Loop (for each) over an array in JavaScript
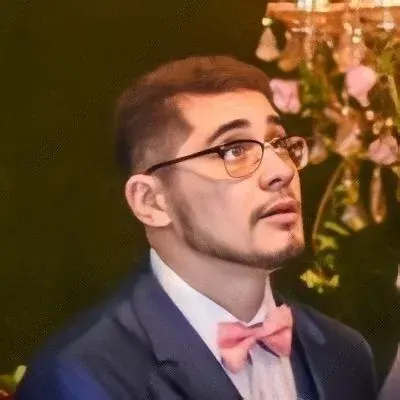
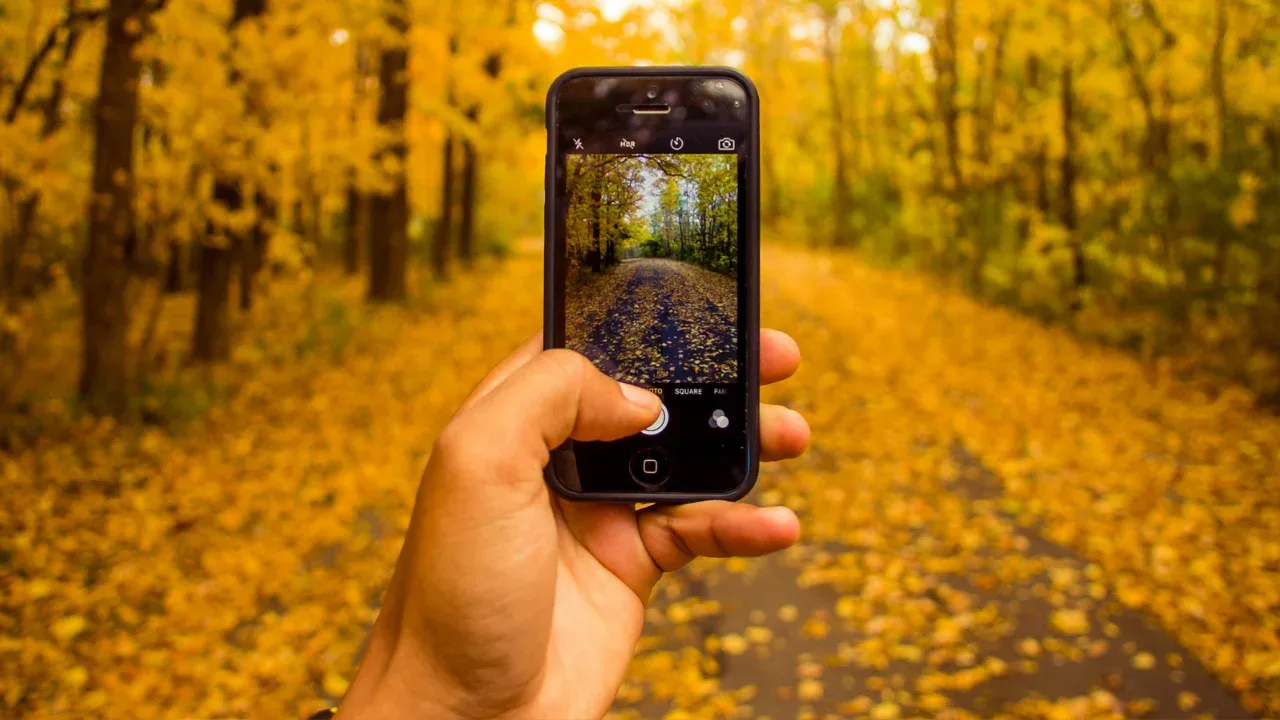
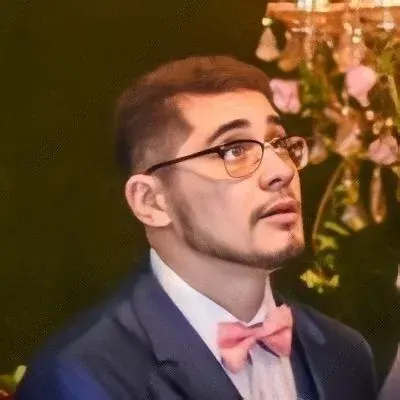
Looping Over an Array in JavaScript: A Hassle-Free Guide đŠâđģđ
Are you tired of manually iterating over every element in an array using JavaScript? đ Don't worry, we've got you covered! In this blog post, we'll explore the best and easiest ways to loop through an array using JavaScript's mighty for each loop. Let's dive right in! đ
The Common Problem đ
Frequently, developers find themselves needing to perform a task on every element within an array. Traditionally, this would involve writing multiple lines of tedious code using for loops. Fortunately, JavaScript provides a simpler alternative with its for each loop. đĒđ
The Solution: For Each Loop đ
The for each loop is a concise and elegant method to iterate over the elements of an array without the need for index tracking. It executes a specified function for each item in the array, making your code more readable and maintainable. Let's see it in action: đđ
const myArray = [1, 2, 3, 4, 5];
myArray.forEach((element) => {
// Perform your operation on each element
console.log(element);
});
In the example above, we created an array called myArray
and used the for each loop to print each element to the console. Feel free to replace console.log(element);
with any operation you need to perform on each element of the array. đ¯
Handling Common Issues đ¤¯
Breaking Loop Execution âšī¸
In some scenarios, you may want to prematurely exit the loop before it finishes iterating over the entire array. To achieve this, you can use the return keyword inside the loop's callback function:
myArray.forEach((element) => {
if (element === 3) {
// Break the loop when element equals 3
return;
}
console.log(element);
});
In the example above, the loop will terminate when it encounters an element equal to 3. You can customize this condition as per your requirements. đ
Modifying the Original Array âī¸
The for each loop is great for reading or manipulating the elements in an array but doesn't directly provide the means to modify the original array. However, you can achieve this by accessing the index parameter available within the loop:
myArray.forEach((element, index) => {
// Modify the array by doubling each element
myArray[index] = element * 2;
});
In the above snippet, we double each element in the array by directly modifying myArray
using the index parameter. Feel free to apply any modifications you desire to suit your specific use case. đĒđĄ
A Call to Action: Share Your Thoughts! đŦđ
Now that you're armed with the knowledge of looping over arrays in JavaScript using the for each loop, go ahead and refactor your code, making it more elegant and concise! Have you encountered any unique scenarios while using this loop? Share your experiences and insights in the comments below! Let's learn and grow together! đąđĄ
Happy coding! đģđ