load scripts asynchronously
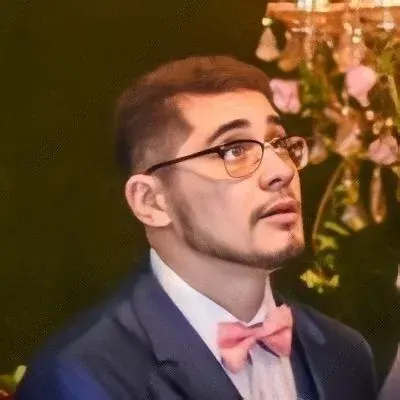
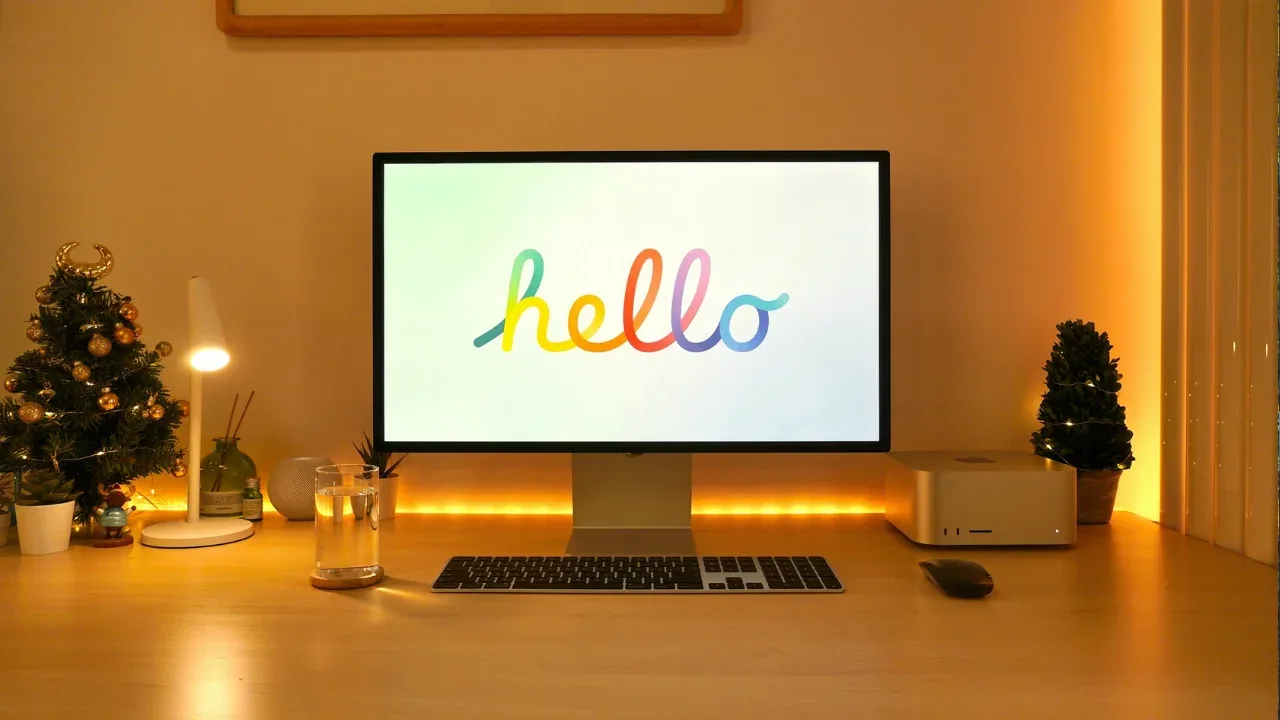
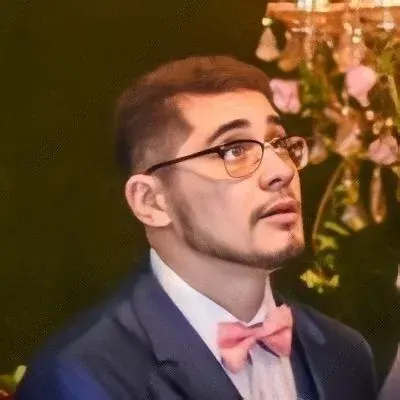
🚀 Load Scripts Asynchronously to Speed up Your Website
Are you tired of waiting for your website to load? Do you want to give your users a smooth browsing experience? Well, you've come to the right place! In this blog post, I'll show you how to load scripts asynchronously and solve common issues that you might encounter along the way. Let's get started! 🏃♂️
The Problem
You have a website with several plugins, custom widgets, and other libraries. As a result, you have multiple .js and .css files to import. Unfortunately, this slows down your website's loading time. But don't worry, we have a solution! 😎
The Solution: Asynchronous Loading
To speed up your website, we can load scripts asynchronously. This means that the scripts will be loaded in the background while other parts of your website are loading. This allows the browser to continue rendering the page without waiting for the scripts to finish loading.
Here's an example of loading a script asynchronously using JavaScript:
(function () {
var s = document.createElement('script');
s.type = 'text/javascript';
s.async = true;
s.src = 'js/jquery-ui-1.8.16.custom.min.js';
var x = document.getElementsByTagName('script')[0];
x.parentNode.insertBefore(s, x);
})();
In this example, we create a new script element, set its type to 'text/javascript', and specify that it should be loaded asynchronously. Then, we set the source (src) attribute to the URL of the script file. Finally, we insert the script element before the first script tag in the document.
Common Issues and Solutions
1. Page Not Working Properly
If your page stops working properly after loading scripts asynchronously, it might be due to dependencies between the scripts. In other words, some scripts might rely on others to function correctly.
To solve this issue, make sure to load the scripts in the correct order. You can use a timeout function to wait for one script to load before proceeding with the next one. For example:
setTimeout(insertNext1, 1000); // Wait for 1 second to load the next script
2. Links Not Clickable
If your links are not clickable after loading scripts asynchronously, it might be due to event listeners not being properly attached to the elements.
To solve this issue, ensure that you have added event listeners to the elements correctly. Double-check your code to verify that the event listeners are being added after the elements have been loaded.
3. Page Freezing
If your page is freezing after loading scripts asynchronously, it could be due to a variety of factors, such as excessive CPU usage or memory leaks.
To solve this issue, make sure your scripts are optimized and efficient. Check for any memory leaks or intensive operations that might be causing the freezing. You can also try reducing the number of scripts being loaded or optimizing your code for better performance.
A Better Alternative?
While loading scripts asynchronously can improve your website's loading time, a better alternative might be to leverage browser caching.
Here's an idea: replace your index.html page with a page that loads all the files asynchronously. Use AJAX to load the files and display a loader while they are being loaded. Once all the files are loaded, redirect users to the page you plan to use. The advantage of this approach is that subsequent visits to your website will be faster since the files will already be cached by the browser.
However, keep in mind that this approach might introduce some errors on your index page. But since the purpose of the index page is only to display the loader, these errors can be tolerated.
Final Thoughts
By loading scripts asynchronously, you can significantly improve your website's loading time and provide a better user experience. Remember to address common issues like script dependencies, event listener attachment, and page freezing.
Give it a try and let us know how it worked for you! If you have any questions or need further assistance, feel free to leave a comment below. Happy coding! ✌️
<hr>
EDIT: After looking at your code, it seems like the styles and JavaScript functions are working, but the page is frozen. To troubleshoot this issue, you can try the following steps:
Check for any JavaScript errors in your browser's console. These errors might be causing the freezing issue.
Ensure that your JavaScript functions are being called and executed correctly.
Verify that the necessary HTML elements exist on the page and are accessible by the JavaScript functions.
Consider using a JavaScript debugger to step through your code and identify any potential issues.
If the freezing issue persists, you might want to consider optimizing your code or seeking help from a developer who can assist you in identifying the root cause.
Happy coding and best of luck with your project! 💪