Javascript : Send JSON Object with Ajax?
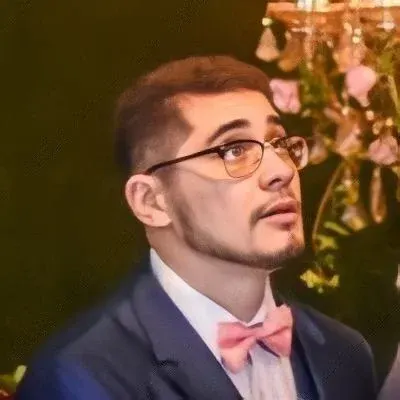
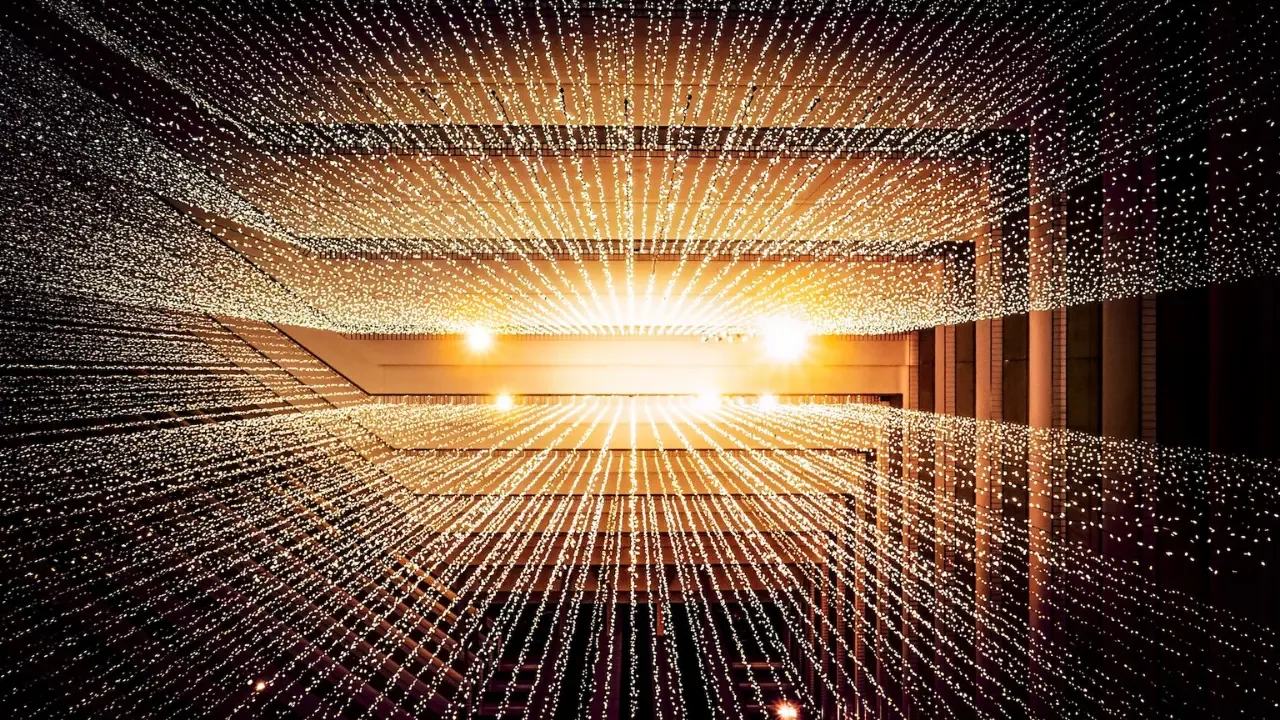
🌐 Javascript: Send JSON Object with Ajax? 📤
So, you're building a web application and want to send a JSON object with Ajax? I hear you, my friend! Sending JSON objects with Ajax can unlock endless possibilities in your web development journey. 💪✨
The Challenge 🤔
You found yourself pondering this question:
<p>Is this possible?</p>
<pre><code>xmlHttp.send({
"test" : "1",
"test2" : "2",
});
</code></pre>
The Simple Solution 🎉
The answer is YES! Sending a JSON object with Ajax is absolutely possible, as long as you follow a few steps. Let's dive into the solution and simplify things for you!
Step 1: Set the "Content-Type" Header ✔️
You need to set the "Content-Type" header to inform the server that you're sending JSON data. There are two options available:
application/json
- Use this if you want to send the JSON object as a JSON payload.xmlHttp.setRequestHeader('Content-Type', 'application/json');
application/x-www-form-urlencoded
- Use this if you prefer to stringify the JSON object and send it as a parameter.xmlHttp.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded');
Step 2: Send the JSON Object 📩
Now that the "Content-Type" header is set correctly, you can send the JSON object using the send
method. Remember to convert the JSON object to a string using JSON.stringify()
:
xmlHttp.send(JSON.stringify({
"test" : "1",
"test2" : "2",
}));
One Last Thing: JSON != JavaScript Object ❗
It's crucial to understand that JSON and JavaScript objects are not the same. JSON stands for JavaScript Object Notation, and it represents data as a string. So, you need to parse the JSON response from the server to access its properties.
Your Turn to Shine! 🌟
Now that you have the solution to send JSON objects with Ajax in your toolkit, you can level up your web development game. Start experimenting, building amazing features, and let your creativity soar!
If you found this guide helpful or have any questions, don't hesitate to leave a comment, share your experience, or reach out to me on social media. Let's keep the conversation going! 👏🗣️
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
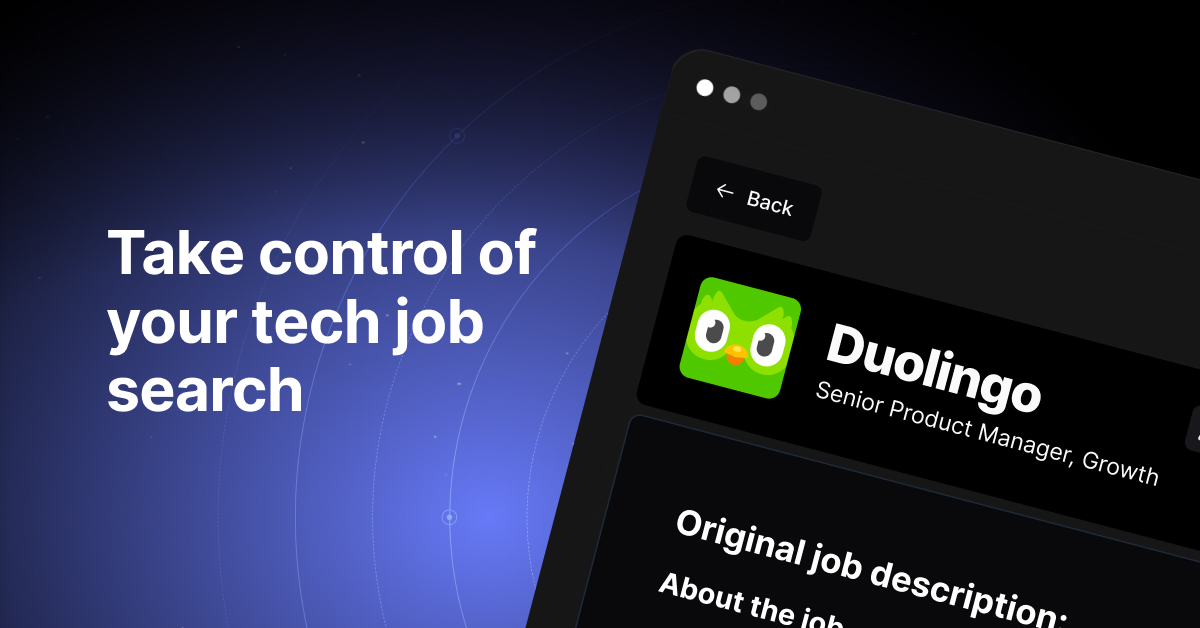