Loading cross-domain endpoint with AJAX
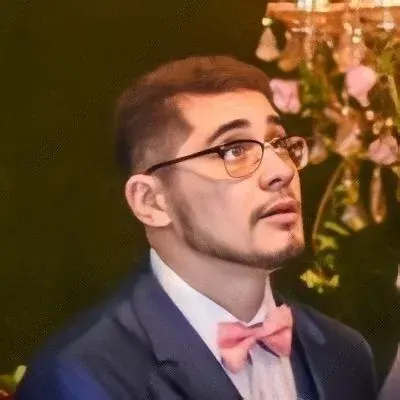
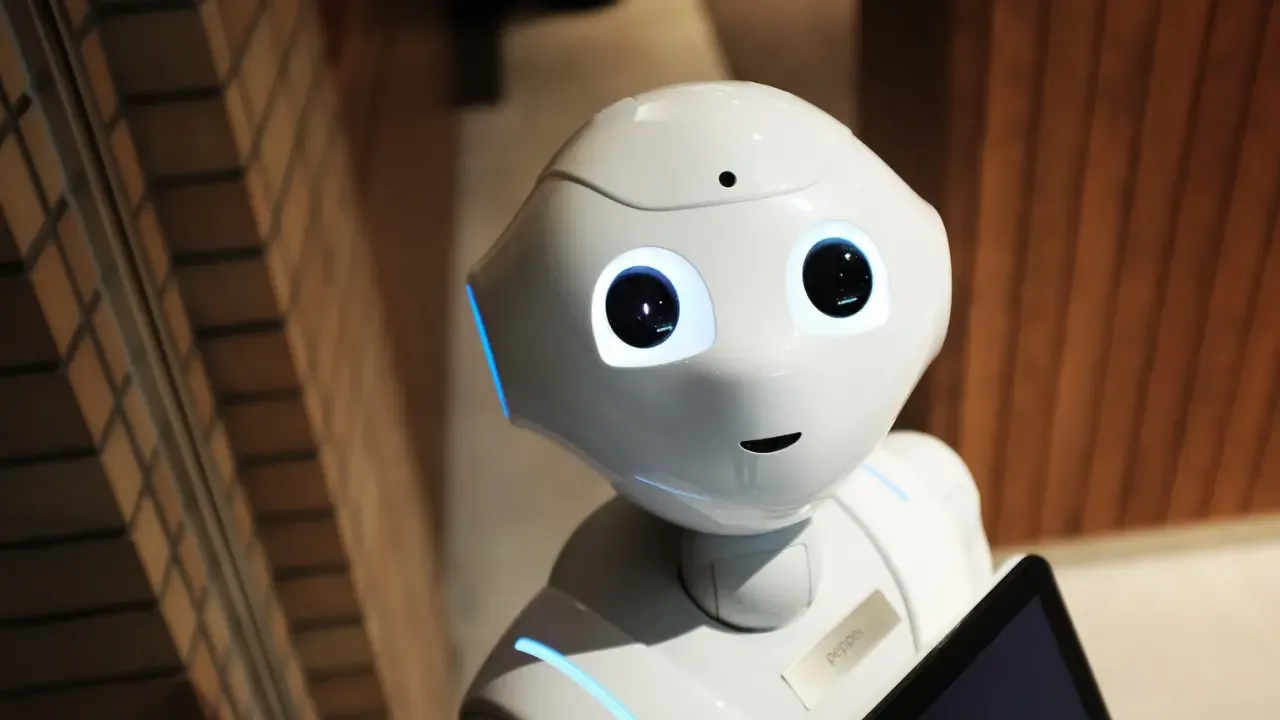
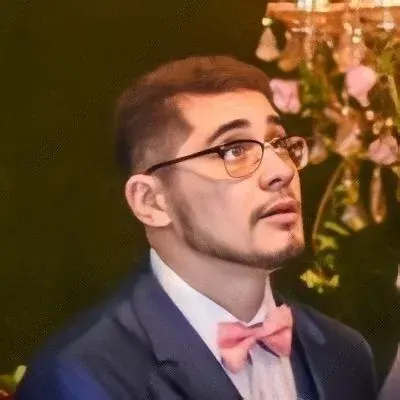
📚 Loading Cross-Domain Endpoint with AJAX: A Complete Guide
Have you ever tried loading a cross-domain HTML page using AJAX and encountered issues with receiving a response? We understand your frustration, but worry not! In this guide, we'll address common issues and provide easy solutions for loading cross-domain endpoints with AJAX. 🌐✨
The Problem: Expecting a Script MIME Type
If you've attempted to load a cross-domain HTML page using AJAX without specifying the dataType
as "jsonp," you might have encountered difficulties in obtaining a response. This is due to the browser expecting a script MIME type but receiving "text/html." 😫
To illustrate the problem, here's an example code snippet:
$.ajax({
type: "GET",
url: "http://saskatchewan.univ-ubs.fr:8080/SASStoredProcess/do?" +
"_username=DARTIES3-2012&_password=P@ssw0rd" +
"&_program=%2FUtilisateurs%2FDARTIES3-2012%2FMon+dossier%2Fanalyse_dc" +
"&annee=2012&ind=V&_action=execute",
dataType: "jsonp",
}).success(function(data) {
$('div.ajax-field').html(data);
});
The code above specifies the dataType
as "jsonp." However, this might not be the ideal solution for your particular situation. So, let's explore some alternatives! 🤔
Solution 1: Leveraging the crossDomain
Parameter
You mentioned that you've tried using the crossDomain
parameter but had no success. However, it's worth revisiting this approach as it can be a viable solution for certain scenarios.
In your AJAX request, add the crossDomain
parameter as follows:
$.ajax({
type: "GET",
url: "http://saskatchewan.univ-ubs.fr:8080/SASStoredProcess/do?" +
"_username=DARTIES3-2012&_password=P@ssw0rd" +
"&_program=%2FUtilisateurs%2FDARTIES3-2012%2FMon+dossier%2Fanalyse_dc" +
"&annee=2012&ind=V&_action=execute",
dataType: "jsonp",
crossDomain: true,
}).success(function(data) {
$('div.ajax-field').html(data);
});
By setting crossDomain: true
, you're explicitly indicating that the AJAX request is intended for a cross-domain interaction. Give this solution a try and see if it resolves your issue! 🌍🔀
Solution 2: Receiving HTML Content in JSONP
If the previous solution didn't work for you, and you still want to avoid using "jsonp" for the request, another option is to receive the HTML content in JSONP format. 📥🔀
To achieve this, you'll need to modify the server-side response to wrap the HTML content within a JSONP function callback. This allows the JSONP response to be processed as expected.
For example, if your current response is:
<html>
<body>
<h1>Hello, World!</h1>
</body>
</html>
You would need to transform it into a JSONP response like this:
callbackFunction({
"html": "<html><body><h1>Hello, World!</h1></body></html>"
});
Where callbackFunction
is a function name defined and handled on the client-side.
By modifying the server-side response to return the HTML content within a JSONP callback, you can overcome the "unexpected <" error and successfully process the response. 🎉
Engage with Us!
We hope these solutions have helped you overcome your cross-domain loading issues with AJAX. If you have any questions or other related topics you'd like us to cover, feel free to reach out to us or leave a comment below! Let's continue exploring and conquering the fascinating world of web development together! 🚀💬
Stay tuned for more informative and engaging content. Don't forget to share this blog post with your fellow developers who might also find it useful! 🤗🔗
Happy coding! 💻✨