jQuery AJAX file upload PHP
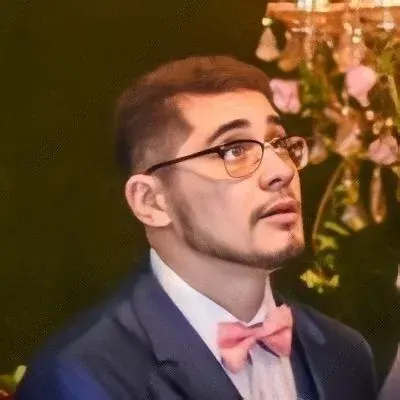
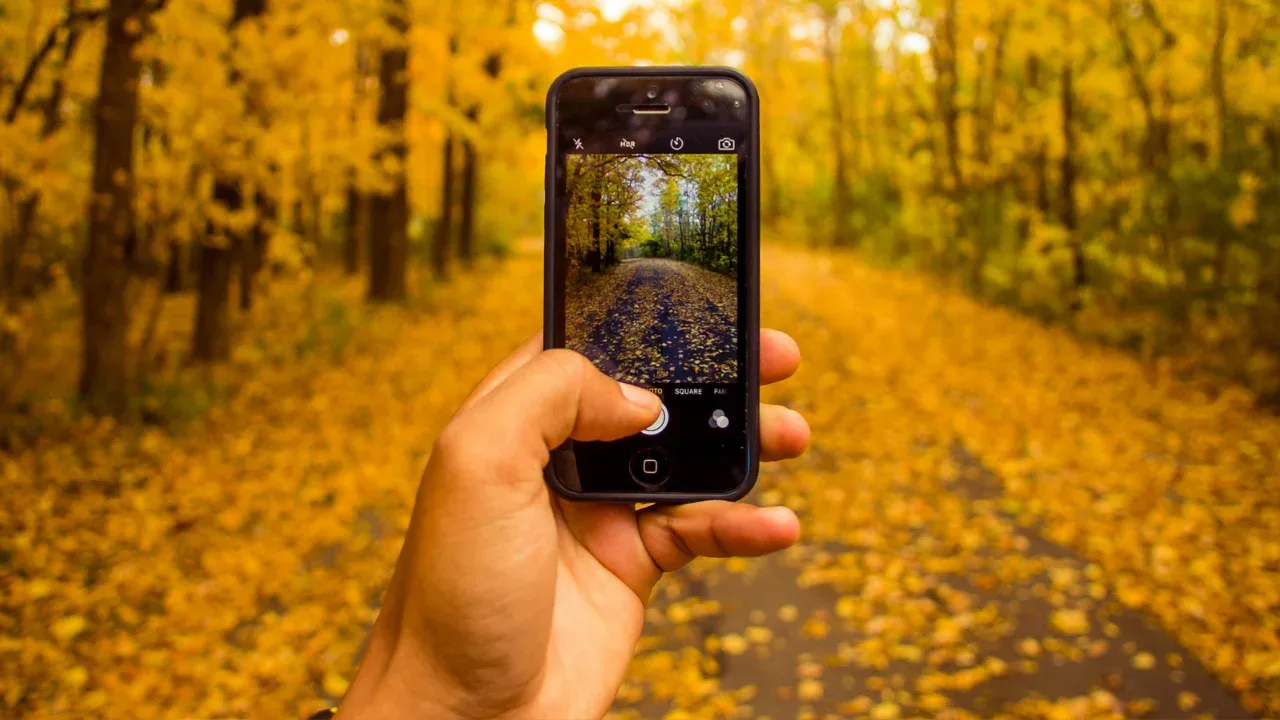
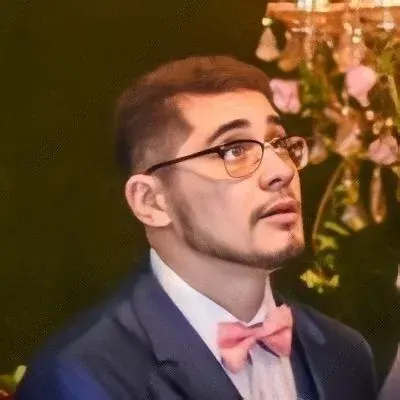
jQuery AJAX File Upload with PHP 📁
File uploads can be a common requirement in web development, and luckily, jQuery AJAX along with PHP can make this task fairly simple. In this tutorial, we'll cover how to implement a basic file upload feature using the smallest setup possible.
The HTML Part 🖥️
Let's start by setting up the HTML part of our file upload feature. Add the following code snippet to your HTML file:
<input id="sortpicture" type="file" name="sortpic" />
<button id="upload">Upload</button>
Here, we have an input element with the id sortpicture
and a button element with the id upload
. These elements will allow users to select a file and trigger the file upload process.
The JavaScript jQuery Script 📜
To handle the file upload functionality, we'll use JavaScript with jQuery. Include the following script in your JavaScript file or within a script tag:
$("#upload").on("click", function() {
var file_data = $("#sortpicture").prop("files")[0];
var form_data = new FormData();
form_data.append("file", file_data);
$.ajax({
url: "/uploads",
dataType: 'script',
cache: false,
contentType: false,
processData: false,
data: form_data,
type: 'post',
success: function(){
alert("File uploaded successfully!");
}
});
});
In this code snippet, we are listening for a click event on the upload
button. When the button is clicked, we grab the selected file using $("#sortpicture").prop("files")[0]
. Then, we create a FormData
object and append the file to it.
Next, we use AJAX to send the form data to the server. Here are the key parameters used in the AJAX request:
url
: The URL where the file should be uploaded. In this case, it's/uploads
.dataType
: The expected data type of the response. We've set it to'script'
, but you can modify it according to your needs.cache
: Set tofalse
to ensure the latest version of the file is uploaded.contentType
andprocessData
: Both set tofalse
to prevent jQuery from automatically processing the data.data
: The form data that contains the file to be uploaded.type
: The HTTP method to be used for the request. In this case, it's'post'
.
Finally, in the success callback function, a simple alert is shown to indicate that the file was uploaded successfully.
Troubleshooting Common Issues ❗
Now let's address the specific issue mentioned in the original question: when the file is selected and the upload button is clicked, the first alert displays [object FormData]
, the second alert is not triggered, and the uploads
folder remains empty.
The first alert displays [object FormData]
because it's showing the string representation of the FormData
object. This is expected behavior and doesn't indicate any error.
The second alert not being triggered and the empty uploads
folder could be due to a couple of potential issues:
Folder Permissions: Ensure that the "uploads" folder in the root directory of your website has the necessary write permissions for both "users" and "IIS_users". You can typically do this through your server's file management system or using command-line tools.
Server-Side File Handling: Make sure you have the appropriate PHP code to handle the uploaded file and save it to the desired location. You can use PHP's built-in
move_uploaded_file
function to move the file from the temporary directory to the desired destination.
Renaming the Uploaded File 🔄
As for renaming the uploaded file with a server-side generated name, here's an example PHP code snippet to accomplish that:
$uploadDirectory = "/path/to/uploads/"; // Replace with your actual upload directory
$originalName = $_FILES['file']['name'];
$extension = pathinfo($originalName, PATHINFO_EXTENSION);
$generatedName = generateUniqueName() . '.' . $extension; // Implement your own function to generate a unique name
if (move_uploaded_file($_FILES['file']['tmp_name'], $uploadDirectory . $generatedName)) {
// File uploaded successfully
// Perform any further actions if needed
echo $generatedName; // Return the generated name to the client-side for reference or further processing
} else {
// Error occurred during file upload
// Handle the error appropriately
echo "Upload failed";
}
In this PHP snippet, we obtain the original name of the uploaded file using $_FILES['file']['name']
. Then, we extract the file extension using pathinfo
and generate a unique name using a custom function (generateUniqueName()
).
Finally, we move the file from the temporary directory ($_FILES['file']['tmp_name']
) to the desired upload directory ($uploadDirectory
) with the newly generated name. If the file is moved successfully, we can perform any further actions or return the generated name to the client-side for reference.
Call-to-Action and Reader Engagement 📣
I hope this guide helps you overcome any hurdles you may face with jQuery AJAX file uploads using PHP. Give it a try and let me know in the comments if you encountered any issues or if you found the solution helpful. 🙌
If you have any additional questions or topics you'd like me to cover, feel free to reach out. Happy coding! 💻