Javascript replace with reference to matched group?
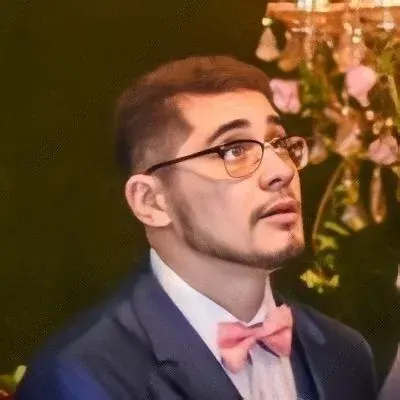
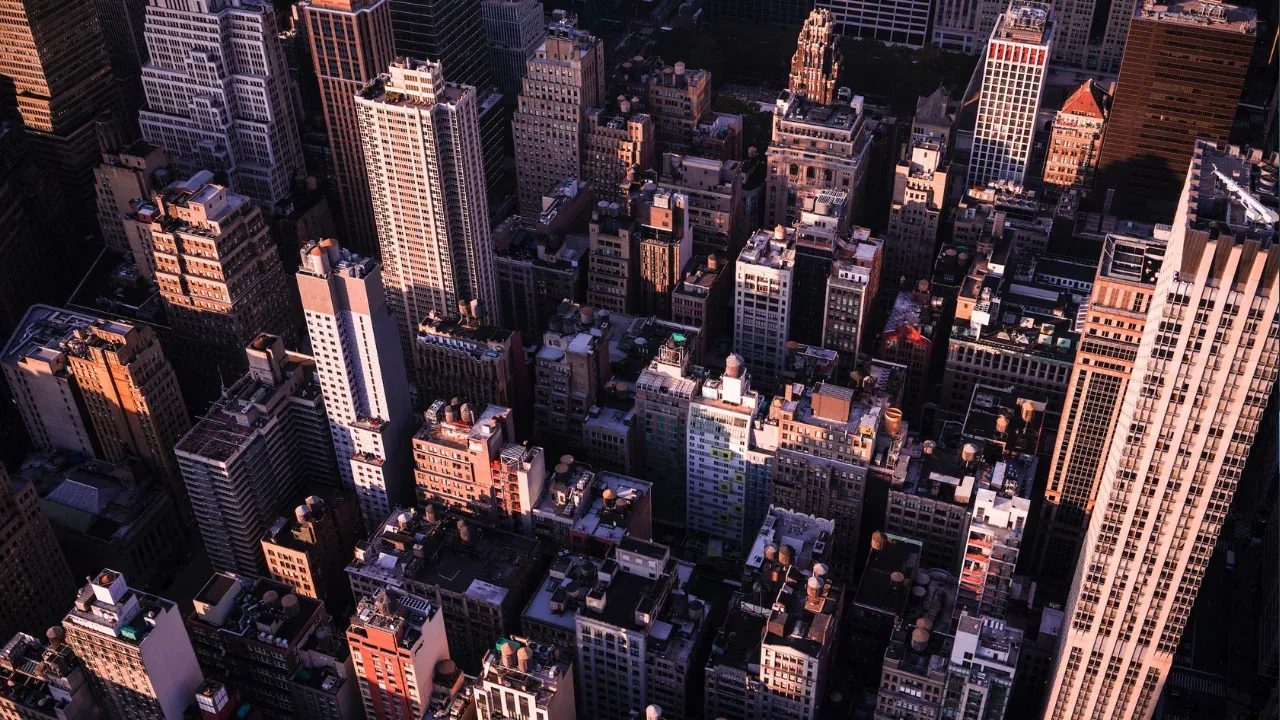
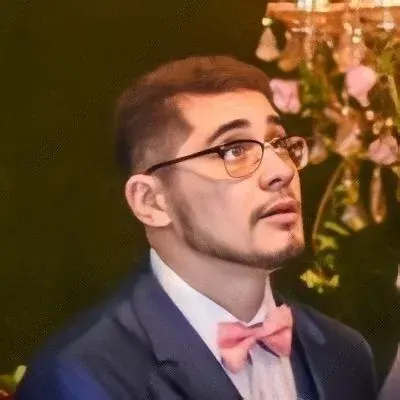
Easy JavaScript Solution to Replace Matched Group with References
So you have a string, and you want to replace a certain pattern with a reference to the matched group using JavaScript. 🔄
Let's say you have a string like this:
const str = "hello _there_";
And you want to replace the underscores with <div>
and </div>
respectively, so the output looks like this:
const output = "hello <div>there</div>";
Sounds like a common issue. But don't worry, I've got your back! 🤩 Here are two easy solutions:
Solution 1: Using the replace()
Method
You can use the replace()
method in JavaScript along with a regular expression to achieve the desired result. Here's how you can do it:
const replacedStr = str.replace(/_(.*?)_/g, "<div>$1</div>");
In the regular expression /_(.*?)_/g
:
_
indicates the underscore character we want to replace,(.*?)
represents the matched group,g
is the global flag to match all instances in the string.
By using $1
in the replacement string, we can reference the matched group within the <div>
tags.
You can see the code in action here:
const str = "hello _there_";
const replacedStr = str.replace(/_(.*?)_/g, "<div>$1</div>");
console.log(replacedStr); // Output: "hello <div>there</div>"
Solution 2: Using a Callback Function
Alternatively, you can use a callback function with the replace()
method to achieve the same result. Here's an example:
const replacedStr = str.replace(/_(.*?)_/g, (match, group) => `<div>${group}</div>`);
In this case, the callback function takes two parameters:
match
: represents the matched group (same as before),group
: represents the captured group (in this case, whatever is between the two underscores).
You can see the code in action here:
const str = "hello _there_";
const replacedStr = str.replace(/_(.*?)_/g, (match, group) => `<div>${group}</div>`);
console.log(replacedStr); // Output: "hello <div>there</div>"
Your Turn! 💪
Now that you have the solutions, it's time to put them to the test! Try them out with your own examples and see the magic happen. ✨
And if you have any other cool ideas or suggestions, feel free to share them in the comments below. Let's make this post even better together! 🤩
🎉 Happy coding! 🚀