map function for objects (instead of arrays)
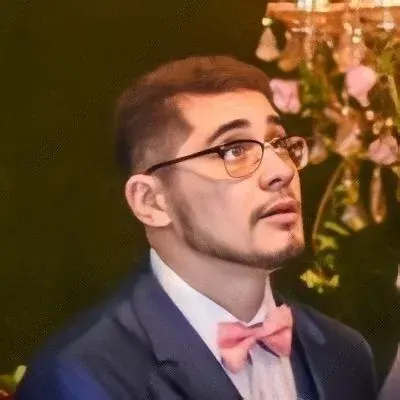
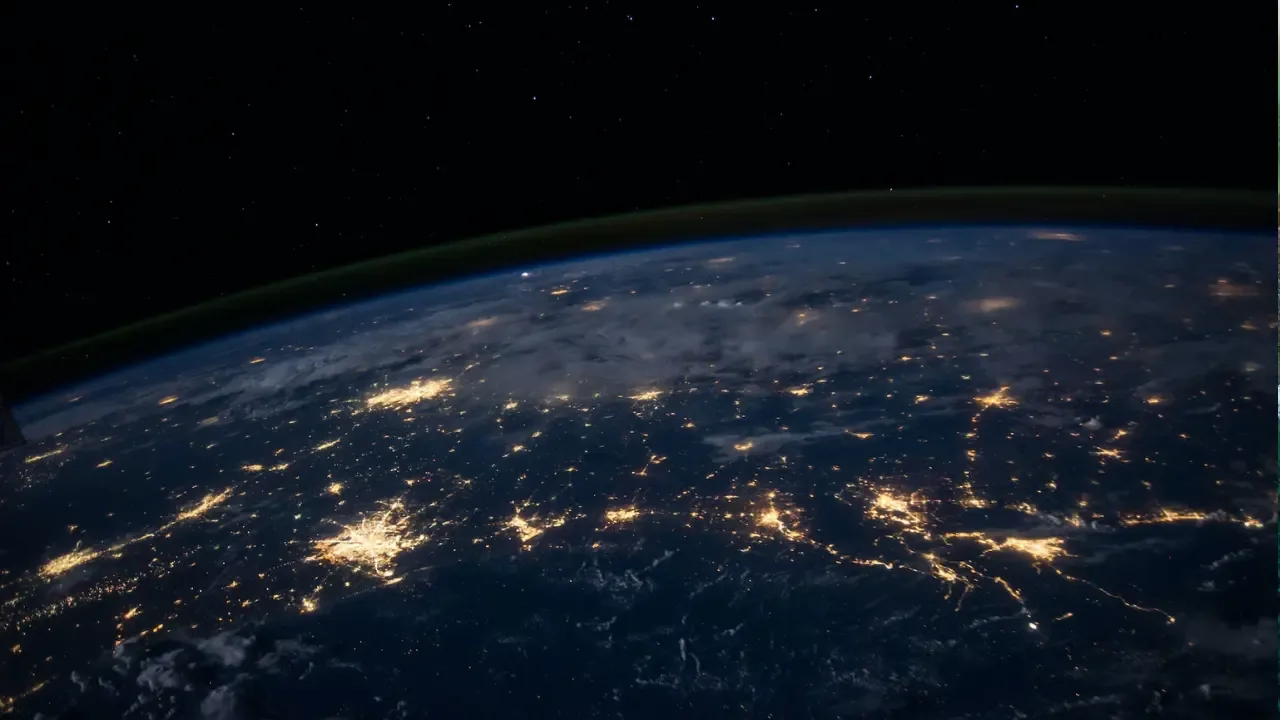
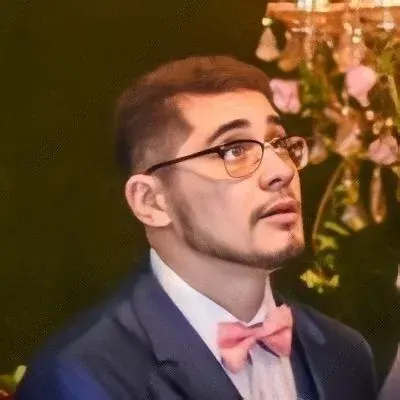
Mapping Objects in JavaScript: No More FOMO!
π Hey there tech enthusiasts! π₯οΈπ Today, we're diving into a fascinating topic: mapping objects in JavaScript! ππΊοΈ Have you ever wondered if there is a native method, just like Array.prototype.map
, that would work for objects instead of arrays? π€ Well, keep reading to find out! πβ¨
The Problem at Hand
Let's set the stage. Imagine you have an object called myObject
, and you want to perform some operations on its values while maintaining the structure. Consider the following example:
myObject = { 'a': 1, 'b': 2, 'c': 3 }
Now, with the power of Array.prototype.map
, we might wish to update each value by squaring it. Ideally, we would use the same approach we'd use for arrays:
newObject = myObject.map(function (value, label) {
return value * value;
});
But hang on a second! There's no such map
function for objects in JavaScript! π± So, what can we do about it? Let's find some easy-peasy lemon squeezy solutions! ππ§
Solution 1: Looping with 'for...in'
One way to map an object is by using a classic loop with the for...in
statement. This allows us to iterate over the properties of an object. π Here's a code snippet to illustrate this method:
newObject = {};
for (const key in myObject) {
if (myObject.hasOwnProperty(key)) {
newObject[key] = myObject[key] * myObject[key];
}
}
By iterating through each key in myObject
and accessing its corresponding value, we can perform any desired operation and store the updated values in a new object.
Solution 2: Utilizing 'Object.entries' and 'reduce'
Feeling like getting rid of the cumbersome for...in
loop? We got you! π Another elegant solution involves using two JavaScript methods: Object.entries
and reduce
. Let me demonstrate:
newObject = Object.entries(myObject).reduce((acc, [key, value]) => ({
...acc,
[key]: value * value,
}), {});
Here, Object.entries
creates an array whose elements are subarrays consisting of a key-value pair. We then apply reduce
on this array to build a new object. Pretty neat, huh? πββοΈ
Call to Action: Share Your Thoughts!
And there you have it, folks! Two simple yet powerful solutions for mapping objects in JavaScript. π Now it's your turn! Have you ever had to deal with similar challenges? Which solution do you prefer? Let us know in the comments below! π¬β¨
Don't keep all this newfound knowledge to yourself! Share this blog post with your fellow developers and make their coding lives easier! πβοΈ
Stay curious, keep coding! π¨βπ»π‘