Javascript Equivalent to PHP Explode()
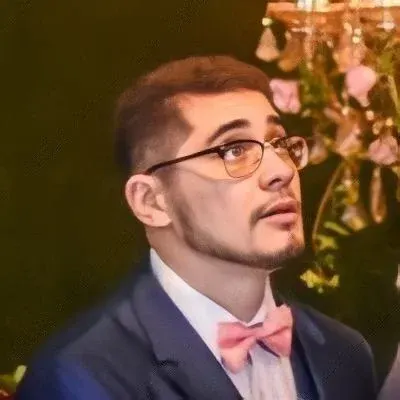
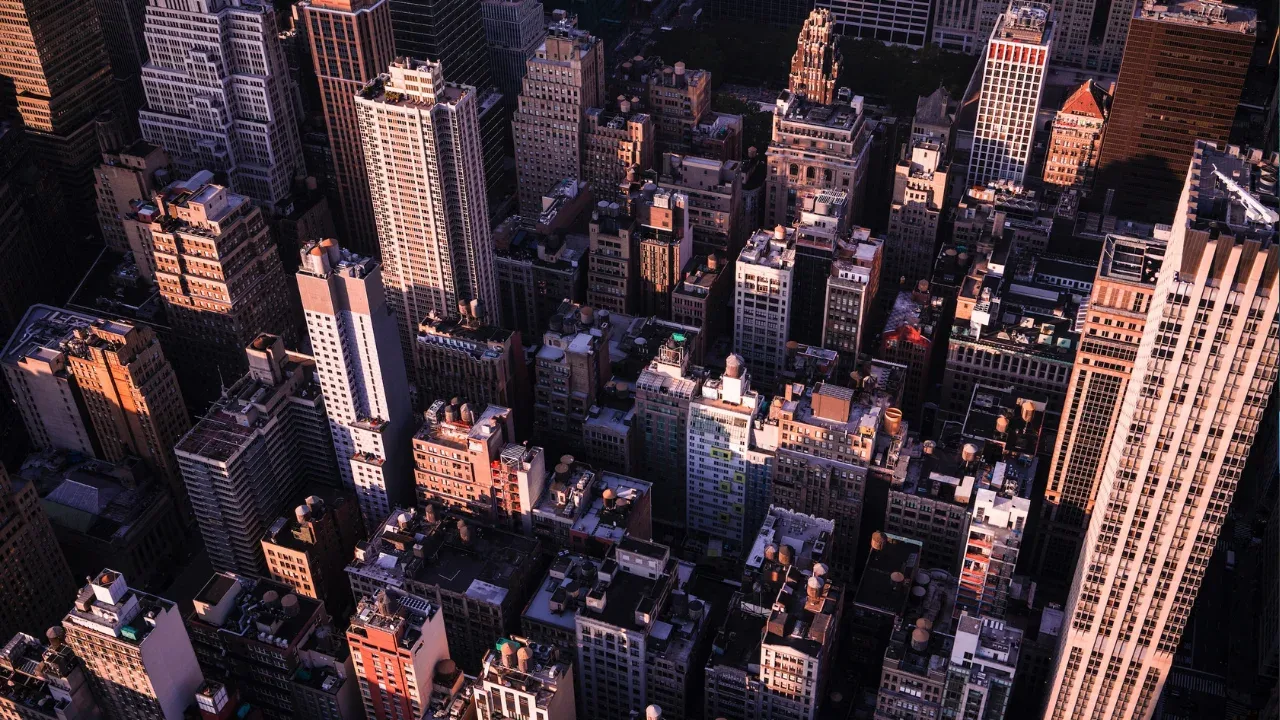
💥 JavaScript Equivalent to PHP Explode()
Are you tired of searching for a JavaScript equivalent to PHP's explode() function? Look no further! In this guide, we'll explore how to effectively split a string in JavaScript, like how it works in PHP. By the end of this article, you'll be equipped with a simple yet powerful solution. Let's dive in!
🎯 The Challenge
Imagine you have the following string:
0000000020C90037:TEMP:data
And you need to extract the substring TEMP:data
. In PHP, you can easily achieve this using the explode() function. But how can we accomplish the same in JavaScript?
🚀 The Solution
JavaScript provides us with a handy function called split(). It allows us to divide a string into an array of substrings based on a specified separator. To obtain the desired result, we can use the following code:
const str = '0000000020C90037:TEMP:data';
const arr = str.split(':');
const result = arr[1] + ':' + arr[2];
console.log(result); // Output: TEMP:data
Let's break it down:
We declare a variable
str
and assign our original string to it.Using the split() function, we divide
str
into an array of substrings based on the':'
separator.We create a new variable
result
by concatenating the second and third elements of thearr
array with':'
in between.Finally, we output
result
to the console.
Voila! You have successfully exploded the string in JavaScript!
😎 Dive Deeper
Now that you know the basic technique, you can take it a step further. Let's explore different use cases for splitting strings in JavaScript:
Splitting Comma-Separated Values
const csv = 'apple,banana,orange';
const fruits = csv.split(',');
console.log(fruits); // Output: ['apple', 'banana', 'orange']
Splitting URL Parameters
const url = 'https://www.example.com?param1=value1¶m2=value2';
const params = url.split('?')[1].split('&');
console.log(params); // Output: ['param1=value1', 'param2=value2']
Splitting Multiline Text
const text = 'Hello\nWorld\nHow\nAre\nYou';
const lines = text.split('\n');
console.log(lines); // Output: ['Hello', 'World', 'How', 'Are', 'You']
🙌 Call to Action
Exploding strings in JavaScript is no longer a mystery! With the power of the split() function, you can easily split strings and conquer complex tasks. Feel free to unleash your creativity and explore how this technique can simplify your coding life.
If you found this guide helpful, share it with your fellow developers and spread the word! Comment below and let us know how you've used the split() function in your projects. Happy coding! 👩💻👨💻
Note: Remember to test your code and handle any edge cases to ensure reliable functionality.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
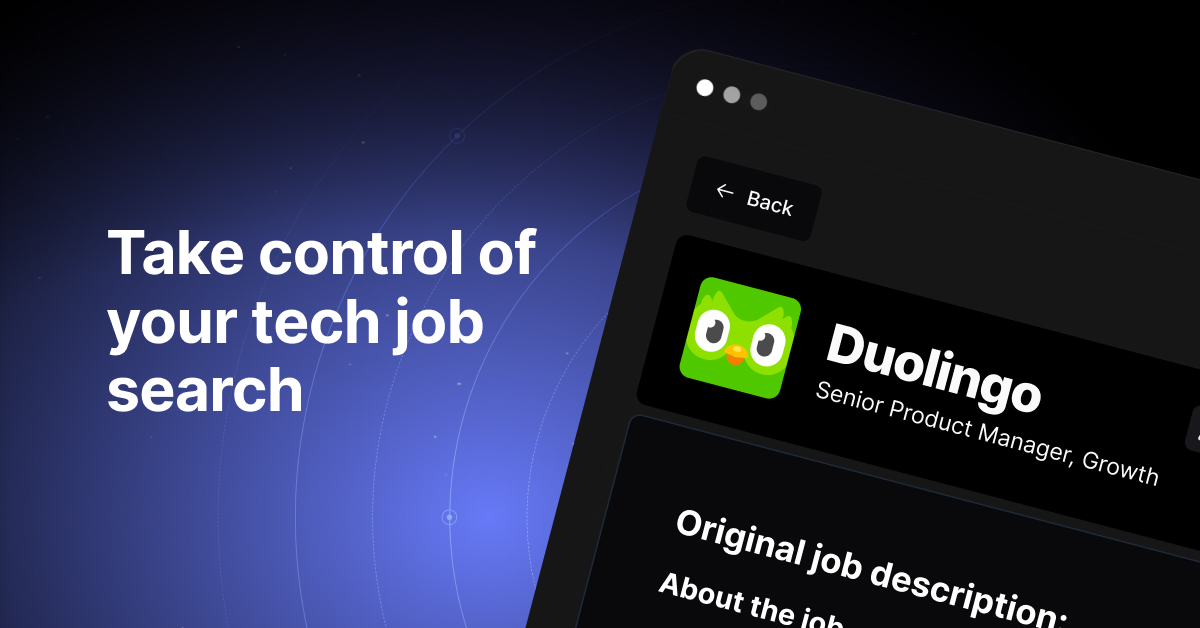