Loop through an array in JavaScript
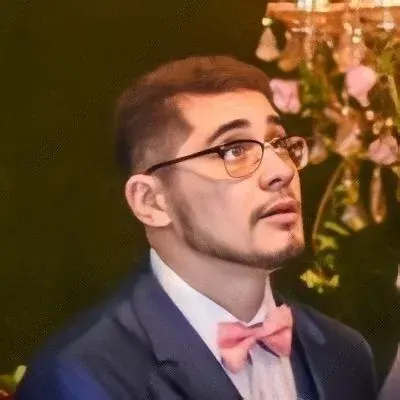
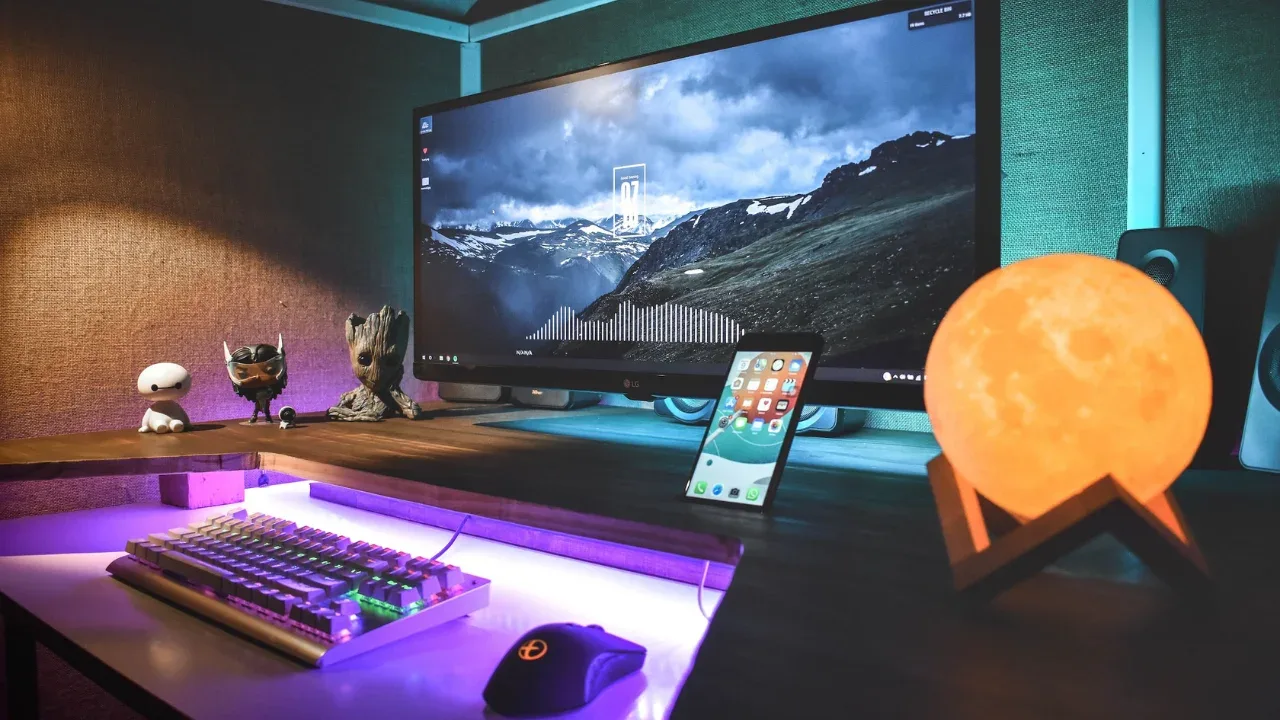
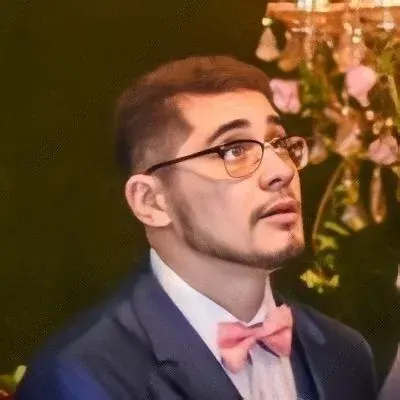
๐๐ Loop Through an Array in JavaScript: Unleash the Power! ๐
Are you tired of getting stuck when it comes to looping through arrays in JavaScript? ๐ฉ Fear not, for I am here to guide you through this mind-boggling challenge! ๐
๐ค So, the burning question is: Can you loop through an array in JavaScript just like you can in Java using a for
loop? ๐คจ Let's find out!
In JavaScript, you have a variety of options to iterate through arrays. One common method is the good ol' for
loop. ๐ช Allow me to enlighten you with some code snippets! ๐
const myStringArray = ["Hello", "World"];
for (let i = 0; i < myStringArray.length; i++) {
// Do something with myStringArray[i]
}
๐ก In the example above, the for
loop starts by initializing a variable i
to 0, as the first element of an array has an index of 0. The loop runs as long as i
is less than the length of the array (myStringArray.length
). After each iteration, the i
variable is incremented by 1.
๐ Oh, but there's more! JavaScript also offers elegant alternatives, such as the mighty forEach
method. ๐ Allow me to introduce you to JavaScript's fancy side! ๐ฉ
const myStringArray = ["Hello", "World"];
myStringArray.forEach((element) => {
// Do something with element
});
๐ฅ The forEach
method is like a breeze of fresh air! It takes a function as an argument and applies that function to each element of the array. So, the function element
represents each individual element in the array as it is iterated. Isn't that cool? ๐
Now that you've conquered looping through arrays in JavaScript, it's time to take your skills to the next level! ๐ฏ Put your newfound knowledge into action and transform your code to its full potential, my dear reader. ๐
But wait, there's one more thing! ๐ I want to hear about your experiences with array looping in JavaScript. Comment below ๐ and share your ingenious solutions to this common challenge. Together, we can conquer any coding obstacle that stands in our way! ๐ช๐ป
Happy coding! ๐๐