript throws the "Maximum call stack size exceeded" error. đą
Does it stop processing completely? đĢâšī¸
Yes, when this error occurs, it brings the JavaScript execution to a screeching halt. It's like a traffic jam on the information highway! đ§đđĨ All further code execution stops, and the error is thrown, making it impossible for your program to continue running.
Fixing the issue in Safari (and other browsers) đĻđ
To resolve this error, you need to identify the part of your code that is causing the infinite function calls or loop. Here are a few common scenarios and their solutions:
1. Recursive functions gone wild đ
If you're using a recursive function, double-check that you have a proper base case that ends the recursion. If your base case is missing or incorrect, your function will keep calling itself endlessly.
function countdown(number) {
if (number <= 0) {
return;
}
console.log("T-minus " + number);
countdown(number - 1);
}
In the above example, the base case is when number
becomes less than or equal to zero. Without it, the countdown function would keep calling itself until the maximum stack size is exceeded.
2. Endless loops đ
Similarly, make sure you don't have an infinite loop in your code. Check for conditions that will eventually allow the loop to break.
let counter = 0;
while (counter < 10) {
console.log("Loop iteration: " + counter);
counter++; // Make sure the loop condition is eventually false
}
In this case, the loop will keep incrementing the counter
variable until it reaches 10, at which point the loop will terminate.
3. Stack-consuming data structures đī¸
If you're using a data structure like a stack or a queue that relies on recursive functions for operations, ensure that you're not inadvertently causing infinite recursion.
Safari-specific "JS:execution exceeded timeout" error â°đĻ
You also mentioned encountering a "JS:execution exceeded timeout" error specifically in Safari on iPad. This error is often a consequence of the same call stack issue we discussed earlier. The difference is that Safari reports it in a different way, emphasizing the timeout aspect.
To address this error, you can try the following steps:
Optimize your code and minimize complex operations that may cause the timeout to be exceeded.
Break your code into smaller chunks or refactor it to avoid long-running operations.
Consider using asynchronous programming techniques such as Promises or async/await to avoid blocking the JavaScript event loop.
Conclusion and Call-to-Action đđŖ
Understanding and fixing the "Maximum call stack size exceeded" error is crucial for JavaScript developers. By identifying the root cause and implementing the appropriate solutions, we can prevent our programs from getting stuck in an infinite loop or recursive madness! đĩđ
If you found this blog post helpful, share it with your fellow developers encountering stack-related mishaps. And don't hesitate to leave a comment below if you have any questions or need further assistance. Happy coding! đģâ¨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
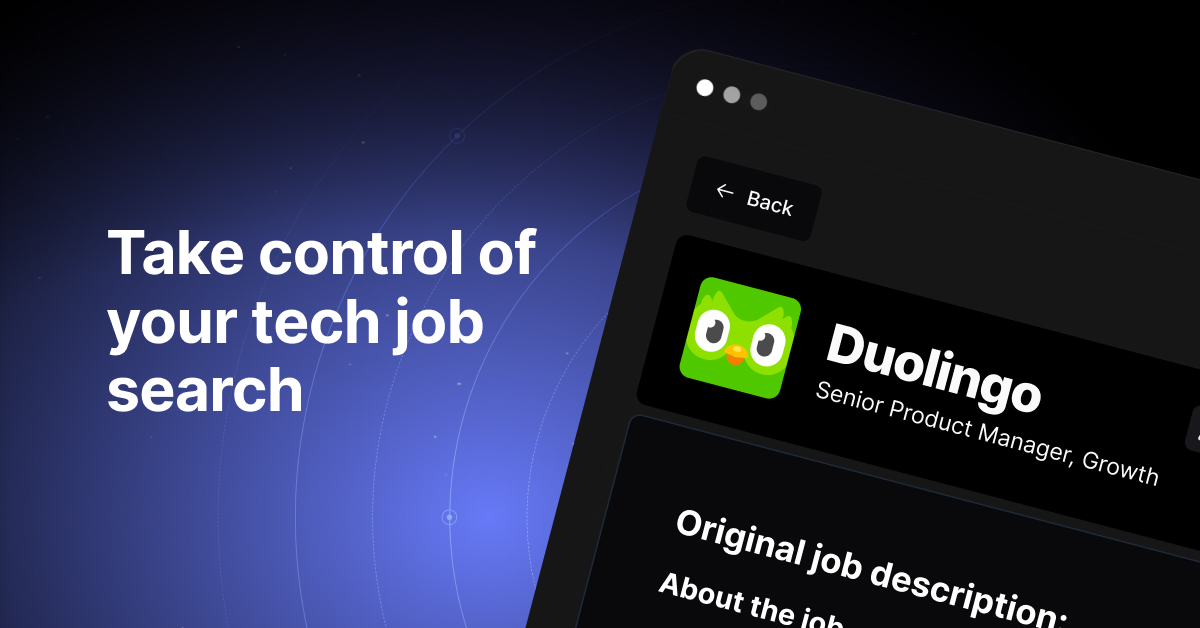