JavaScript regex multiline text between two tags
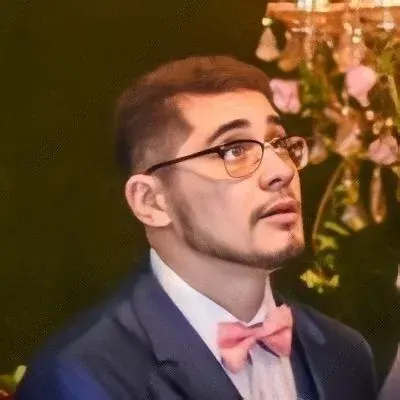
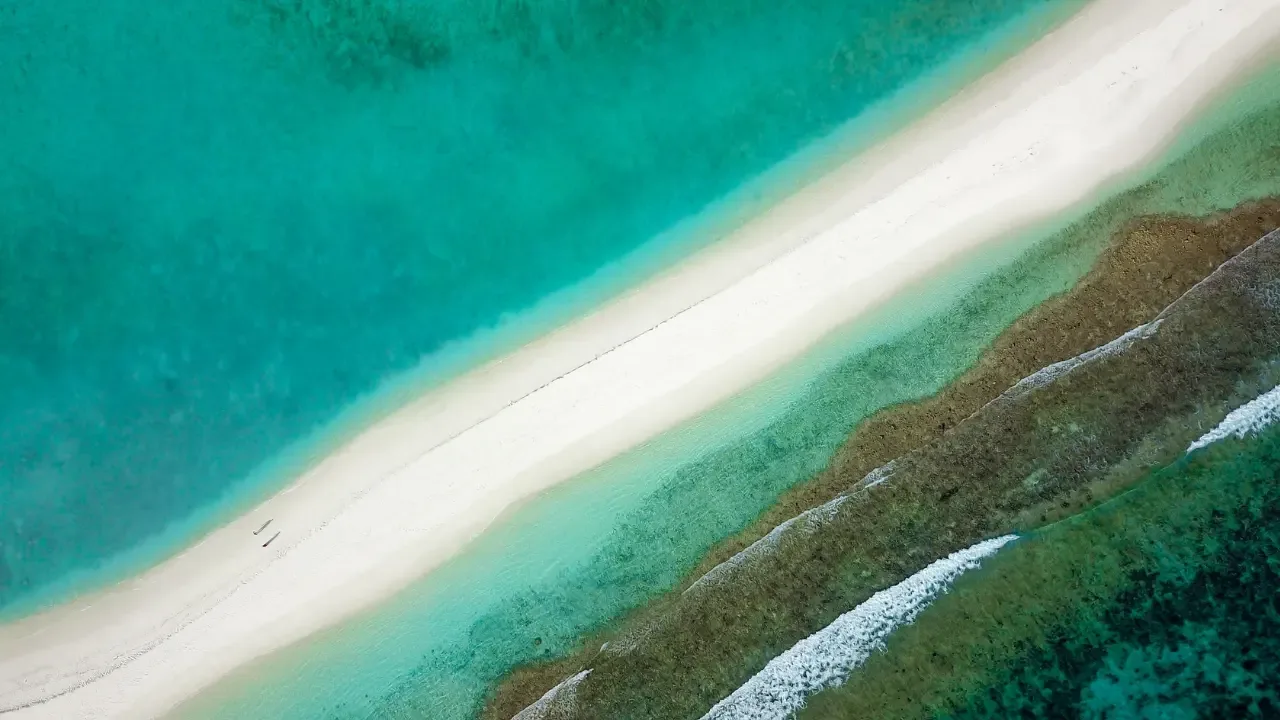
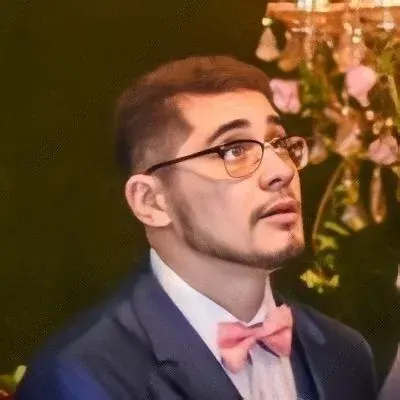
š Easy Solution for JavaScript Regex Multiline Text between Two Tags š
š¤ Are you struggling with your JavaScript regex to fetch the text between two tags, only to find that the multiline flag is not working as expected? We've got you covered! In this blog post, we'll address this common issue and provide you with an easy solution.
What's the Problem?
š Let's start by examining the problem you mentioned. You wrote a regex pattern to extract the text within an <h1>
tag from an HTML string. However, you noticed that the pattern fails when the text spans multiple lines and contains line breaks (\n
).
The Pattern
š¤ Here's the pattern you shared:
var pattern= /<div class="box-content-5">.*<h1>([^<]+?)<\/h1>/mi;
m = html.search(pattern);
return m[1];
Within the pattern, you use the /m
flag to enable multiline matching. However, the results are not as expected when there are line breaks in the text.
The Issue with Line Breaks
š„ The issue lies with how the dot (.
) character interacts with line breaks. By default, the dot matches any character except a line break. That's why your pattern fails when the text contains line breaks.
The Solution: Using the Dot-All Flag š
⨠The Dot-All flag (/s
) is the key to solving this problem. It allows the dot (.
) to match any character, including line breaks. Let's update your code:
var pattern = /<div class="box-content-5">.*<h1>([^<]+?)<\/h1>/ms; // Note the change!
m = html.search(pattern);
return m[1];
By replacing the /mi
flags with /ms
, you now enable the Dot-All behavior and ensure that the dot matches line breaks.
Testing the Improved Regex
šµļøāāļø Let's put this solution to the test with a sample string. Consider the following string with line breaks:
<div class="box-content-5">
<h1>This is a
multiline title</h1>
</div>
With the improved regex, you can now successfully extract the multiline title.
Call-to-Action: Share Your Regex Struggles!
š Congratulations, you're now equipped with an easy solution to overcome JavaScript regex issues when dealing with multiline text between two tags. If you found this blog post helpful, make sure to share it with your fellow developers.
š¬ Have you encountered any other regex challenges? Let us know in the comments below, and we'll be more than happy to help you find solutions!
Happy coding! š