Listening for variable changes in JavaScript
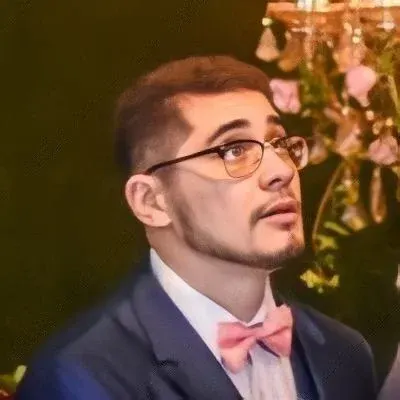
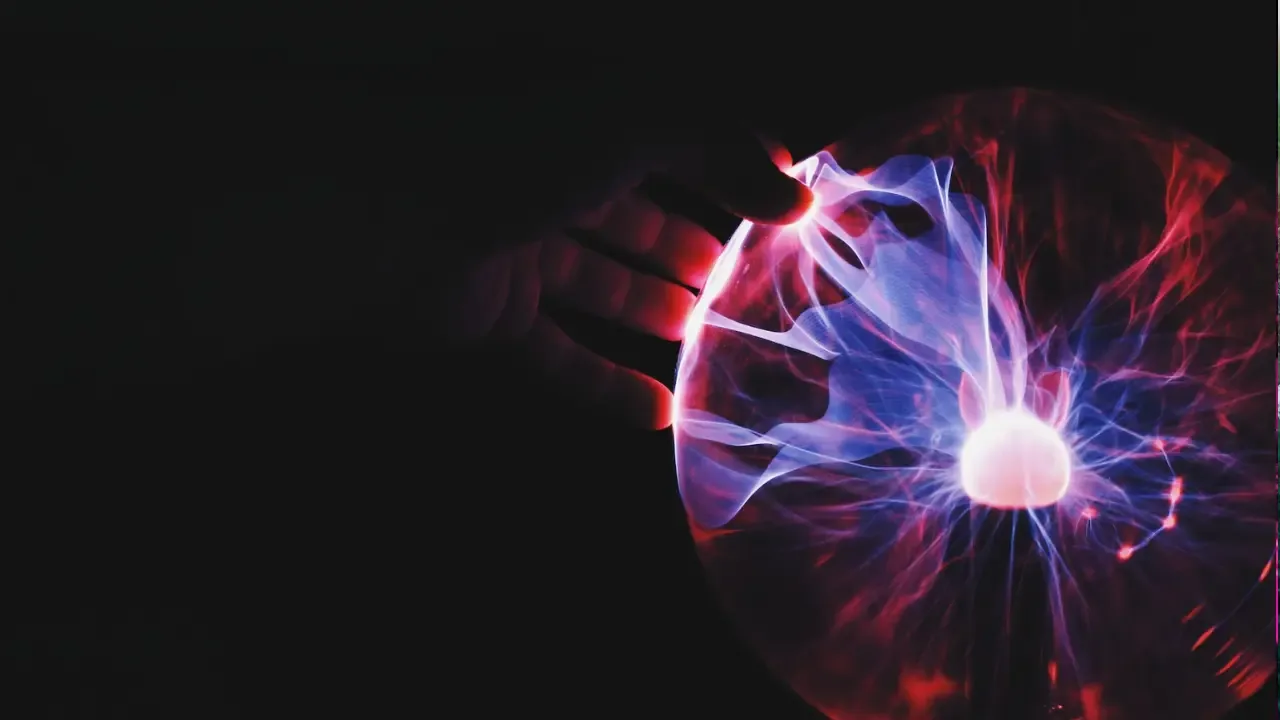
π§ Listening for Variable changes in JavaScript: A Guide for Rockstar Developers
π Hey there, Rockstar Developers! π€
So, you've got a variable and you want to know when its value changes, huh? π€ Well, you've come to the right place! In this guide, we're going to explore some common issues and easy solutions for listening to variable changes in JavaScript, with the added bonus of using the awesome power of jQuery! πͺ
The Challenge: Variable Changes on the Dance Floor πΊπ
Before we jump into the solutions, let's set the stage. Imagine you have a variable called myVariable
that you're keeping an eye on. Whenever myVariable
changes its value, you want to be notified and do some fancy stuff. Is it even possible? π€·ββοΈ
Solution 1: Polling - "Ask Thy Variable" π
One way to approach this is by constantly "asking" the variable if its value has changed. This technique is called polling. We can achieve this by using a neat little jQuery method called .data()
. Here's how you can do it:
// Set up the initial value
var previousValue = $('#myVariable').val();
// Poll the variable regularly
setInterval(function() {
var currentValue = $('#myVariable').val();
if (previousValue !== currentValue) {
// Do something if the value has changed
console.log('Variable has changed!');
// Update the previous value
previousValue = currentValue;
}
}, 500); // Check every 500 milliseconds
With this solution, we continuously compare the current value of the variable with its previous value. If they differ, we can trigger actions accordingly. However, keep in mind that polling can be a resource-intensive approach, especially if you have many variables to monitor. β±
Solution 2: Mutation Observer - "The Watchful Eye" π
For a more elegant and efficient solution, we can harness the power of Mutation Observer. This JavaScript API allows us to asynchronously observe changes to DOM elements. Let's see how we can apply this technique to our variable conundrum:
// Select the target element
const target = document.getElementById('myVariable');
// Create a new instance of Mutation Observer
const observer = new MutationObserver(function(mutationsList) {
// Check if the value property has changed
if (mutationsList[0].type === 'attributes' && mutationsList[0].attributeName === 'value') {
// Do something if the value has changed
console.log('Variable has changed!');
}
});
// Start observing the target element for value changes
observer.observe(target, { attributes: true });
// Whenever you want to change the value of the variable
$('#myVariable').val('new value');
By using Mutation Observer, we can specifically target the value
attribute of the element associated with the variable we're interested in. This approach is more efficient since it only triggers when the value truly changes, ensuring we don't waste resources. π
Your Turn to Rock and Roll! πΈ
So, Rockstar Developers, are you ready to unleash the power of listening to variable changes in JavaScript? π Try out these solutions in your code and get ready to rock the programming world! π€
If you have any other cool techniques or questions, let's connect in the comments section below! Together, we'll take our JavaScript skills to the next level! π₯π»
Keep on jamming! πΆ
PS: Don't forget to subscribe to our newsletter for more rockin' content! π©
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
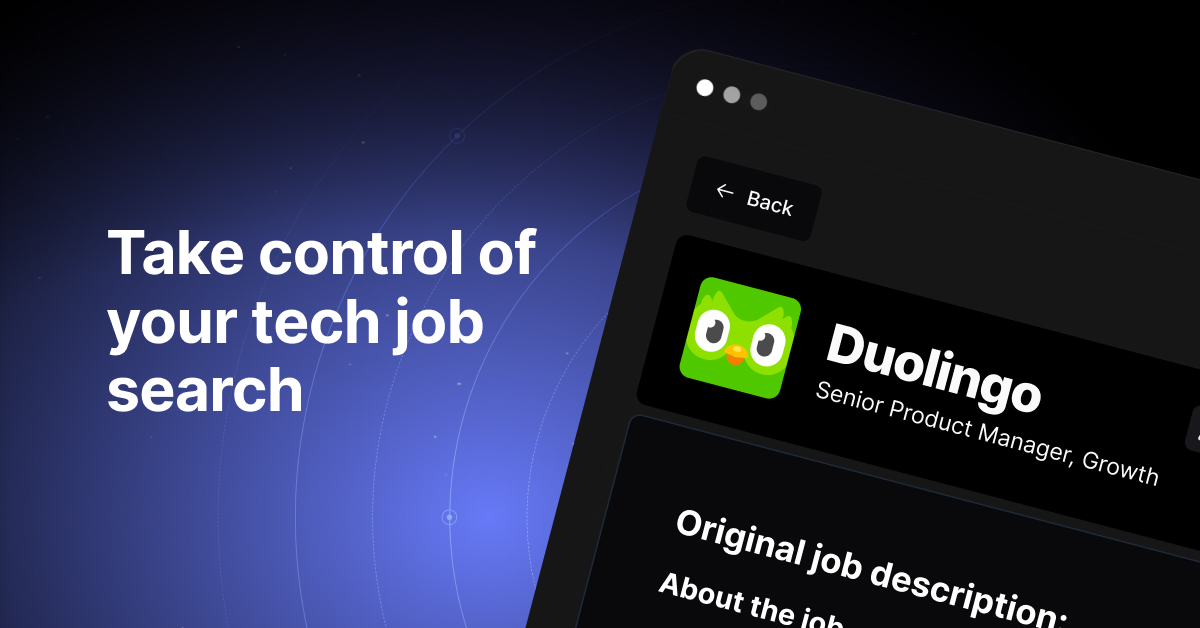