Length of a JavaScript object
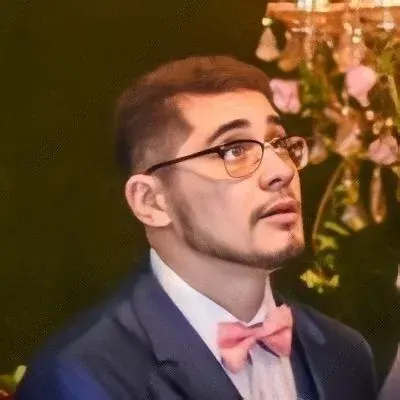
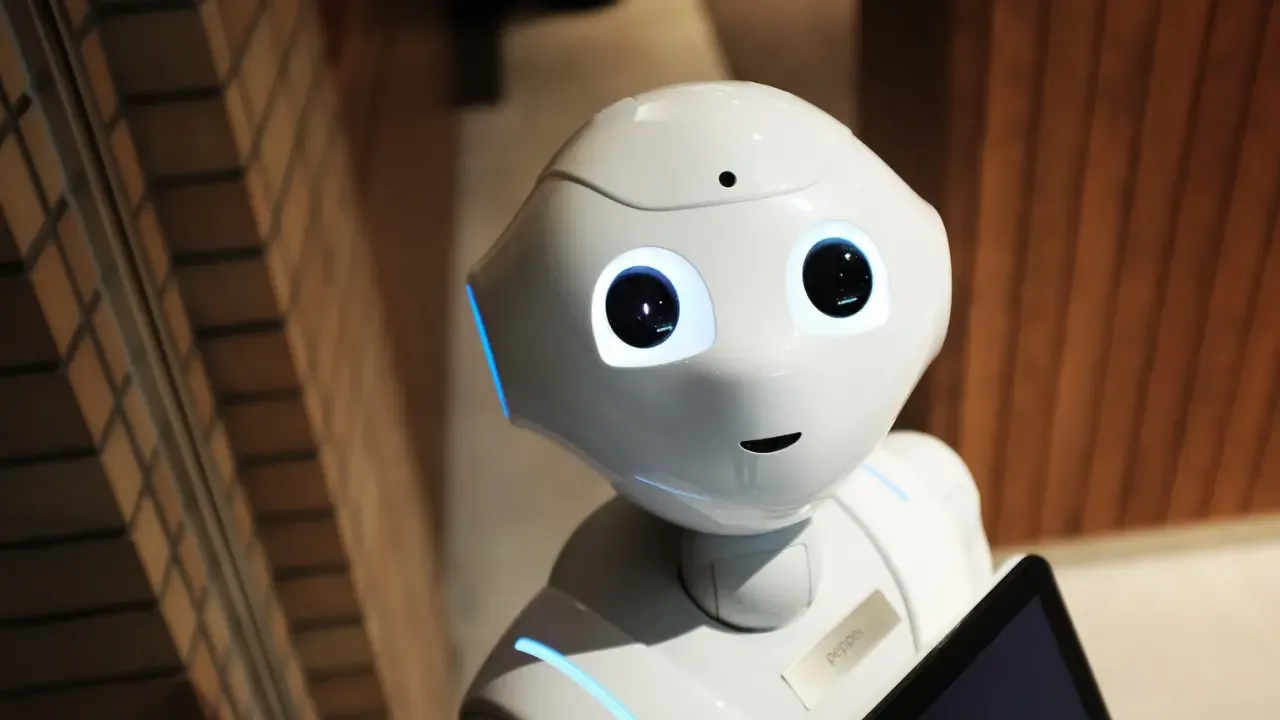
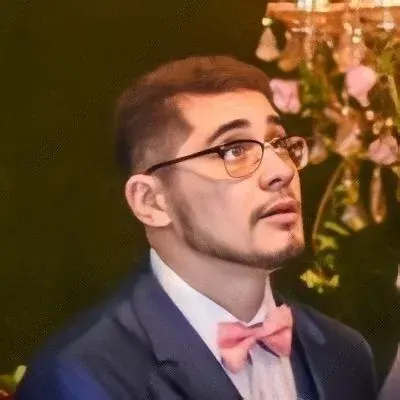
How to Get the Length of a JavaScript Object 📏
So, you have a JavaScript object and you're scratching your head wondering how to find its length. Don't worry, my friend, it's a common question. And in this blog post, I'm going to show you not just one, but two easy solutions to tackle this problem. Cool, right? Let's dive in! 💪
The Challenge: Getting the Length of a JavaScript Object
Imagine you have a JavaScript object named myObject
that looks like this:
const myObject = {
"firstname": "Gareth",
"lastname": "Simpson",
"age": 21
};
Now, the question is: How do you determine the length of this object? 🤔
Solution 1: Using Object.keys()
One popular way to measure the length of a JavaScript object is by using the Object.keys()
method. This method returns an array of all the keys in the object, and the length of this array is the length of the object.
Here's how you can do it:
const objectLength = Object.keys(myObject).length;
console.log(objectLength); // Output: 3
In this example, Object.keys(myObject)
returns an array ["firstname", "lastname", "age"]
, and calling .length
on this array gives us the desired length 3
. Bingo! 🎉
Solution 2: Iterating through the Object
Another way to find the length of a JavaScript object is by using a for...in
loop to iterate through the object and count the number of properties.
Check out this code snippet:
let objectLength = 0;
for (const key in myObject) {
objectLength++;
}
console.log(objectLength); // Output: 3
In this example, we initialize objectLength
to 0
and then use the for...in
loop to iterate through each property of myObject
. For each iteration, we increment the objectLength
variable, ultimately giving us the length of the object.
The Call-to-Action: Share Your Thoughts! 🗣️
Now that you have two simple ways to get the length of a JavaScript object, why not put them to the test? Try it out on your next coding adventure and let me know how it goes! Share your thoughts, experiences, and any other cool tips in the comment section below. I'd love to hear from you! 😊
Until next time, happy coding! 👩💻👨💻