jQuery disable/enable submit button
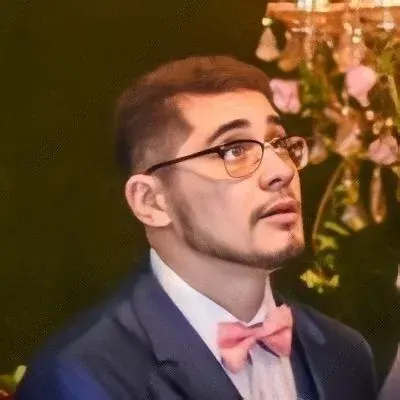
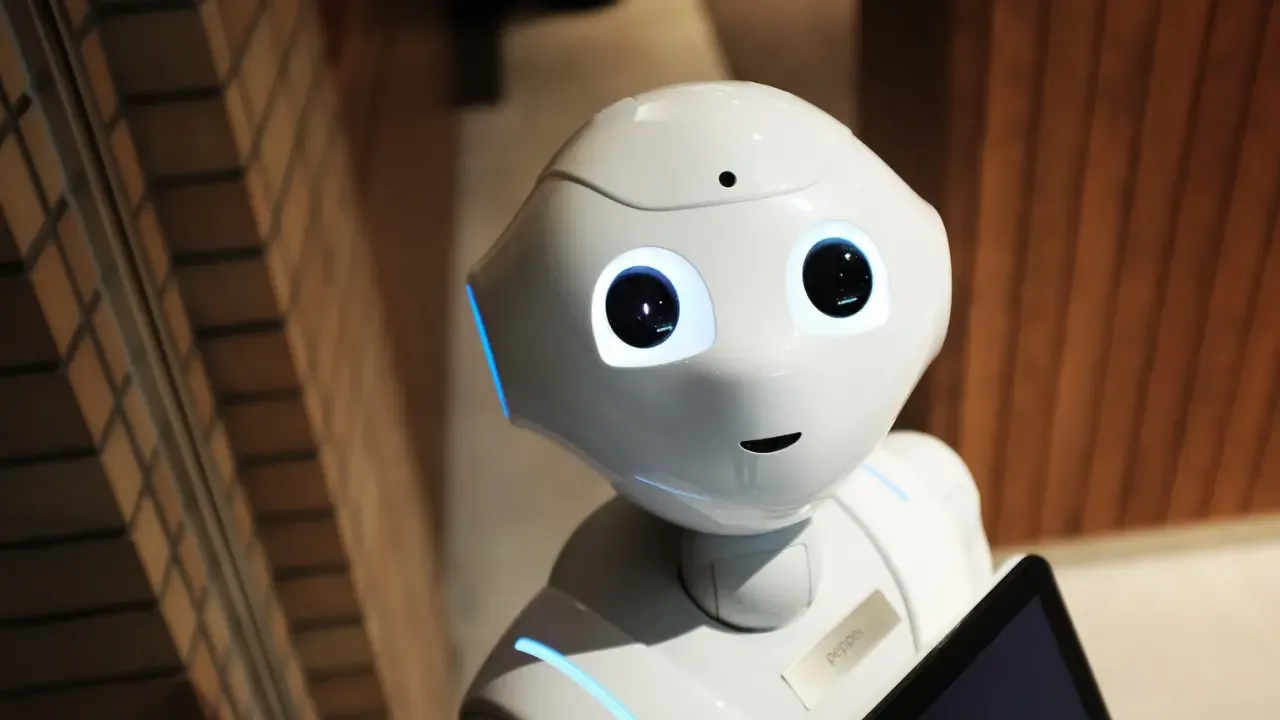
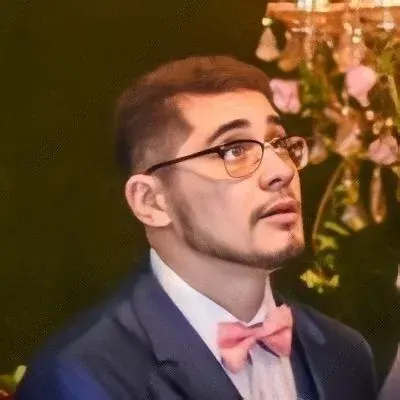
💡 jQuery Disable/Enable Submit Button: A Guide
Are you struggling with disabling/enabling a submit button in jQuery? 🤔 You're not alone! Many developers face this challenge, but worry not! We're here to provide you with easy solutions to this common issue. Let's dive right in! 💪
The Problem
The scenario involves a text field and a submit button in your HTML code. The goal is to disable the submit button when the text field is empty and enable it when something is typed. Additionally, if the text field becomes empty again, the submit button should be disabled once more. Seems straightforward enough, right? 😉
The Attempted Solution
Here's the code snippet you tried:
$(document).ready(function(){
$('input[type="submit"]').attr('disabled','disabled');
$('input[type="text"]').change(function(){
if($(this).val != ''){
$('input[type="submit"]').removeAttr('disabled');
}
});
});
However, you noticed this solution isn't working as expected. Let's understand why and find a better way to achieve the desired result. 🤔
Analyzing the Code
The issue lies in the comparison operation within the if
condition. The val()
function is missing parentheses, resulting in the incorrect comparison. The code $(this).val != ''
should be modified to $(this).val() != ''
. This change fixes the issue with your current code. 😄
Easy Solution
Alternatively, you can achieve the same result without using the change()
event. We can simplify the code by utilizing the input
event along with a concise jQuery chaining. Voila! 🎉
$(document).ready(function(){
$('input[type="submit"]').attr('disabled','disabled');
$('input[type="text"]').on('input', function(){
var submitBtn = $('input[type="submit"]');
if($(this).val() != ''){
submitBtn.removeAttr('disabled');
} else {
submitBtn.attr('disabled', 'disabled');
}
});
});
Now, whenever a key is pressed or deleted in the text field, the input
event will trigger, and the code will check if the text field is empty or not. It will enable or disable the submit button accordingly. 🙌
Let's Recap
To recap, the incorrect comparison operation was causing the issue in your original code. By using the val()
function with parentheses, you can retrieve the value of the text field correctly. Additionally, by using the input
event, you can track changes in real-time more efficiently. 🔄
Time to Rock and Roll!
Now, it's your turn to implement these changes and witness the magic happen! Feel free to customize the code according to your specific needs. We hope this guide provided you with a clear and easy solution to disabling/enabling a submit button using jQuery. 🚀
Have any questions or facing any issues? Feel free to let us know in the comments below. We're here to help! 😊
Let's Connect!
Don't forget to follow us on social media for more exciting tech tips and tricks! Join our vibrant community and stay up-to-date with the latest trends in the tech industry. 💻✨
Instagram: Follow us Facebook: Like us Twitter: Follow us
Keep coding, keep exploring! Happy jQuerying! 💻🌟