Loop inside React JSX
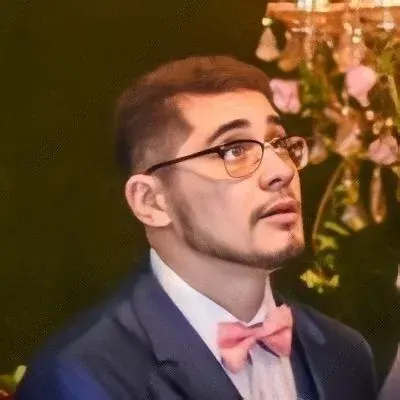
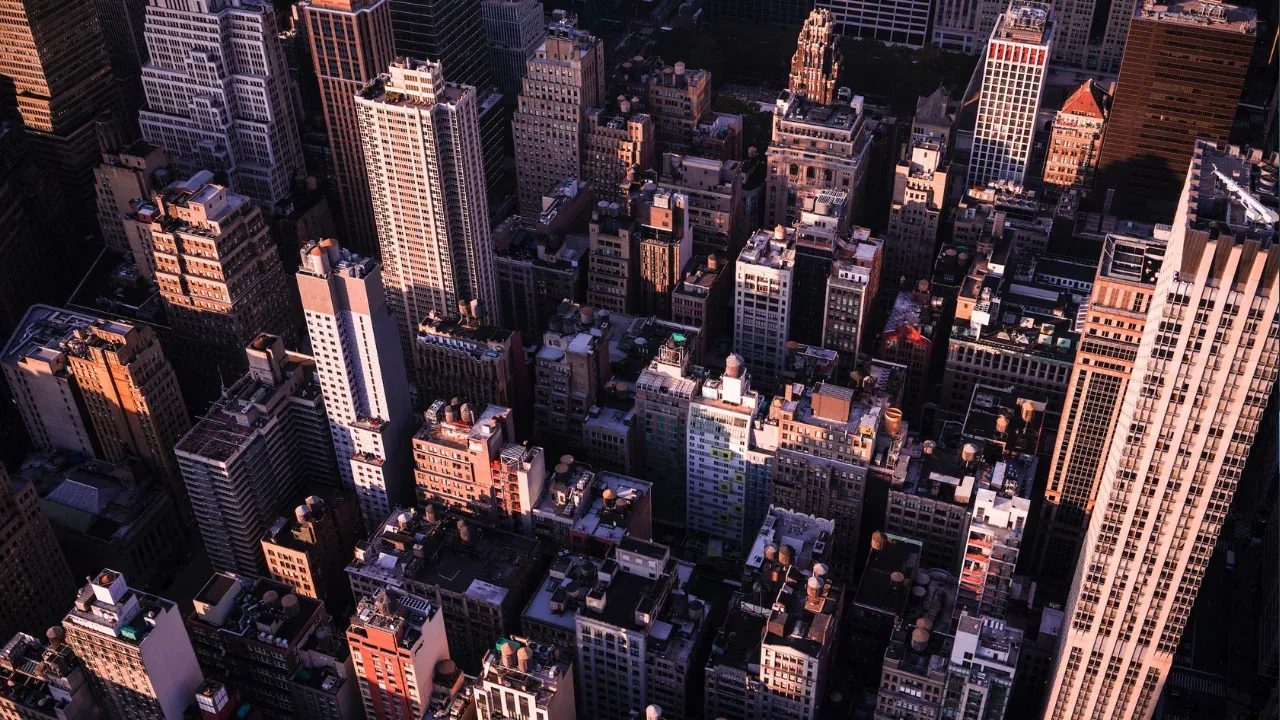
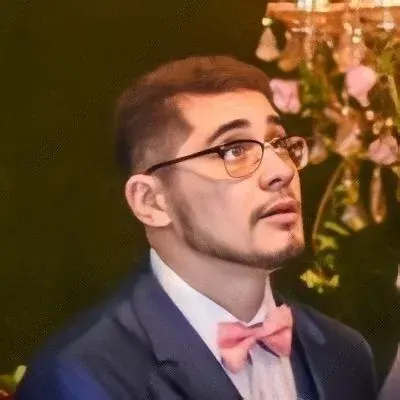
Blog Post: Loop Inside React JSX 🔄
Hey there! 👋 Are you trying to create a loop inside your React JSX code? You're not alone! Many developers coming from a template background find themselves unsure of how to achieve this in JSX. But fear not! In this blog post, we'll dive into the common issues and provide easy solutions to loop inside React JSX. Let's get started! 💪
The Challenge 🤔
Here's the scenario: you want to iterate over a collection or repeat a component multiple times using a loop inside your React JSX code. However, you quickly realize that the traditional for loop syntax doesn't work in JSX as it maps to function calls. So, how do you achieve this?
The Solution 💡
Don't worry, we've got you covered! There are a few ways to loop inside React JSX. Let's explore the most common and easy-to-understand solutions:
1. Using Array.map() 🌈
One of the simplest solutions is to use the Array.map()
method in JavaScript. Here's an example of how you can leverage it in React JSX:
<tbody>
{Array(numrows).fill().map((_, index) => (
<ObjectRow key={index} />
))}
</tbody>
In the code snippet above, we first create an array of length numrows
using Array(numrows).fill()
. Then, we use map()
to iterate over the array and return a new array of JSX elements, with each element being an <ObjectRow />
component. Make sure to provide a unique key
for each repeated component to optimize rendering.
2. Using a for loop and an array 📚
If you prefer a more traditional loop approach, you can combine a for loop with an array to achieve the desired result. Here's an example:
<tbody>
{(() => {
const rows = [];
for (let i = 0; i < numrows; i++) {
rows.push(<ObjectRow key={i} />);
}
return rows;
})()}
</tbody>
In this approach, we declare an empty array, rows
, and use a for loop to push the <ObjectRow />
components into it. Finally, we return the array within an immediately invoked function expression (IIFE) to ensure it is executed during render.
Note: Remember to assign a unique key
to each repeated component to avoid any rendering issues.
Conclusion 🎉
Looping inside React JSX doesn't have to be complicated or confusing. By leveraging Array.map()
or combining a for loop with an array, you can easily achieve the desired outcome.
So go ahead, give these solutions a try and level up your React JSX game! 🚀 If you have any questions or other techniques you'd like to share, feel free to leave a comment below. Let's loop and create magic in your JSX code! 💫