jQuery checkbox change and click event
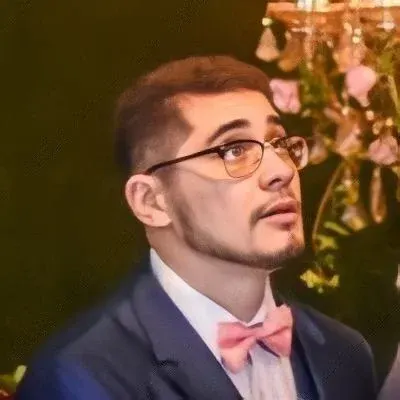
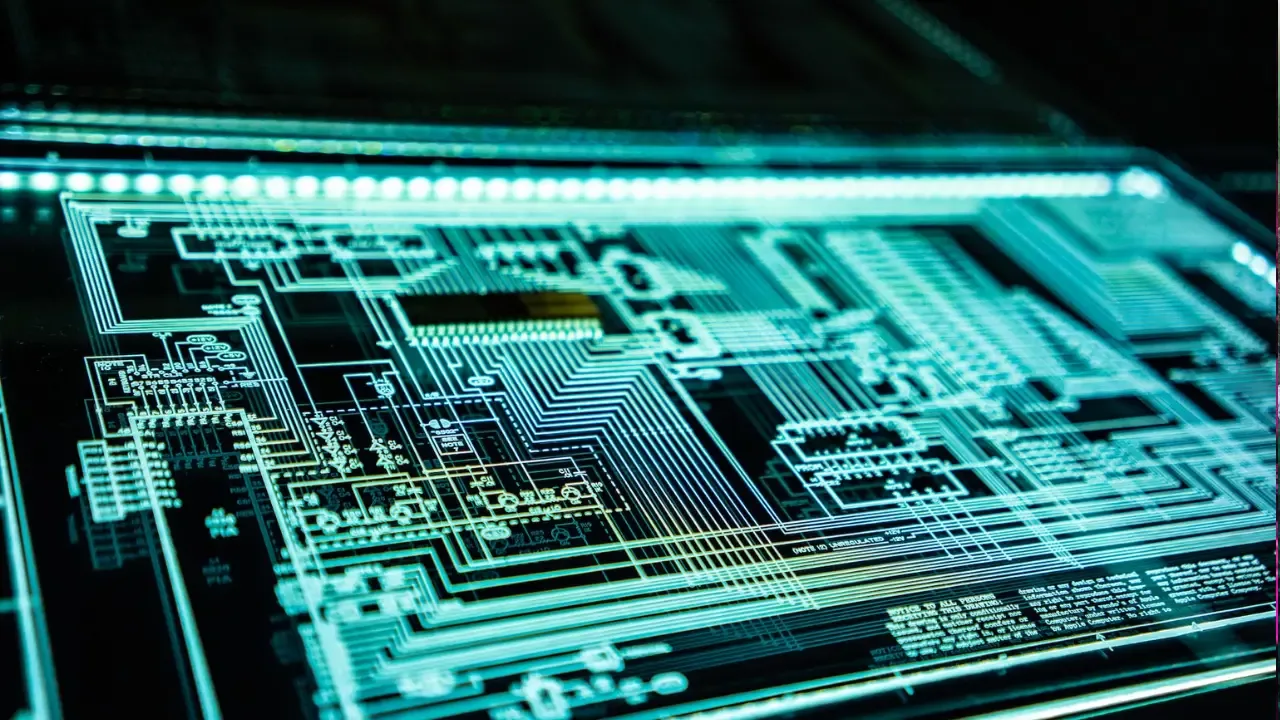
š Title: How to Handle jQuery Checkbox Change and Click Events Confidently
š¤ Introduction: We've all faced the challenge of updating the textbox value based on checkbox status and confirming uncheck actions using jQuery. However, this process can lead to inconsistent states and incorrect textbox values. In this blog post, we'll explore a common problem faced by developers and provide easy solutions to keep the textbox value consistent with the check state.
š Problem:
The current implementation uses the .change()
and .click()
events to update the textbox value based on checkbox status and confirm the action on uncheck. However, if the user cancels the action, the textbox value remains inconsistent with the check state.
š” Solution: To ensure consistent textbox values, follow these steps:
Step 1: Add a new variable
isChecked
to keep track of the checkbox status.$(document).ready(function() { var isChecked = $('#checkbox1').is(':checked'); $('#textbox1').val(isChecked); });
Step 2: Modify the
.change()
event to update the textbox value and update theisChecked
variable.$('#checkbox1').change(function() { isChecked = $(this).is(':checked'); $('#textbox1').val(isChecked); });
Step 3: Refactor the
.click()
event to use the updatedisChecked
variable and handle the checkmark restoration.$('#checkbox1').click(function() { if (!isChecked) { return confirm("Are you sure?"); } });
By using the
isChecked
variable, we can ensure that the textbox value remains consistent with the check state, regardless of the user's confirmation decision.
ā Example: Let's see the updated code implementation in action:
$(document).ready(function() {
var isChecked = $('#checkbox1').is(':checked');
$('#textbox1').val(isChecked);
$('#checkbox1').change(function() {
isChecked = $(this).is(':checked');
$('#textbox1').val(isChecked);
});
$('#checkbox1').click(function() {
if (!isChecked) {
return confirm("Are you sure?");
}
});
});
šÆ Call-to-Action: Now that you know how to handle the checkbox change and click events confidently, try implementing these changes in your code to keep your textbox values consistent with the check state. Share your experiences or any additional tips in the comments below!
š£ Conclusion: Updating the textbox value based on checkbox status and handling the confirmation on uncheck can be tricky. However, by using the provided solution, we can ensure consistent textbox values and improve the overall user experience. Remember to implement these changes in your code and share your success stories with us!
Keep coding and stay consistent! š„ļøšŖ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
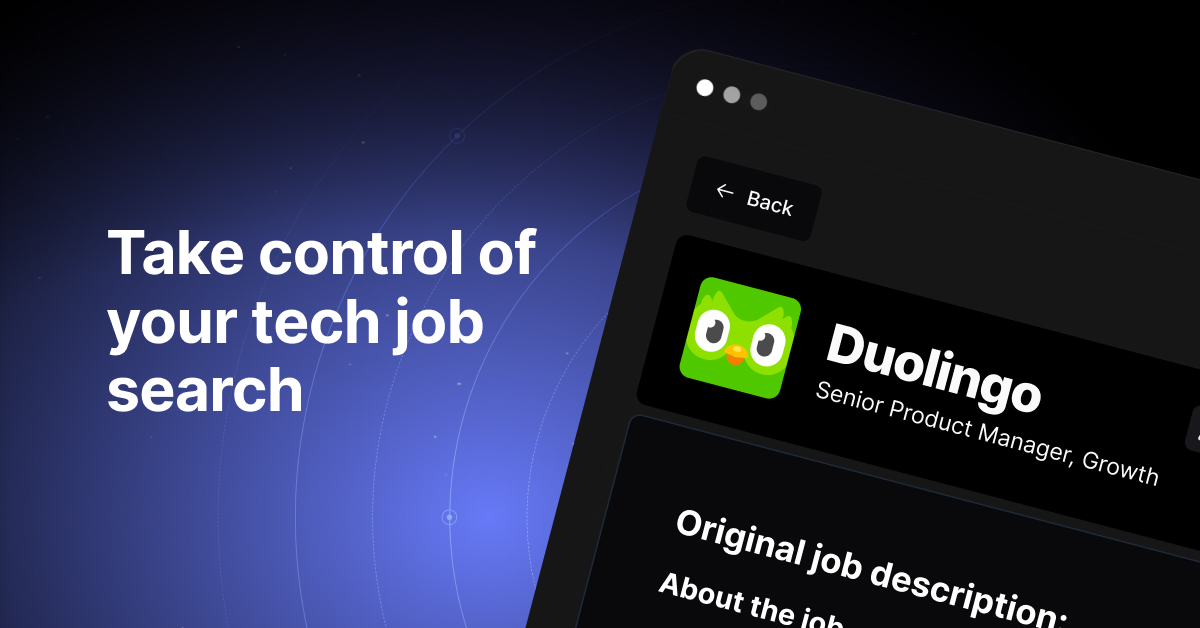