jQuery Event Keypress: Which key was pressed?
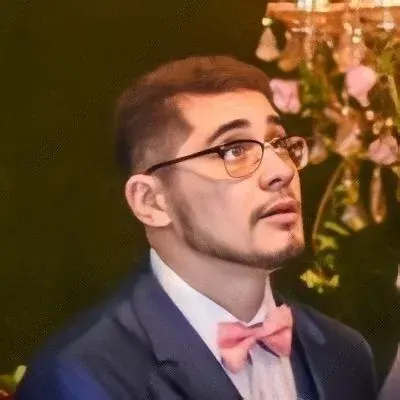
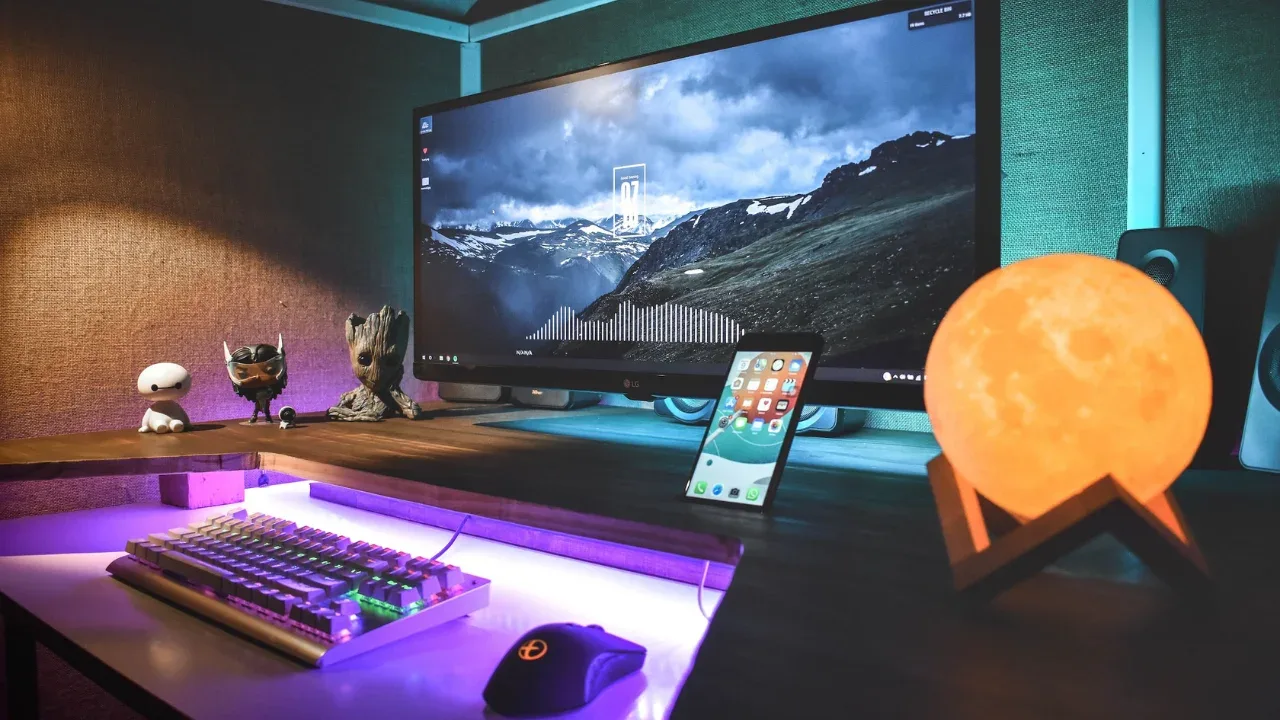
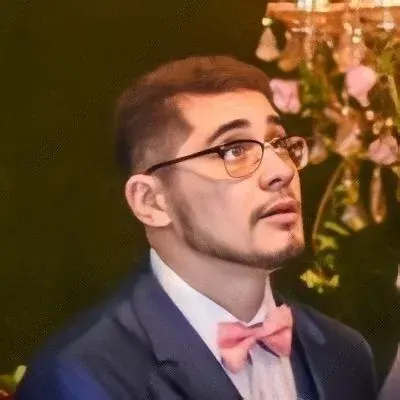
jQuery Event Keypress: Which key was pressed?
So you want to know which key was pressed when binding to the keypress event using jQuery? Well, you've come to the right place! In this blog post, we'll address common issues and provide easy solutions to this question.
The Problem
Let's start with the problem at hand. You have a search box and you want to trigger a submit event when the user presses the "ENTER" key. Here's the code snippet you have:
$('#searchbox input').bind('keypress', function(e) {});
Now, how do you find out which key was pressed inside the event handler function? Let's dive in!
The Solution
To determine which key was pressed, you can utilize the keyCode
or which
property of the event object. The keyCode
property is widely supported by most browsers, while the which
property is an older property that exists for backward compatibility.
Here's how you can use the keyCode
property to check if the "ENTER" key was pressed:
$('#searchbox input').bind('keypress', function(e) {
if (e.keyCode === 13) {
// "ENTER" key was pressed
// Perform your submit action here
}
});
Alternatively, you can use the which
property like this:
$('#searchbox input').bind('keypress', function(e) {
if (e.which === 13) {
// "ENTER" key was pressed
// Perform your submit action here
}
});
Understanding the Difference
You might be wondering if there's a difference between the keyCode
and which
properties, especially when you're specifically looking for the "ENTER" key. The truth is, for the "ENTER" key, it will never be a unicode key.
Both properties should provide the same result for the "ENTER" key across most modern browsers. However, it's worth noting that the keyCode
property is officially standardized in the W3C DOM Level 3 Events Specification, whereas the which
property is not.
Reader Engagement: Share Your Insights!
We hope this guide has helped you understand how to determine which key was pressed using the keypress event in jQuery. Now, we want to hear from you!
Have you encountered any browser compatibility issues when using the keyCode
or which
property? Do you prefer one over the other? Share your insights and experiences in the comments below. Let's learn from each other and find the best solutions together!
Remember, sharing is caring! If you found this blog post helpful, don't forget to share it with your fellow developers. Happy coding! 🚀🔥