JavaScript/jQuery to download file via POST with JSON data
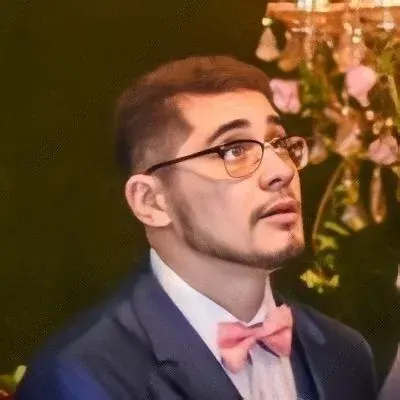
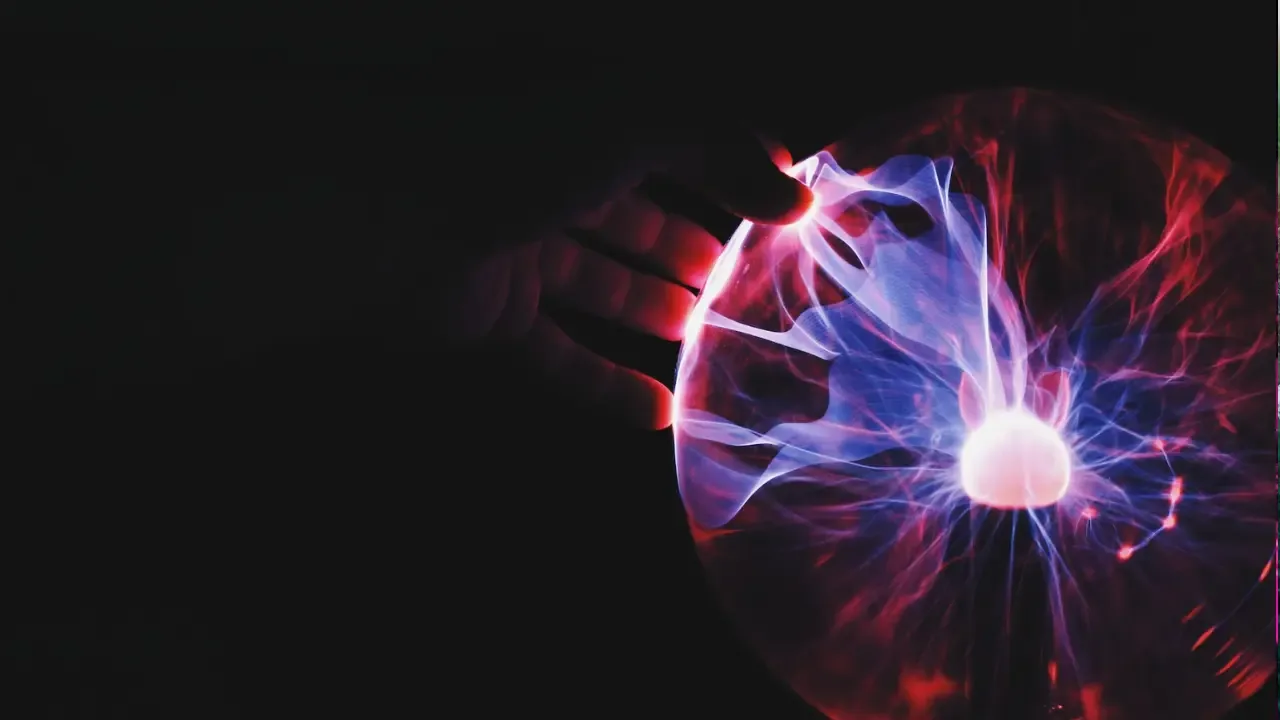
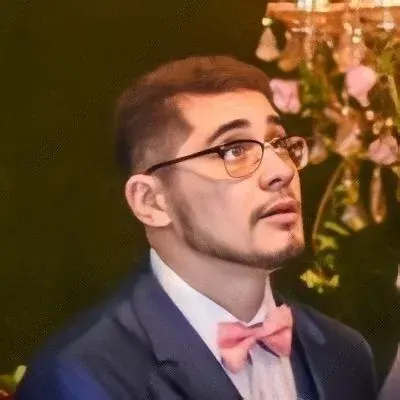
📥 Downloading Files via POST in JavaScript/jQuery
Have you ever wondered how to download a file using a POST request in JavaScript/jQuery while also sending JSON data? It can be a bit tricky, but fear not - I am here to guide you through the process!
The Challenge: Downloading Files with JSON Data
Imagine having a single-page web app that communicates with a RESTful web service through AJAX calls. You have successfully achieved steps 1 and 2: submitting a POST request with JSON data and receiving a JSON response. Now, you face the challenge of step 3: downloading a binary file (e.g., PDF, XLS) based on the specified response type.
The Solution: Server-Side Approach
One possible solution is to generate the file on the server and return a JSON response that includes a URL to the file. Then, you can use JavaScript to create a new AJAX call in the success handler and redirect the user to the generated file's URL. Here's an example of how it could be done:
$.ajax({
type: "POST",
url: "/services/test",
contentType: "application/json",
data: JSON.stringify({ category: 42, sort: 3, type: "pdf" }),
dataType: "json",
success: function(json, status) {
window.location.href = json.url; // Redirect to the generated file's URL
},
error: function(result, status, err) {
console.log("Error loading data");
}
});
This approach allows you to get additional information about the request before starting the download, such as the time to generate, file size, and error messages. However, there is a downside to this method: it requires extra server-side file management.
Alternate Approach: Direct File Download
If you'd rather avoid server-side file management and make a single AJAX call for downloading the file, here are a couple of options:
Form Submission: Instead of using AJAX, you can submit a form post and embed your JSON data into the form values. This approach may involve working with hidden iframes and additional complexities.
GET Request: Convert your JSON data into a query string and build a standard GET request. Set
window.location.href
to the generated URL to initiate the file download. You might need to useevent.preventDefault()
in your click handler to prevent the browser from navigating away from the application URL.
Both options have their pros and cons. Consider the complexity and specific requirements of your project before choosing the best approach.
Your Turn: Share Your Thoughts and Experiences!
Have you faced a similar challenge when downloading files with JSON data in JavaScript/jQuery? How did you overcome it? Are there any other creative solutions you've discovered? Share your thoughts and experiences in the comments section below!
👉🏼 Read More Tech Tips and Tricks on My Blog
Remember, sharing is caring - so hit that share button to help others who may be struggling with the same issue!