jQuery Ajax File Upload
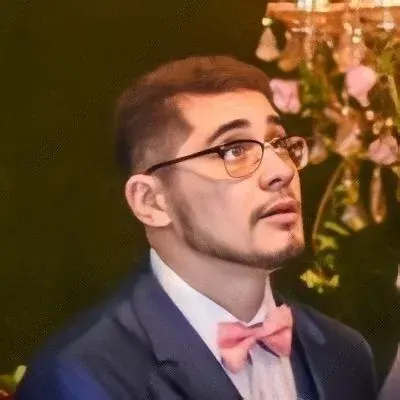
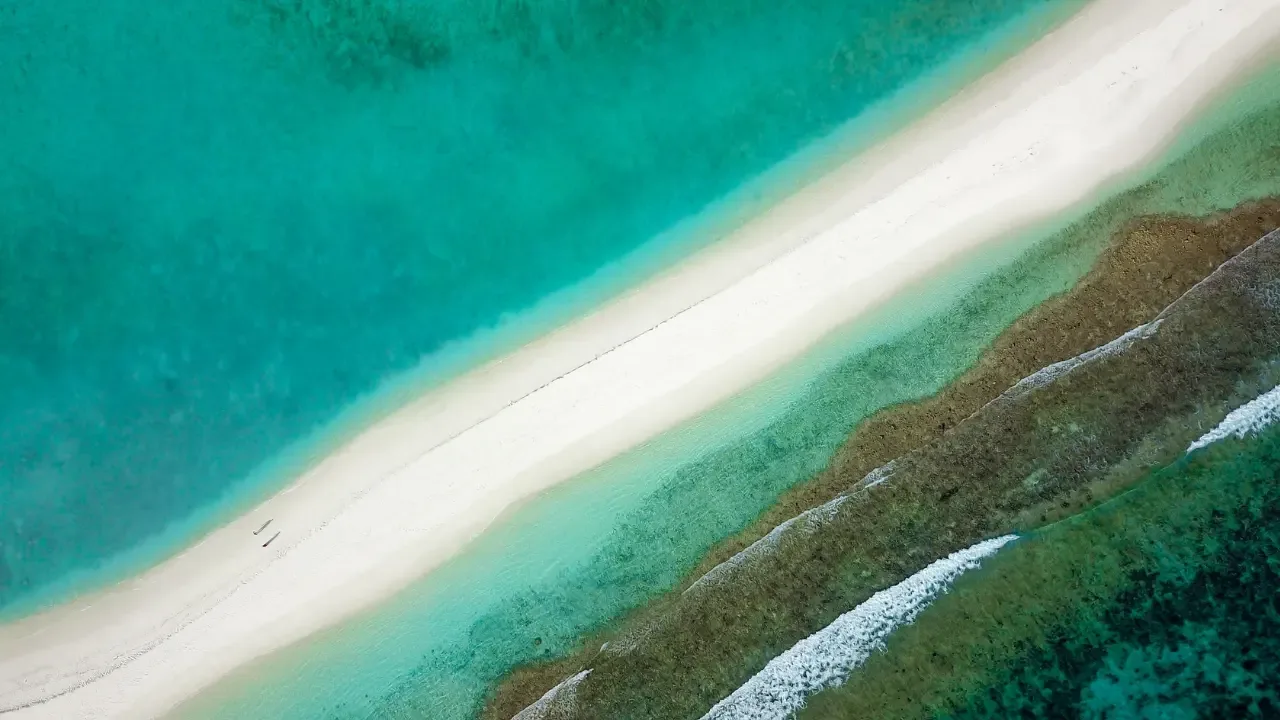
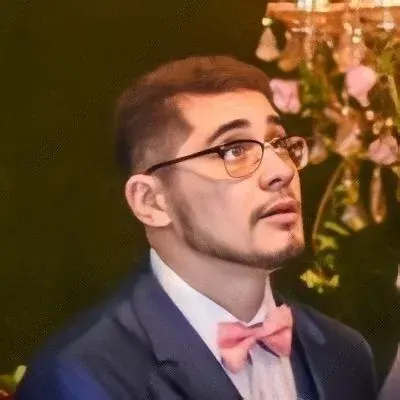
🌟 Easy File Upload with jQuery Ajax 🌟
Are you tired of googling around and finding complicated plugins for file uploads? Look no further! In this blog post, we'll show you how to perform a file upload using the jQuery Ajax POST method, without the need for a plugin. 📁📤
🔑 The Code:
Let's take a look at the code snippet you provided:
$.ajax({
type: "POST",
timeout: 50000,
url: url,
data: dataString,
success: function (data) {
alert('success');
return false;
}
});
This code is using jQuery's $.ajax()
function with the POST method. It sets the URL for the server to handle the file upload and specifies the data to be sent. However, it seems like you are unsure about the data
part and whether it needs to be filled. Let's address that next. 💡
🔍 The Data:
To perform a file upload using Ajax, you do need to populate the data
property. But what exactly should you put there? 🤔
In the case of file uploads, instead of sending the file itself as a string, you need to send it as a FormData
object. This object allows you to append files and other data to be sent to the server. Here's an example:
var formData = new FormData();
formData.append('file', file); // 'file' is the name you assign to the file input
$.ajax({
type: "POST",
timeout: 50000,
url: url,
data: formData,
processData: false, // Required for sending FormData objects
contentType: false, // Required for sending FormData objects
success: function (data) {
alert('Success!');
return false;
}
});
By creating a FormData
object and appending your file to it, you can ensure that the file is correctly sent to the server. The processData
and contentType
options should be set to false
to prevent jQuery from automatically processing the data or setting the content type.
✅ The Solution:
In summary, to perform a file upload using jQuery Ajax without a plugin, make sure to:
Create a
FormData
object.Append the file (obtained from the file input) to the
FormData
object.Pass the
FormData
object as the value for thedata
property in the Ajax request.Set the
processData
andcontentType
options tofalse
.
📣 Let's Take Action:
Now that you have the solution, it's time to put it to use! Try implementing this code in your project and enjoy hassle-free file uploads with jQuery Ajax. If you have any further questions or run into any issues, leave a comment below. We're here to help! 🤝💻
Happy coding! 😄🚀