JSON datetime between Python and JavaScript
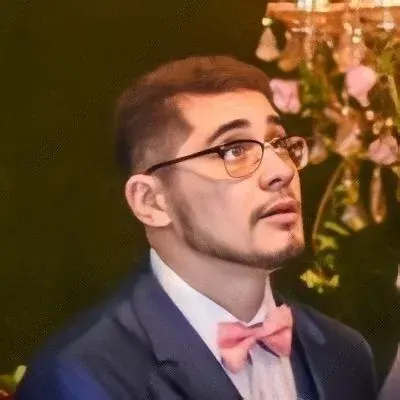
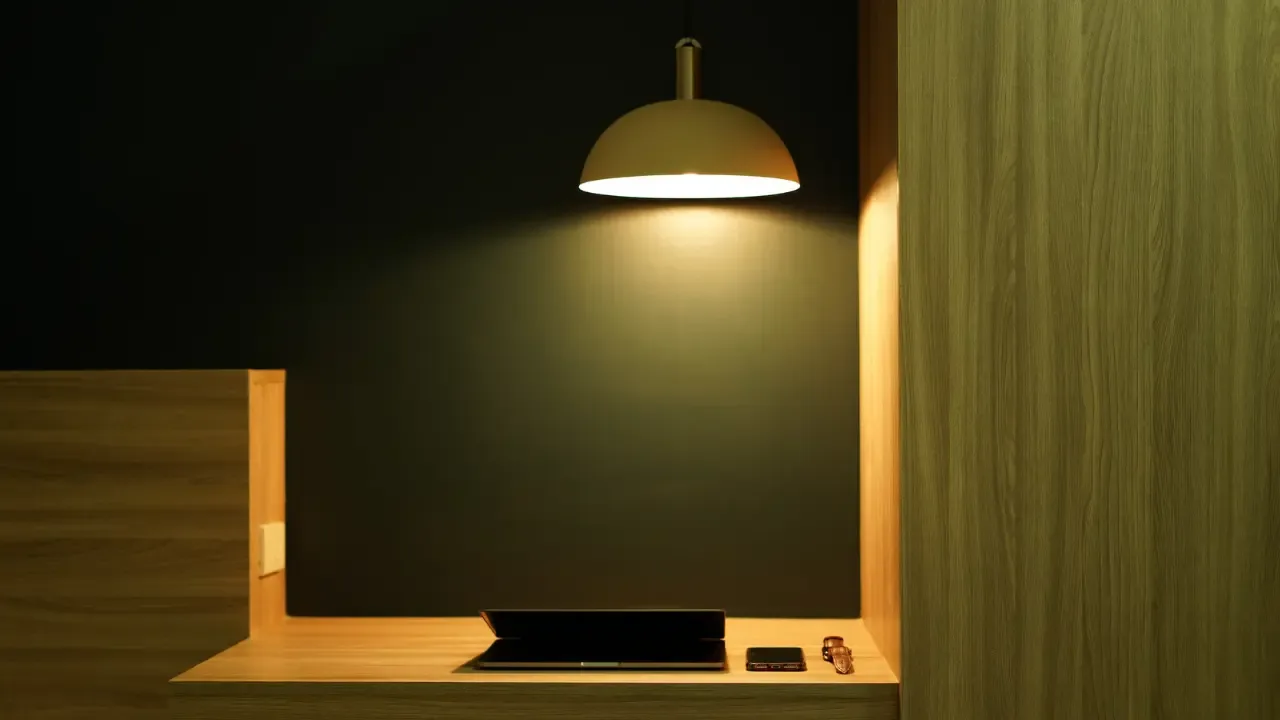
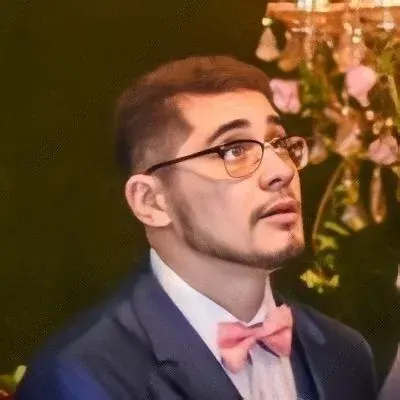
JSON datetime between Python and JavaScript: The Ultimate Guide 📅
Have you ever been stuck in a coding dilemma where you needed to send a datetime
object from Python to JavaScript using JSON? Fear not! In this guide, we will explore common issues, provide easy solutions, and ensure a smooth data transfer between these two programming languages. 🚀
The Problem: Serializing and Deserializing Datetime Objects ⏳
The question arises when we need to transmit a datetime.datetime
object, which is native to the Python programming language, to JavaScript. JSON (JavaScript Object Notation) serves as the intermediator since it is widely supported by both languages. However, transmitting datetime
objects can pose challenges due to differences in syntax and formatting.
Common Issues 💥
Issue 1: Incompatible Datetime Formats
Python and JavaScript have different default formats for representing datetime objects. Python uses the YYYY-MM-DD HH:MM:SS
format, while JavaScript uses the YYYY-MM-DDTHH:MM:SS
format. This difference can cause conflicts during the deserialization process.
Issue 2: Missing Datetime Functionality
JavaScript doesn't have a built-in datetime
object like Python does. It lacks many standard datetime
functionalities, such as formatting and computations.
Easy Solutions 🛠️
To successfully send datetime
objects from Python to JavaScript, and vice versa, let's explore two practical solutions:
Solution 1: Use Unix Timestamps
Unix timestamps provide a platform-independent solution. In Python, you can convert a datetime
object to a Unix timestamp using the timestamp()
method. In JavaScript, you can convert the Unix timestamp back to a Date
object by multiplying it with 1000 and passing it to new Date()
.
Here's a code snippet to help you understand better:
import datetime
import json
datetime_obj = datetime.datetime.now()
unix_timestamp = datetime_obj.timestamp()
json_data = json.dumps(unix_timestamp)
# Send json_data to JavaScript
In JavaScript:
const json_data = getJsonData(); // assuming you received the JSON data
const unix_timestamp = parseFloat(json_data);
const js_datetime = new Date(unix_timestamp * 1000);
console.log(js_datetime);
// Output: Fri Jan 01 2022 00:00:00 GMT+0000 (Coordinated Universal Time)
Solution 2: Format the Datetime String
If you prefer transmitting datetime
objects as strings instead of Unix timestamps, you can standardize the format on both the Python and JavaScript sides. Use the strftime()
method in Python to format the datetime
object and parse the formatted string in JavaScript using libraries like Moment.js.
Python:
import datetime
import json
datetime_obj = datetime.datetime.now()
formatted_datetime = datetime_obj.strftime("%Y-%m-%d %H:%M:%S")
json_data = json.dumps(formatted_datetime)
# Send json_data to JavaScript
In JavaScript using Moment.js:
const json_data = getJsonData(); // assuming you received the JSON data
const formatted_datetime = moment(json_data, "YYYY-MM-DD HH:mm:ss").toDate();
console.log(formatted_datetime);
// Output: Fri Jan 01 2022 00:00:00 GMT+0000 (Coordinated Universal Time)
Call-To-Action: Share Your Experience! 💬
Have you encountered issues while transmitting datetime objects between Python and JavaScript? What solutions have you tried? We would love to hear from you in the comment section below! Let's share our experiences and help fellow developers overcome this common challenge. 🙌
Remember, smooth data transfer is key in any programming project. By following these solutions, you can ensure seamless communication between Python and JavaScript, even when it comes to datetime objects! 🌟