JQuery .each() backwards
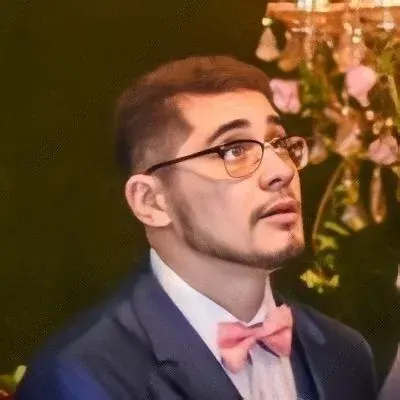
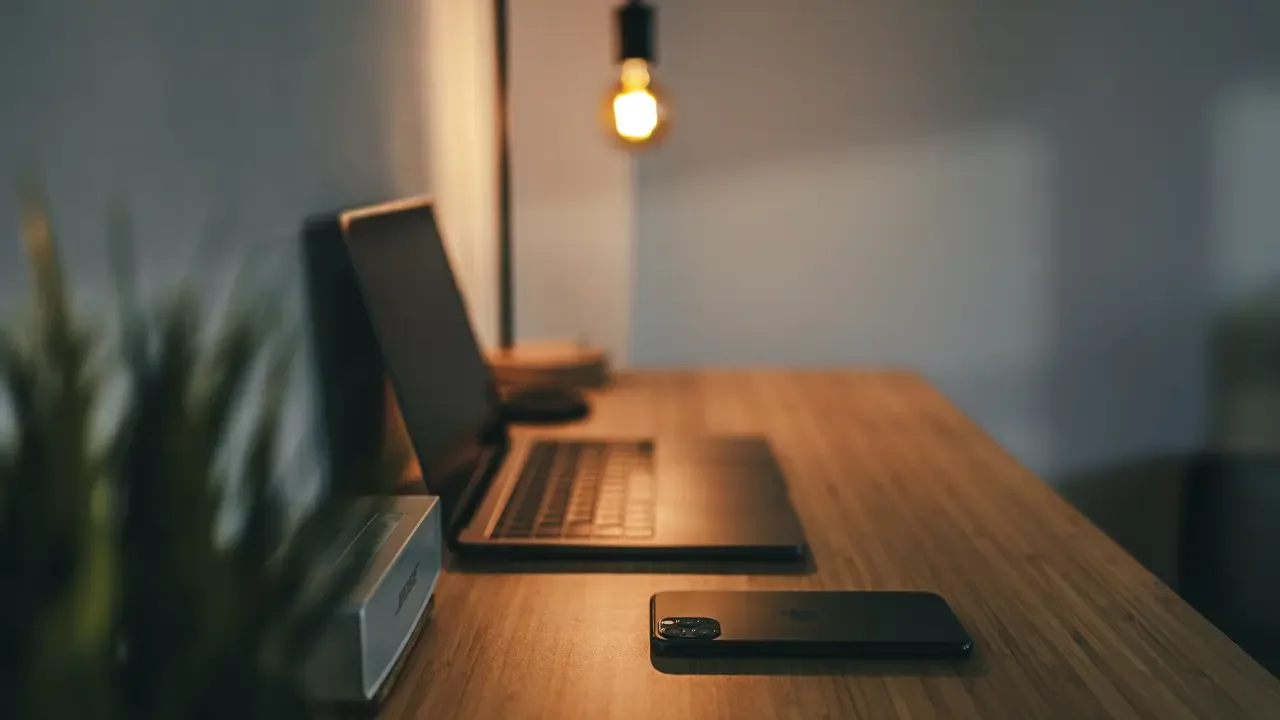
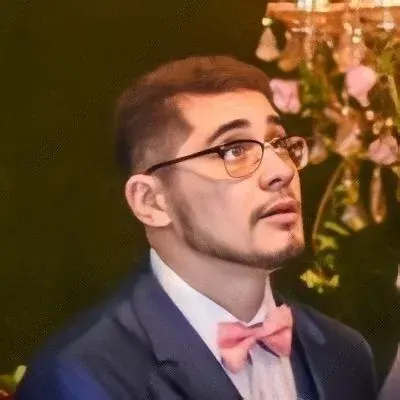
š jQuery .each() Backwards: A Simple Solution
š¤ Are you struggling with selecting elements in reverse order using the jQuery .each()
method? Don't worry, you're not alone! Many developers face this problem when trying to manipulate elements in the DOM. But fear not, because in this blog post, we will explore a simple and elegant solution to this common issue.
š„ Let's dive right in!
ā” Understanding the Problem
The jQuery .each()
method is designed to iterate over a collection of elements in the order they appear in the DOM. However, there are scenarios where you might want to process these elements in reverse order. Take the example below:
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
<li>Item 4</li>
<li>Item 5</li>
</ul>
If you want to select all the <li>
items and apply the .each()
method on them, but starting from "Item 5" and moving backwards, how can you achieve this?
š The Solution: Reverse the Selection
Fortunately, there is a straightforward solution to this problem. Instead of selecting the elements directly, we can reverse the order of the selection itself. Let's have a look at the code:
$("ul li").toArray().reverse().forEach(function(element) {
// Your code goes here
});
š Explanation:
$("ul li")
selects all the<li>
elements within the<ul>
element..toArray()
converts the jQuery selection to an array, making it easier to manipulate..reverse()
reverses the order of the array..forEach()
iterates over each element in the reversed array.
š” Example Usage:
To demonstrate the practical implementation of this solution, let's imagine we want to change the text color of each list item to red:
$("ul li").toArray().reverse().forEach(function(element) {
$(element).css('color', 'red');
});
By using the reversed selection, we can now apply the desired changes in reverse order, starting from "Item 5" and moving backwards. š
š£ Call-to-Action: Share Your Experience!
Now that you have discovered this simple solution to the jQuery .each()
backwards problem, we encourage you to give it a try in your own projects. Share your experience with us in the comments below! Is there another approach you prefer? Let us know! We love hearing from fellow developers. š
So why wait? Start tackling this issue with confidence and enjoy the benefits of reverse element iteration using jQuery's .each()
method!
Happy coding! š»š