jQuery get textarea text
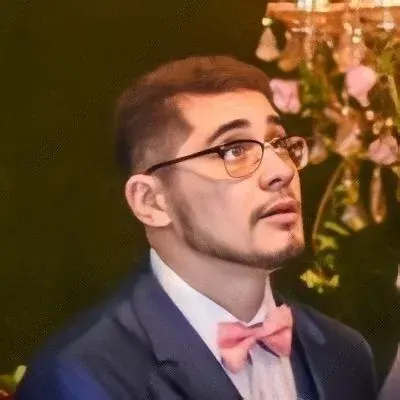
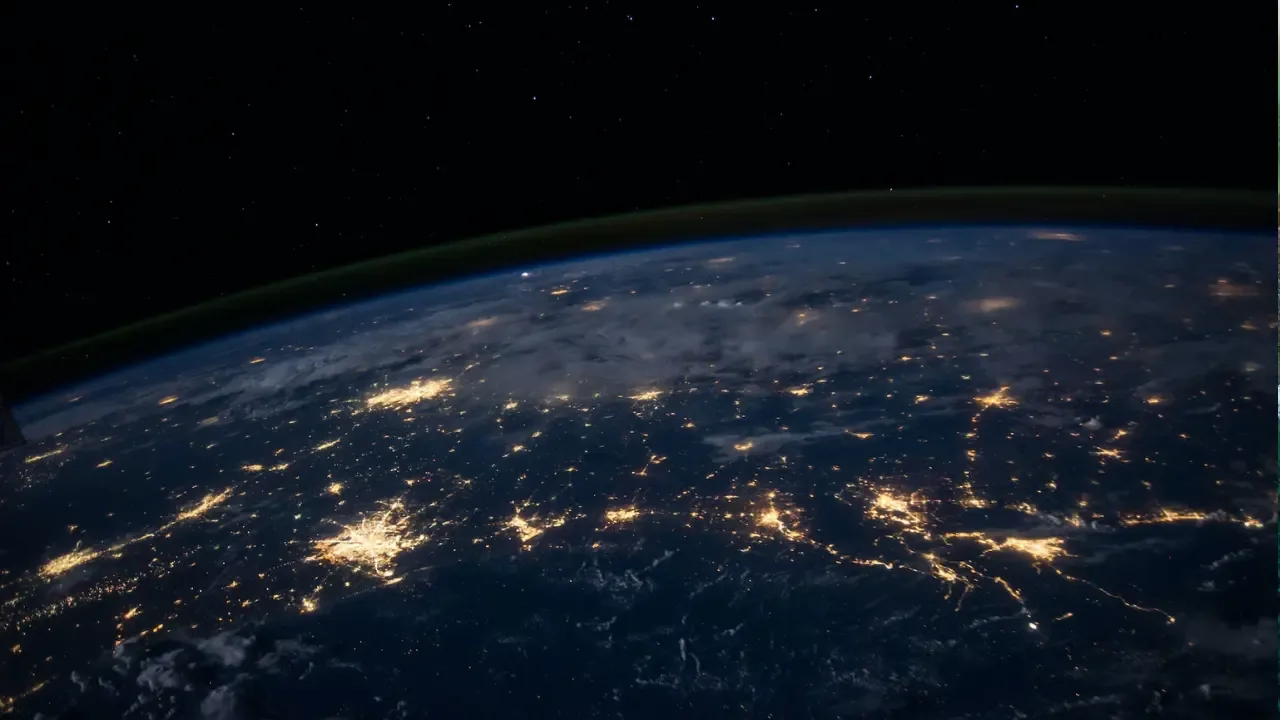
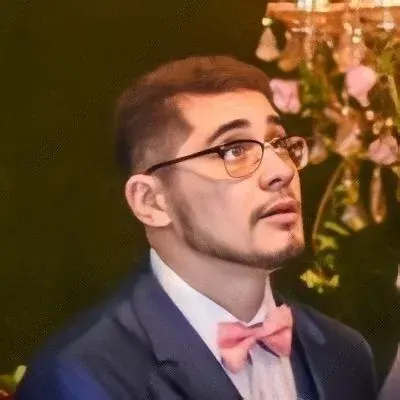
How to Get the Text from a Textarea Using jQuery 😄
So you've been dabbling with jQuery and you want to create a cool functionality on your webpage - a console-like feature where you can enter text and send it back to the server. But you're stuck on how to get the text from a textarea. Don't worry, I've got you covered! In this blog post, I'll show you how to accomplish this with easy solutions and provide answers to some common questions you may have. Let's dive in! 🏊♀️
Problem: Getting the Text from a Textarea
The original question posed by our visitor was how to retrieve the text from a textarea using jQuery. The visitor wanted to either split the text or convert key events to ASCII characters and send them to the server. But they couldn't find any information on how to achieve this. 😢
Solution #1: Using the val() Function
Luckily, jQuery makes it super easy to grab the text from a textarea. All you need is the val()
function. Here's an example:
var text = $('textarea').val();
In this example, we're selecting the textarea element using the jQuery selector $()
, and then calling the val()
function to get the text inside the textarea. The retrieved text is then stored in the text
variable. 📝
Solution #2: Using the text() Function
Alternatively, you can use the text()
function if you prefer. However, note that this function retrieves the inner text of an element, so it will not preserve line breaks or formatting within the textarea.
var text = $('textarea').text();
Just like in the previous example, we're selecting the textarea element and calling the text()
function to retrieve the text. The result is stored in the text
variable. 📋
Solution #3: Handling Key Events
If you want to capture key events and perform specific actions, such as converting keycodes to ASCII characters, you can listen for the keyup
event on the textarea. Here's an example:
$('textarea').keyup(function(event) {
var keyCode = event.keyCode || event.which;
var char = String.fromCharCode(keyCode);
// Perform desired actions with the character
});
In this example, we're attaching a keyup
event handler to the textarea element. The event
parameter gives us access to the keycode of the pressed key. We use the fromCharCode()
method to convert the keycode to its corresponding ASCII character. From there, you can perform any desired actions with the character. 🎮
Encouraging Reader Engagement: Share Your Experience!
I hope this guide has helped you in retrieving the text from a textarea using jQuery. Now it's your turn to take action! Have you ever faced a similar challenge in your web development journey? What other jQuery tricks or issues do you want to learn more about? Share your thoughts and experiences in the comments section below. Let's keep the conversation going! 💬
Remember, as a tech community, we grow and thrive by sharing knowledge and helping each other out. So don't hesitate to ask questions, provide insights, or offer alternative solutions. Together, we can make our web development adventures even more exciting! 🌟
Now go ahead and show off your newfound skills by implementing the textarea text retrieval in your project. Happy coding! 💻🚀