jQuery: Return data after ajax call success
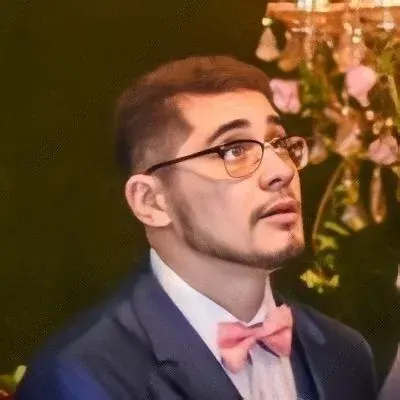
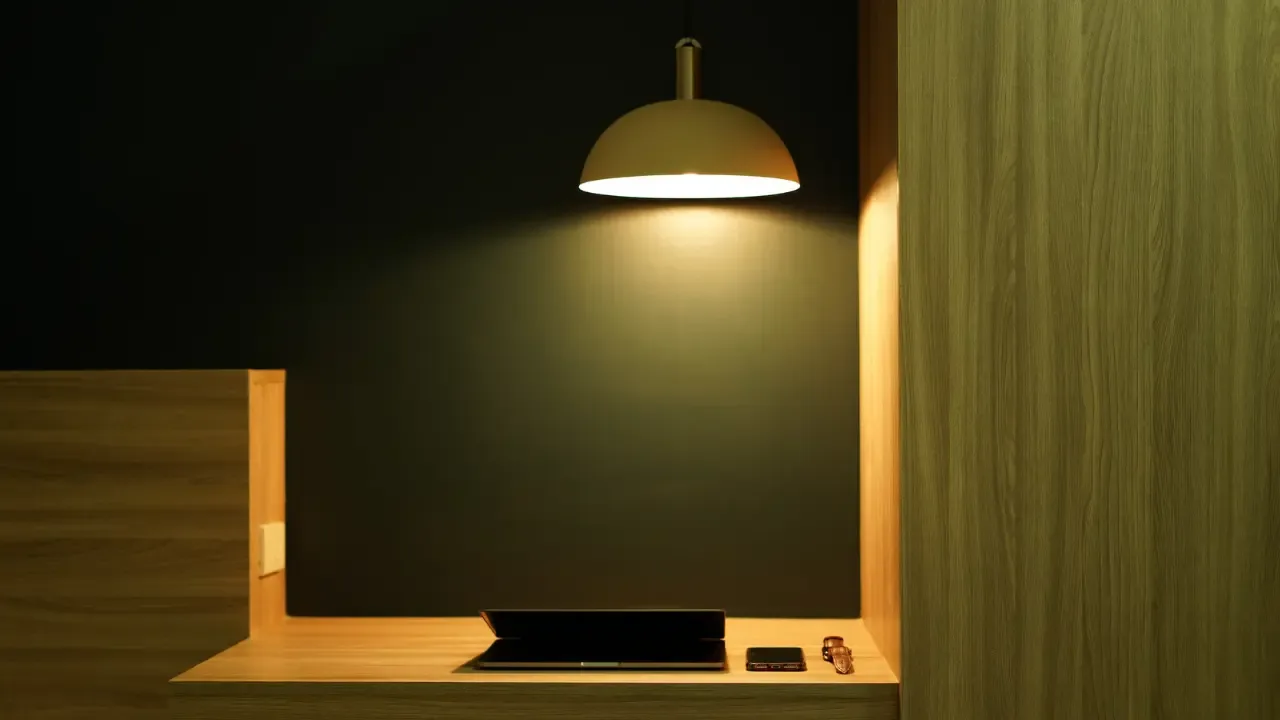
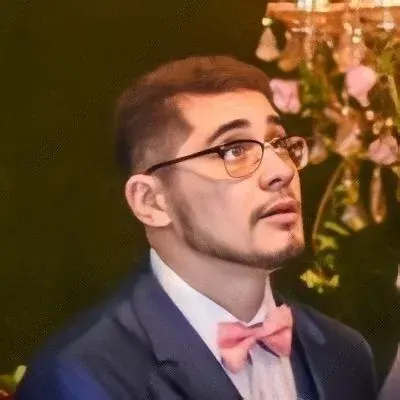
jQuery: Return data after ajax call success
Hello there tech enthusiasts! Have you ever encountered the problem of trying to return data after a successful AJAX call in jQuery? 🤔 Fear not, for today I'll guide you through this common issue and provide you with easy solutions. By the end of this post, you'll be able to handle this situation like a pro! 💪
The Problem
Let's take a look at the code snippet provided by our fellow developer:
function testAjax() {
$.ajax({
url: "getvalue.php",
success: function(data) {
return data;
}
});
}
var output = testAjax();
console.log(output); // undefined...
As you can see, the testAjax()
function makes an AJAX call to "getvalue.php"
and attempts to return the received data
. However, when assigning the result of testAjax()
to output
, it turns out to be undefined
.
The Explanation
The reason for this unexpected behavior is that the AJAX call operates asynchronously. This means that while the request is being made, the code execution continues without waiting for a response. Consequently, the return data;
statement inside the success
callback is executing after the testAjax()
function has already returned.
The Solution
To overcome this issue, we need to make use of JavaScript's Promises, which allow us to handle asynchronous operations in a more controlled manner.
function testAjax() {
return new Promise(function(resolve, reject) {
$.ajax({
url: "getvalue.php",
success: function(data) {
resolve(data);
},
error: function(error) {
reject(error);
}
});
});
}
testAjax()
.then(function(data) {
console.log(data); // the received data
})
.catch(function(error) {
console.log("An error occurred: " + error);
});
In the updated code, we return a Promise object that encapsulates the asynchronous AJAX call. The resolve
method is used inside the success
callback to fulfill the promise, passing the received data as the value. Conversely, the reject
method is used inside the error
callback to reject the promise if an error occurs.
By chaining the then
and catch
methods to the testAjax()
call, we can handle the retrieved data or any encountered errors respectively.
Conclusion
Now you're equipped with the knowledge to deal with returning data after a successful AJAX call in jQuery. Remember to embrace the power of Promises to control the asynchronous flow of your code.
Feel free to share your own experiences or ask any questions in the comments below! Let's dive into a discussion and enhance our tech know-how together! 🚀