JQuery - $ is not defined
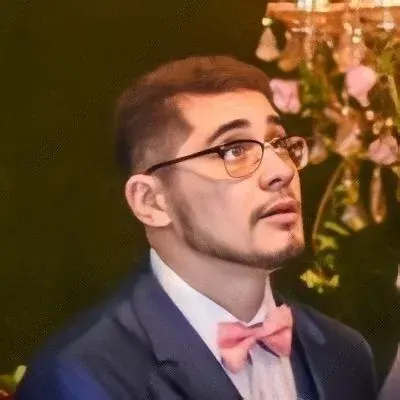
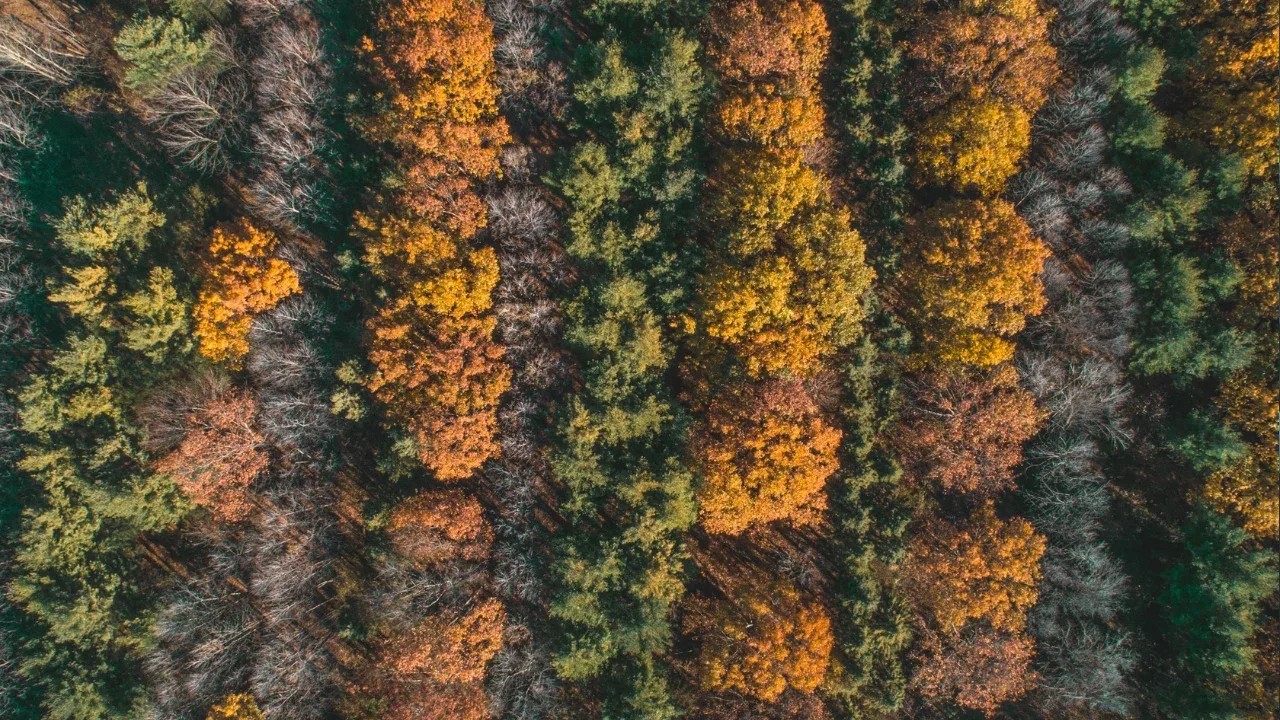
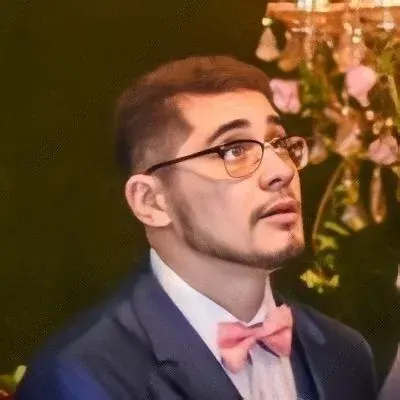
💡 Understanding the "$ is not defined" Error in jQuery
Have you ever encountered the "$ is not defined" error while working with jQuery? Don't worry, you're not alone! This common issue often arises when jQuery is being used incorrectly or when there are problems with its reference. In this blog post, we will dive into the causes of this error and provide easy solutions to help you get your jQuery code up and running smoothly.
📝 The Problem: $ is not defined
The error message "$ is not defined" typically occurs when jQuery is not properly loaded or referenced in your code. Let's take a closer look at the code provided in the context:
<p>I have a simple jQuery click event</p>
<pre><code><script type="text/javascript">
$(function() {
$('#post').click(function() {
alert("test");
});
});
</script>
</code></pre>
<p>and a jQuery reference defined in the site.master</p>
<pre><code><script src="<%=ResolveUrl("~/Scripts/jquery-1.3.2.js")%>" type="text/javascript"></script>
</code></pre>
In the provided code, the issue lies in the missing reference or improper loading of the jQuery library.
🛠️ The Solution: Properly Load and Reference jQuery
To resolve the "$ is not defined" error, follow these simple steps:
1. Check the jQuery Reference
First, ensure that the jQuery library is correctly referenced in your HTML file. Double-check the path and make sure it is being loaded before your code that uses jQuery. In our example, the script tag in the site.master file references the jQuery library correctly.
2. Confirm jQuery Is Loaded
Next, verify that the jQuery library is indeed being loaded on your webpage. You can do this by checking the Network tab in your browser's Developer Tools (e.g., Firebug).
3. Use $(document).ready()
Instead of using $(function() {})
to encapsulate your code, try using $(document).ready(function() {})
. This ensures that your code only executes once the DOM is fully loaded, which can prevent issues with accessing jQuery. Update the code as follows:
<script type="text/javascript">
$(document).ready(function() {
$('#post').click(function() {
alert("test");
});
});
</script>
4. Update jQuery Version
Consider updating the jQuery version you are using. Outdated versions may have compatibility issues. As a best practice, it is recommended to use the latest stable version of jQuery.
5. Check for Conflicts
Ensure that there are no conflicts with other JavaScript libraries on your webpage. Conflicts can occur if you are using multiple versions of jQuery or if another library is overwriting the '$' symbol. jQuery provides a noConflict()
method to handle such situations.
<script type="text/javascript">
$.noConflict();
jQuery(document).ready(function($) {
// Your jQuery code here
});
</script>
📣 Take Action: Share Your Thoughts
We hope this guide helped you understand and resolve the "$ is not defined" error in jQuery. Give these solutions a try and let us know in the comments if they worked for you! If you have any other questions or want to share additional tips, feel free to join the conversation. Happy coding! 💻🚀