JavaScript set object key by variable
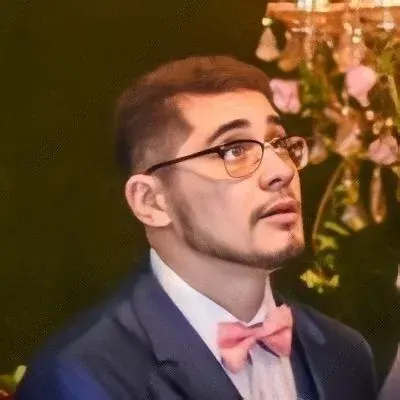
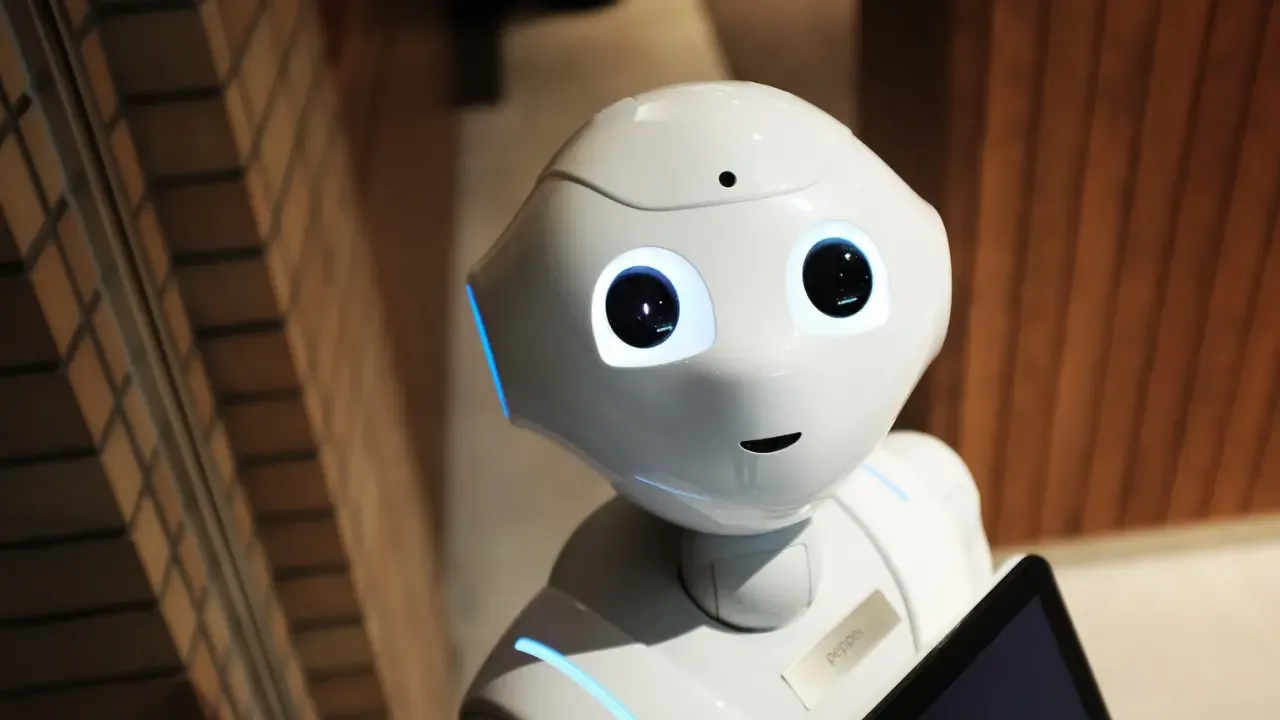
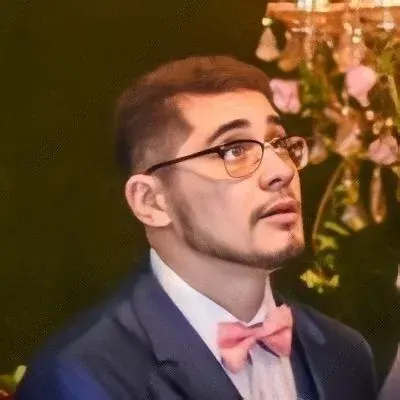
JavaScript: Setting Object Key by Variable 🗝️
Are you trying to set an object key using a variable in JavaScript? You're not alone! This is a common issue that many developers face when working with objects and arrays. But fret not, because I'm here to provide you with easy solutions to this problem. Let's dive in! 💪
The Problem 😫
Let's take a look at the code snippet provided in the context:
var key = "happyCount";
myArray.push( { key : someValueArray } );
In this example, the developer wants to use the value of the key
variable as the key for each object pushed into the myArray
. However, when examining the array, they find that the key is always "key"
instead of the desired value of the variable key
.
The Explanation 🧠
The issue here is due to the way JavaScript interprets object literals. When an object literal is defined, the key is expected to be a literal value. In other words, it's treated as a string and not as a variable. So, in the code snippet above, the key is literally set as "key"
instead of the value of the key
variable.
The Solutions 🛠️
Thankfully, there are a few simple solutions to solve this problem. Let's explore two common approaches:
Solution 1: Bracket Notation
One way to dynamically set the key of an object using a variable is by using bracket notation. Instead of using dot notation to define the key, we can enclose the variable name in square brackets.
var key = "happyCount";
var obj = {};
obj[key] = someValue;
By using bracket notation, we can now assign the value someValue
to the object's key, which is the value of the key
variable.
Solution 2: Computed Property Names (ES6+)
If you're using ECMAScript 6 (ES6) or a newer version of JavaScript, you can leverage the computed property names feature. This allows you to use an expression in square brackets to define an object's key.
const key = "happyCount";
const obj = {
[key]: someValue,
};
Using computed property names, we can directly set the key of the object to the value of the key
variable.
See It in Action! 💥
If you want to experiment with these solutions, you can check out this JSFiddle that demonstrates the code in action: JSFiddle - Setting Object Key by Variable.
Conclusion and Call-to-Action 📝
Setting an object key by variable in JavaScript doesn't have to be a tricky business. By using bracket notation or computed property names, you can easily assign dynamic keys to your objects.
So next time you face this issue, remember the solutions I've shared with you. Give them a try and see which one works best for your situation. And don't forget to share your experience with me in the comments below. Happy coding! 😊🚀