jQuery - setting the selected value of a select control via its text description
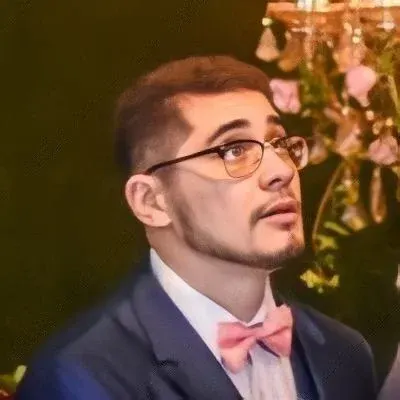
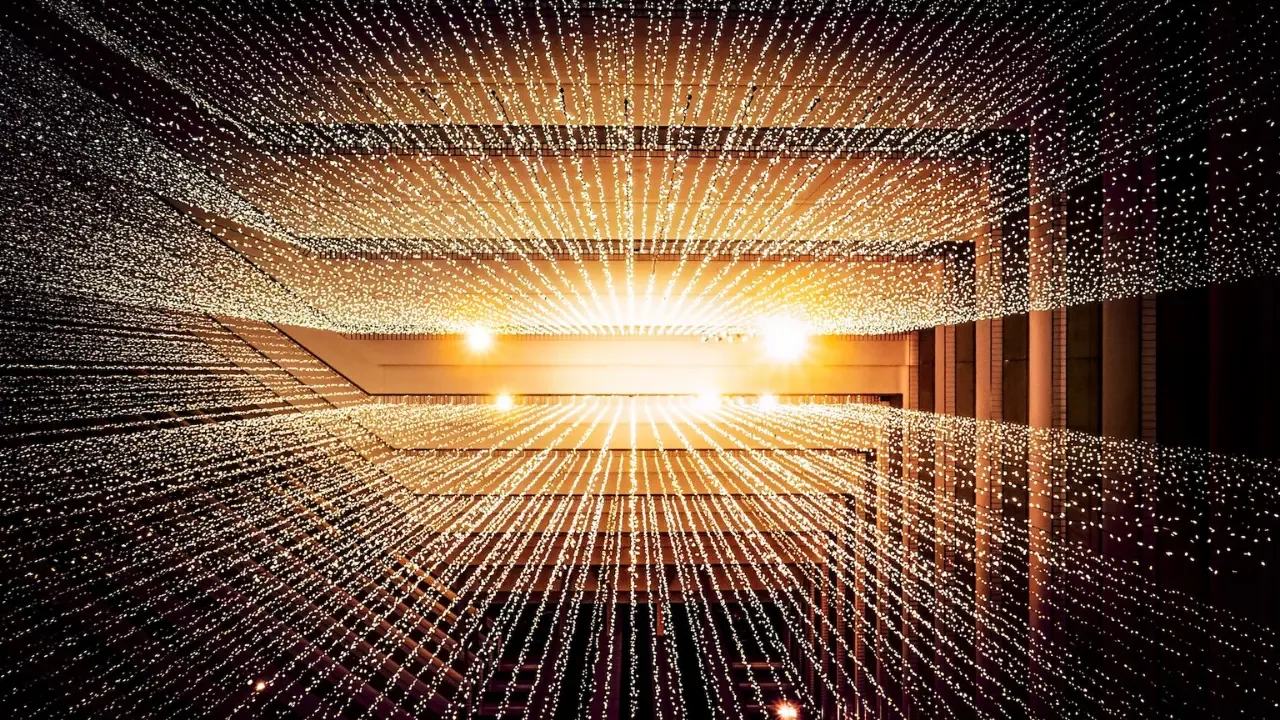
How to Set the Selected Value of a Select Control in jQuery?
š¤ Are you looking to set the selected value of a select control in jQuery based on its text description instead of its value? You've come to the right place! In this blog post, we'll address this common question and provide you with easy solutions to accomplish this task. šŖ
The Challenge
Let's first understand the scenario. You have a select control in your HTML, and you have a JavaScript variable containing a text string. Your goal is to set the selected element of the select control based on this text description, rather than using its value.
The typical method to set the selected value using the value itself is straightforward. For example:
$("#my-select").val(myVal);
But how do we achieve this when we only have the text description available? Let's dive into the solutions!
Solution 1: Using the :contains Selector
One way to solve this problem is by using the :contains selector in jQuery. This selector selects all elements that contain the specified text. Here's how you can use it to set the selected value:
// Assuming the text description is stored in a variable 'myText'
$("#my-select option:contains(" + myText + ")").prop("selected", true);
In this code snippet, we select the option elements within the #my-select select control that contain the desired text using the :contains selector. Then, we set the "selected" property to true for the matched option, which sets it as the selected value.
This solution works well, but it has a limitation. The :contains selector is case-sensitive, so it might not find a match if the case does not match exactly.
Solution 2: Using a Custom jQuery Plugin
If you want a more flexible and case-insensitive approach, you can create a custom jQuery plugin. This plugin will extend the functionalities of jQuery and allow you to set the selected value using the text description. Here's an example of how you can achieve this:
$.fn.selectByText = function (text) {
var $options = $(this).find('option');
var $selectedOption = $options.filter(function () {
return $(this).text().toLowerCase() === text.toLowerCase();
});
$selectedOption.prop("selected", true);
};
To use this plugin, simply call it on your select control and pass the desired text as an argument:
$("#my-select").selectByText(myText);
The plugin will search for the option with the matching text (case-insensitive) and set it as the selected value.
Conclusion
Now, setting the selected value of a select control in jQuery based on its text description should no longer be a challenge for you. You have two effective solutions at your disposal:
Use the :contains selector to find and set the selected value based on the text description.
Create a custom jQuery plugin for a more flexible and case-insensitive approach.
Choose the one that suits your needs and remember to give your website visitors a seamless user experience.
š Now that you know how to solve this problem, go ahead and implement it on your website! If you have any other questions or need further assistance, feel free to leave a comment below. Happy coding!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
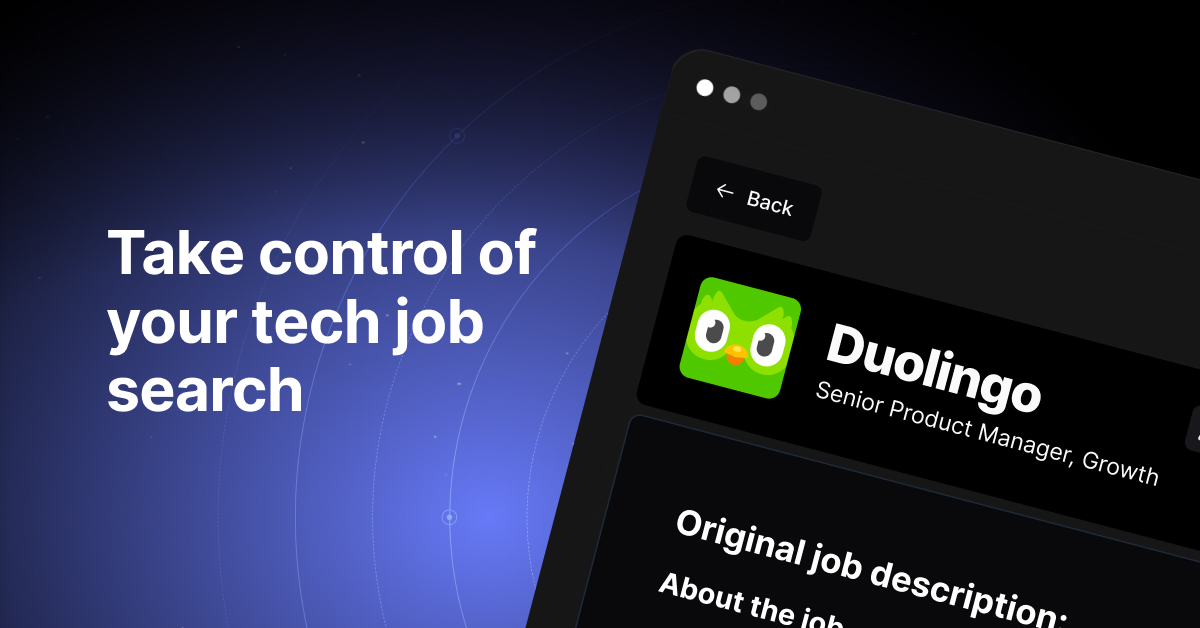