JavaScript post request like a form submit
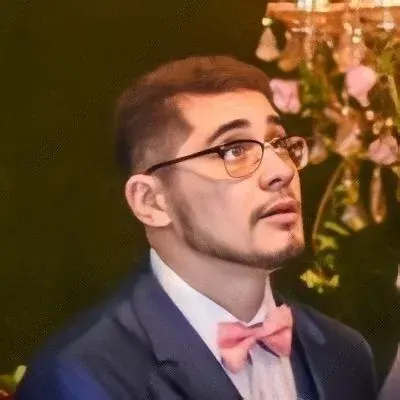
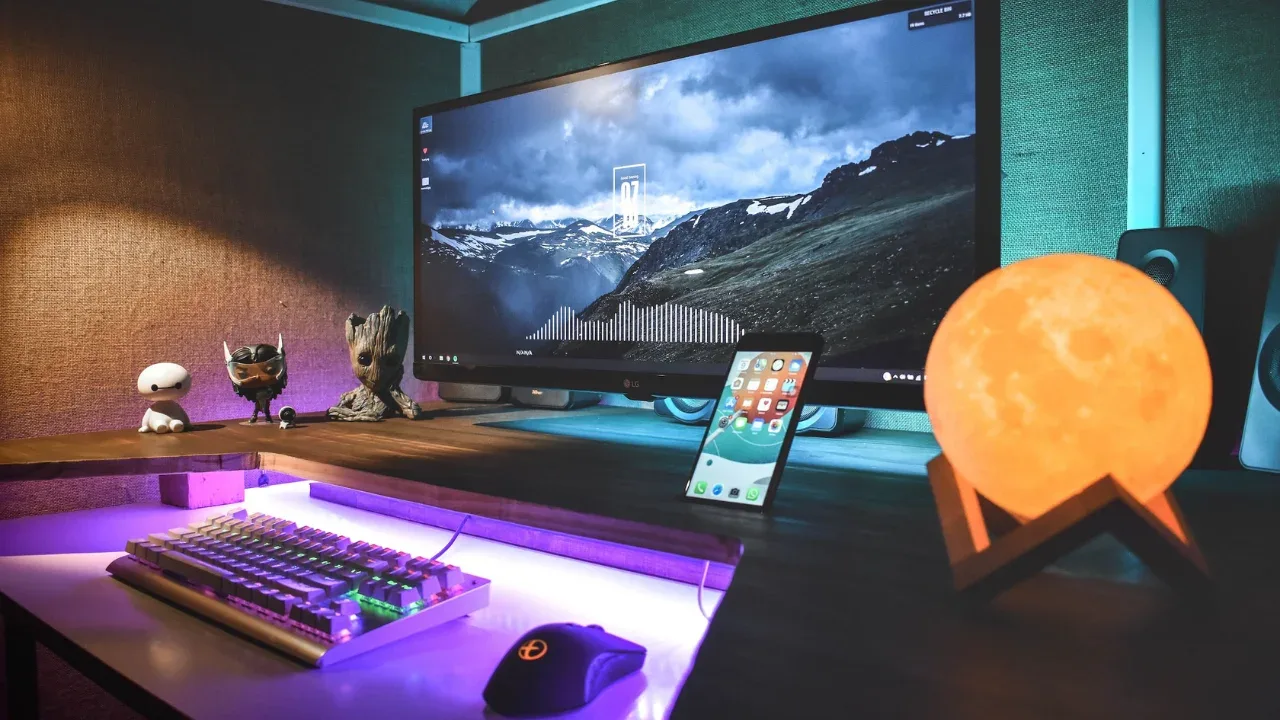
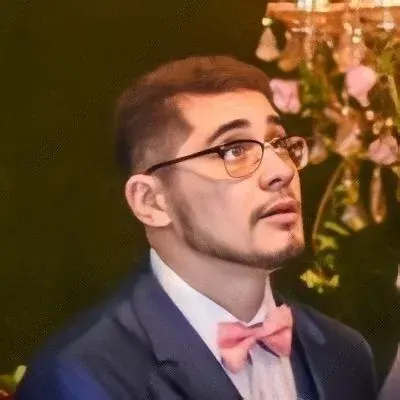
๐JavaScript Post Request Made Easy: Submitting a Form in Style! ๐
Are you tired of struggling with JavaScript post requests that don't work like submitting a form? Well, fret no more! In this blog post, we'll explore the best cross-browser implementation that allows you to change the browser's location, just like submitting a form. No asynchronous or XML mumbo jumbo - we're keeping it simple and effective! ๐
Understanding the Problem
Let's take a look at our example scenario. You want to direct the browser to a different page but using a POST request instead of a GET request. You may have tried using document.location.href
for GET requests and wondered how to achieve the same effect with a POST request.
If the resource you're trying to access allows only POST requests, a plain URL change won't suffice. You need an alternative method that simulates the behavior of submitting a form, but with JavaScript. Exciting, right? Let's dive into the solution!
The Easy Solution: post_to_url()
Function ๐
To achieve a JavaScript post request that emulates form submission, we'll make use of a custom post_to_url()
function. This versatile function will allow you to specify the URL and data you want to send, all while maintaining the simplicity and efficiency you desire. Check out the code snippet below:
function post_to_url(url, data) {
const form = document.createElement('form');
form.method = 'POST';
form.action = url;
for (const key in data) {
if (data.hasOwnProperty(key)) {
const hiddenField = document.createElement('input');
hiddenField.type = 'hidden';
hiddenField.name = key;
hiddenField.value = data[key];
form.appendChild(hiddenField);
}
}
document.body.appendChild(form);
form.submit();
}
How to Use the post_to_url()
Function ๐ค
Using this function is a piece of cake! Simply follow these steps:
Copy the
post_to_url()
function to your JavaScript file.Call the
post_to_url()
function wherever you want to trigger the POST request, providing the URL and the data as arguments.
Here's an example usage:
post_to_url('http://example.com/', {'q':'a'});
In this example, we're sending a POST request to http://example.com/
with the data object {'q':'a'}
. Feel free to customize the URL and data according to your specific needs.
The Browser Compatibility Advantage ๐ฅ
You might be wondering if this solution is compatible with all major browsers. Rest assured, the post_to_url()
function works seamlessly across browsers, ensuring a smooth experience for all your users. It's time to leave behind your cross-browser worries and embrace this hassle-free solution!
Engage with Us! ๐ข
We hope this blog post has helped you master the art of JavaScript post requests that mimic form submission. Now it's your turn to engage with us! Let us know in the comments if you found this solution helpful, or if you have any other JavaScript-related questions or topics you'd like us to cover in future blog posts.
Happy coding! ๐ปโจ