Javascript Regex: How to put a variable inside a regular expression?
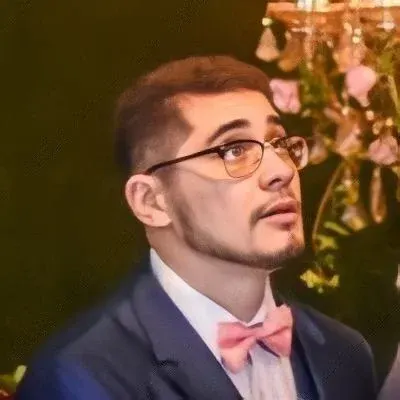
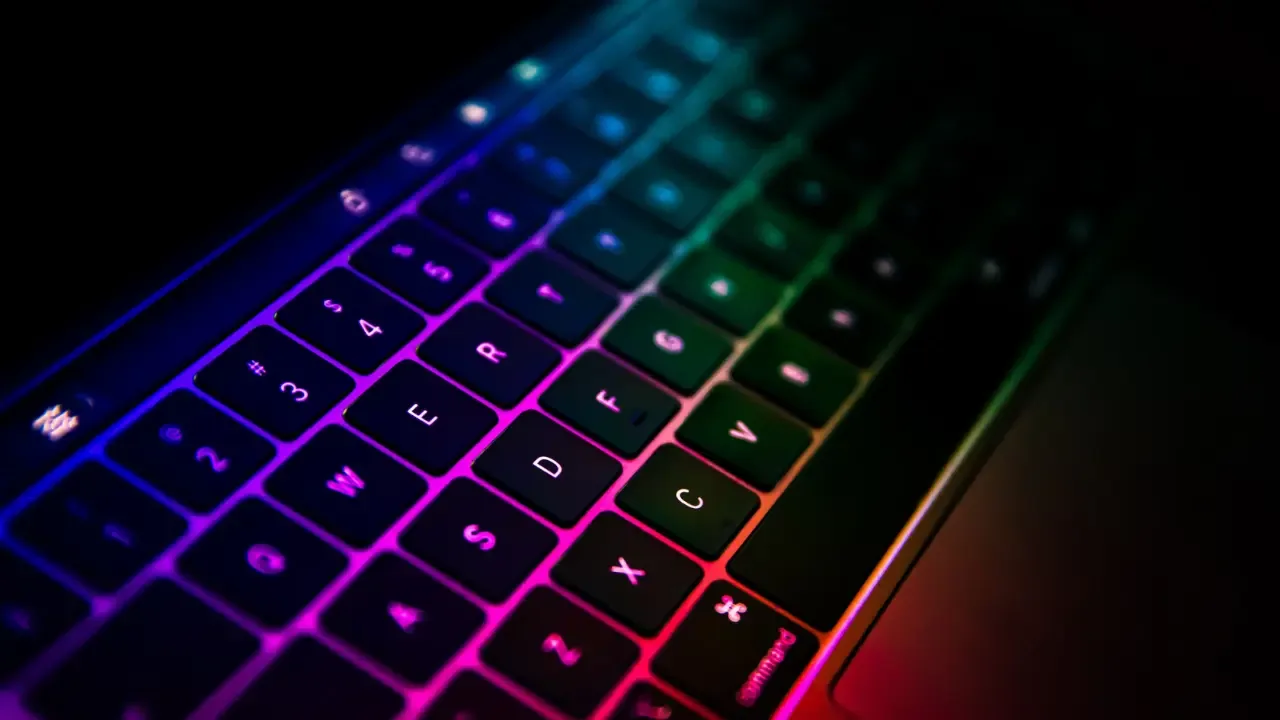
How to Put a Variable Inside a JavaScript Regex?
Have you ever come across a situation where you need to use a variable inside a regular expression in JavaScript? 🤔 It can be a bit tricky, but fear not! In this blog post, we will explore common issues and provide easy solutions to help you conquer this challenge. Let's dive in! 💪
The Challenge
Let's say you have a JavaScript function that takes an input and a test variable. You want to replace a specific pattern in a string with a replacement, but the pattern includes the test variable. Here's an example to illustrate the problem:
function(input){
var testVar = input;
string = ...
string.replace(/ReGeX + testVar + ReGeX/, "replacement")
}
In this code snippet, our goal is to replace a pattern surrounded by "ReGeX" with the word "replacement", but using the testVar
variable inside the regular expression. Unfortunately, this approach doesn't work as expected.
Solution 1: Using the RegExp
Constructor
To use a variable inside a regular expression in JavaScript, you can leverage the RegExp
constructor. Here's how you can modify your code to make it work:
function(input){
var testVar = input;
var regex = new RegExp("ReGeX" + testVar + "ReGeX", "g");
string = ...
string = string.replace(regex, "replacement");
}
By creating a new RegExp
object, we can concatenate the desired pattern with the testVar
variable inside the regular expression. The "g"
parameter passed as the second argument to the RegExp
constructor ensures that it replaces all occurrences of the pattern in the string.
Solution 2: Using Template Literals and the RegExp
Constructor
ES6 introduced template literals, which are handy for string interpolation. We can leverage template literals along with the RegExp
constructor to simplify our code even further:
function(input){
var testVar = input;
string = ...
string = string.replace(`ReGeX${testVar}ReGeX`, "replacement");
}
Using template literals, we can directly insert the testVar
variable inside the regular expression by enclosing it in ${}
. This approach eliminates the need for explicit concatenation and makes the code more readable.
Empowering Your Regex Skills! 🚀
Now that you know how to put a variable inside a JavaScript regex, you can tackle more complex scenarios and unleash the full power of regular expressions! Whether you're validating user input, extracting data, or manipulating strings, regex can be your best friend.
Feel free to experiment and adapt these techniques to suit your specific needs. And if you have any cool uses of regex with variables, let us know in the comments below! We would love to see your creative implementations. 😎
Happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
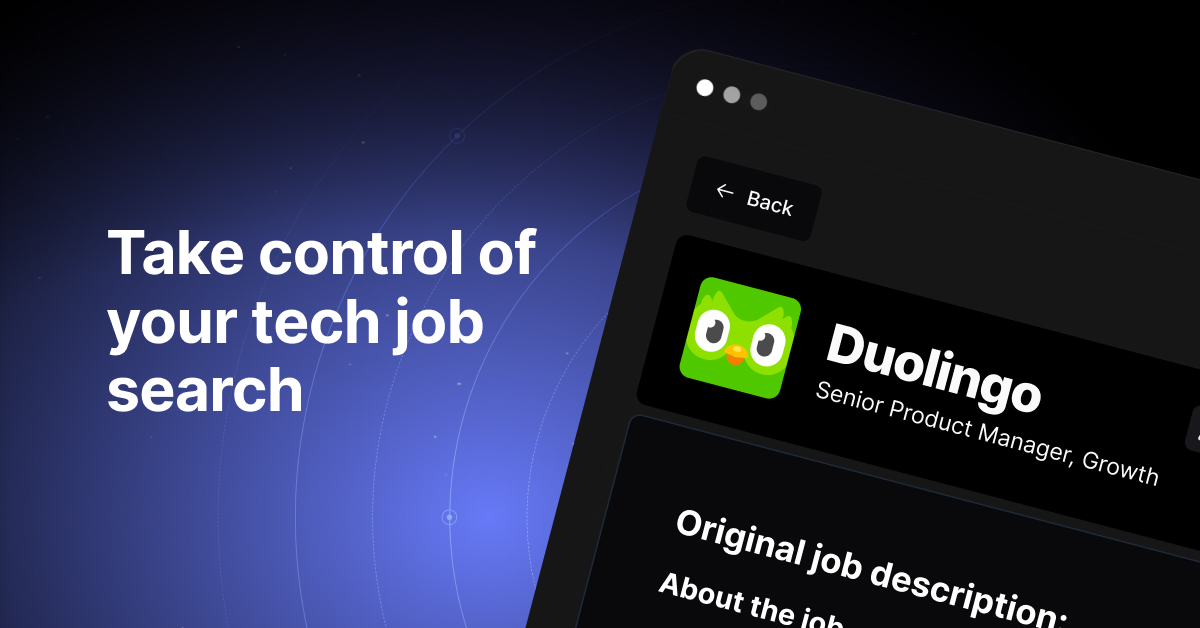