jQuery to loop through elements with the same class
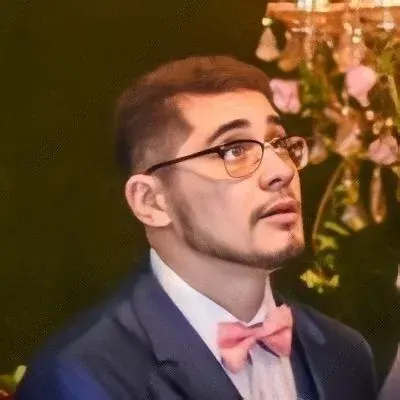
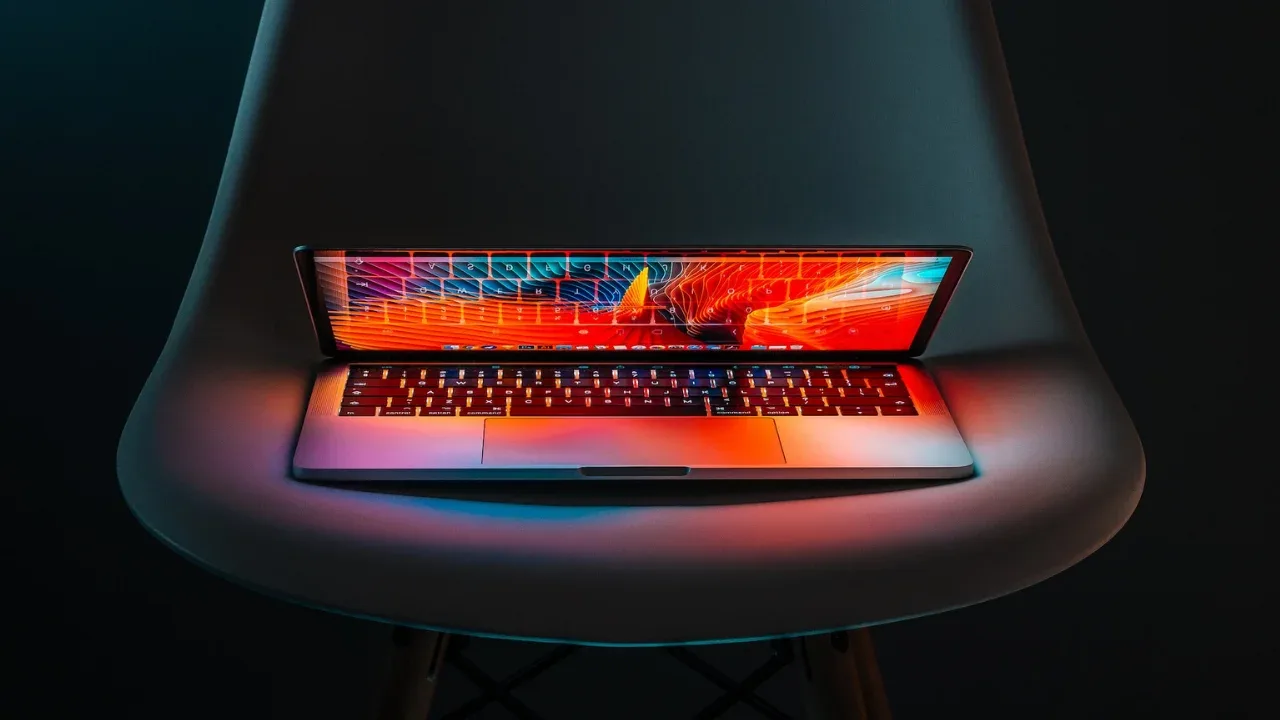
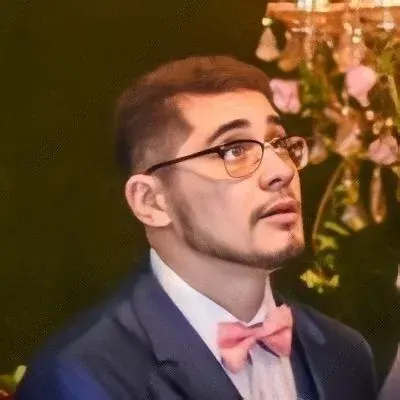
📝 Tech Blog Post: Looping Through Elements with the Same Class in jQuery 🤔💡🖥️
Introduction
Do you have a bunch of HTML elements with the same class and want to perform a specific action on each element based on certain conditions? Look no further! In this post, we'll explore an easy solution using jQuery to loop through elements with the same class. 🎉🔁💪
The Problem
Imagine you have a collection of <div>
elements with the class testimonial
and you want to check a specific condition for each of them. If the condition is true, you need to perform an action on that specific <div>
. However, figuring out the best approach can be confusing and time-consuming. 😫
The Solution
Fear not! jQuery provides a handy method called .each()
that allows us to loop through elements with a given class and perform actions on each one. Let's dive into some code examples to illustrate how this process works. 🕵️♀️🎩🔍
$('.testimonial').each(function() {
// Inside this function, "this" refers to the current element in the loop
if ($(this).find('.condition').length > 0) {
// Perform your desired action here
$(this).addClass('highlight');
}
});
In the code snippet above, we use the $
function to select all elements with the class testimonial
. The .each()
method is then called on this selection, allowing us to loop through each matching element. Inside the loop, we can access the current element using the this
keyword. We can then apply any condition using jQuery functions or methods to perform the desired action. In our example, we add a class highlight
to elements that contain a child element with the class condition
. 🙌🌟🚀
Alternate Approach
Alternatively, you can also use the for...of
loop in vanilla JavaScript to achieve the same result without relying on jQuery:
const testimonials = document.querySelectorAll('.testimonial');
for (const testimonial of testimonials) {
if (testimonial.querySelector('.condition')) {
testimonial.classList.add('highlight');
}
}
In this approach, we use querySelectorAll()
to select all matching elements and perform the same action in the loop.
Conclusion
Now you have two efficient ways to loop through elements with the same class using jQuery or vanilla JavaScript. Whether you prefer the simplicity and power of jQuery or the lightweight nature of pure JavaScript, you can easily check conditions on each element and perform actions accordingly. 🎉🔁🚀
So go ahead and give it a try in your project! If you have any questions or alternative methods, please leave a comment below. Let's loop through those elements like pros! 💪💻👍
📢 Now it's your turn! 📢
Did you find this blog post helpful? Have you tried any other approaches? Share your thoughts and experiences in the comments section below! Let's learn and grow together! 🌱💬🚀