JavaScript, Node.js: is Array.forEach asynchronous?
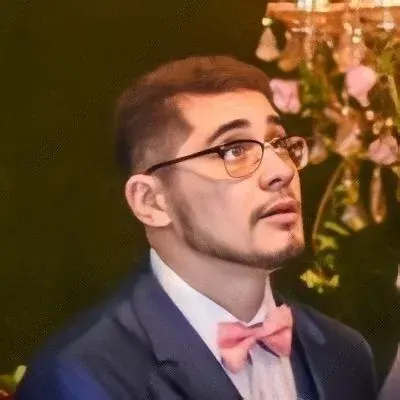
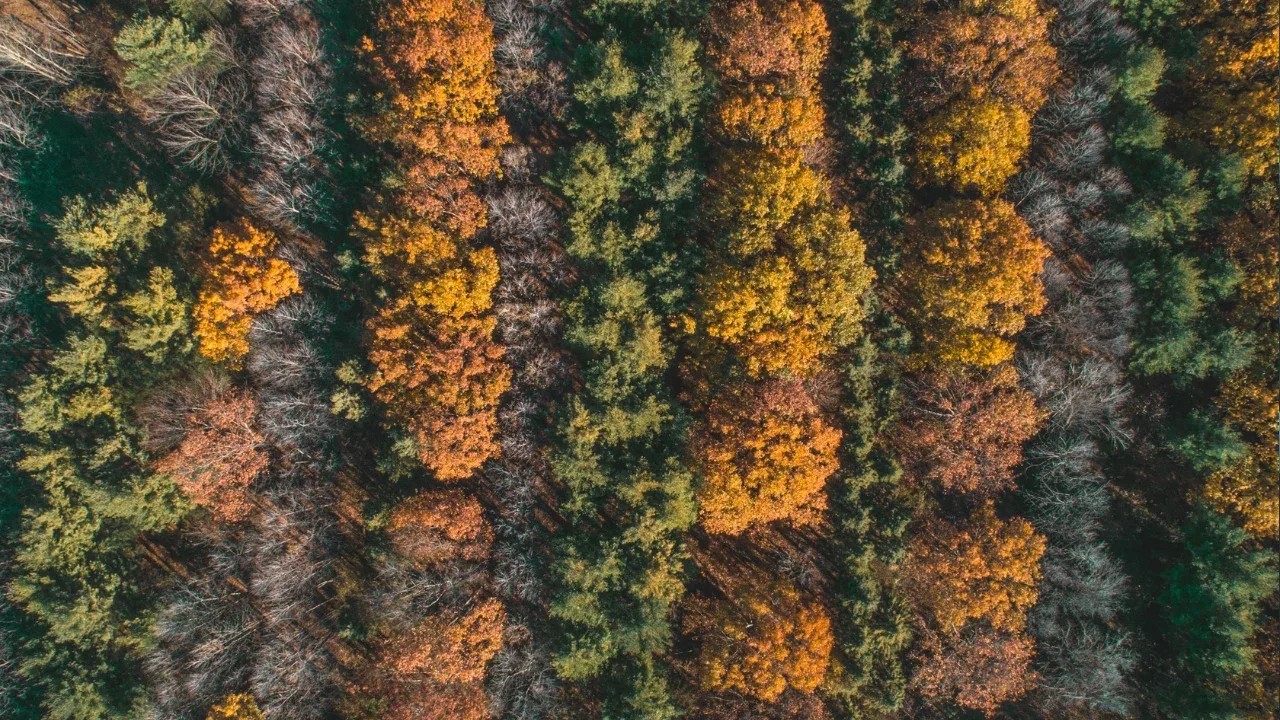
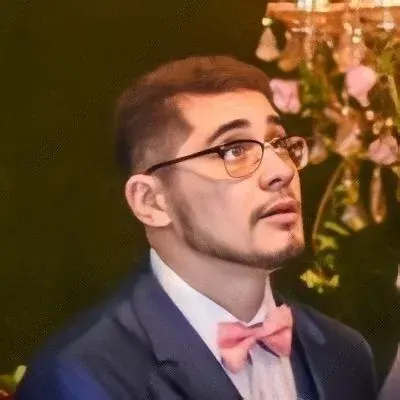
Is Array.forEach Asynchronous in JavaScript and Node.js? 🤔
Are you wondering if the Array.forEach
method in JavaScript and Node.js is asynchronous? 🤔 You're not alone! Many developers have had this question while working on their projects. In this blog post, we'll explore the behavior of Array.forEach
and whether or not it is asynchronous. 🚀
Understanding the Native Array.forEach Method
Before we dive into whether Array.forEach
is asynchronous or not, let's first understand what it does. The Array.forEach
method allows you to iterate over each element of an array and perform a specific action on each element. It takes a callback function as an argument and executes that function for each element in the array. 👍
[many many elements].forEach(function() {lots of work to do});
The Asynchronicity Dilemma
Now, let's address the burning question: is Array.forEach
asynchronous? 🤔 The answer is both yes and no! The Array.forEach
method itself is synchronous, meaning it executes each iteration one after the other. However, the code inside the callback function you provide can be asynchronous, leading to potential confusion. 😅
Callback Functions and Asynchronous Code
When the code inside your Array.forEach
callback function contains asynchronous operations, such as making API calls or performing I/O operations, the callback function itself won't wait for their completion. This can create a misconception that the Array.forEach
method is asynchronous 🌐
It's important to remember that the asynchronicity lies with the code inside the callback function, not the Array.forEach
method itself.
Handling Asynchronicity
If you need to ensure that all iterations in an Array.forEach
loop are completed before moving on to the next step in your code, you will have to handle the asynchronicity on your own.
A common approach is to use Promises
or async/await
with Array.reduce
to ensure that all asynchronous tasks inside the callback function are completed before proceeding.
const array = [many many elements];
const asyncTask = async () => {
await array.reduce(async (previousPromise, currElement) => {
await previousPromise;
await asyncWorkToDo(currElement);
}, Promise.resolve());
console.log('All asynchronous tasks completed!');
};
asyncTask();
The code snippet above demonstrates how to use async/await
and Array.reduce
to handle asynchronicity within an Array.forEach
loop. By making each iteration of the loop await the previous iteration's completion, we can ensure that all asynchronous tasks are completed before moving on to the next step in our code.
Share Your Thoughts and Experiences! 💬
Have you ever encountered confusion with the asynchronicity of Array.forEach
in JavaScript and Node.js? Share your thoughts, experiences, and any additional tips you have in the comments below! Let's learn from each other and make coding even more enjoyable. 😄 Together, we can conquer any asynchronous challenge!
So, that's it! Now you know the ins and outs of the Array.forEach
method and its potential asynchronicity. Remember, it's the code inside the callback function that can be asynchronous, not the method itself. Armed with this knowledge, go forth and conquer your coding tasks with confidence! 💪
Happy coding! 👩💻👨💻