JavaScript check if variable exists (is defined/initialized)
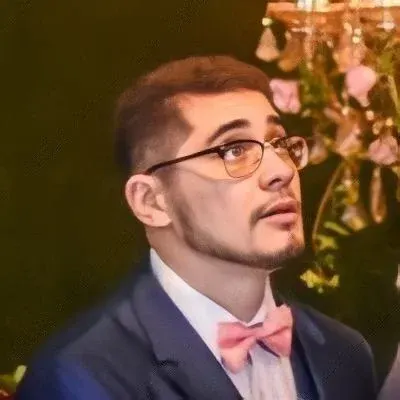
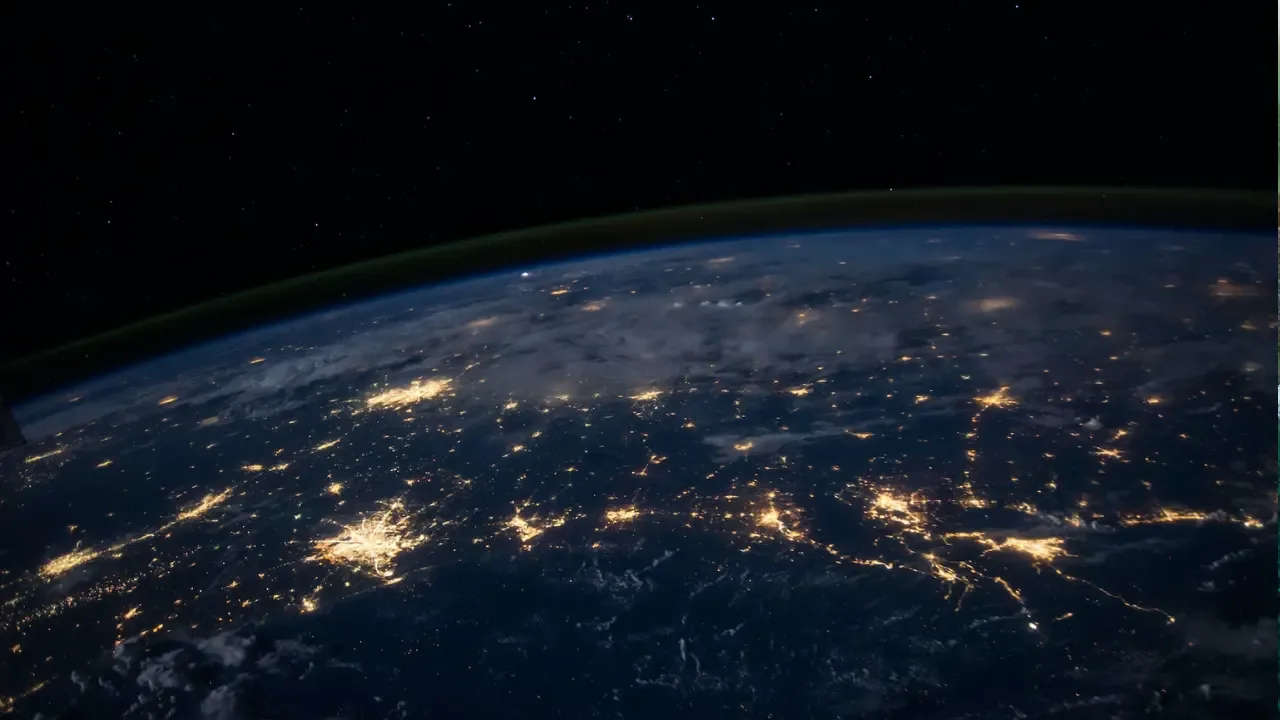
👋👋 Hey there, tech enthusiasts! Today we're diving into the world of JavaScript and tackling a common question that often pops up in our coding adventures: how can we check if a variable exists and is initialized in JavaScript? 🤔💻
Imagine this scenario: you're working on a JavaScript project, juggling variables of various types 🎪 (strings, numbers, objects, functions, you name it!). But before performing any operations on these variables, you need to ensure they exist and have been initialized. So, how do we go about this? Let's explore some popular methods! 🕵️♂️
1️⃣ Using a simple if statement:
if (elem) {
// Code to execute if elem exists and is truthy
}
This approach relies on JavaScript's truthy and falsy values. By using if (elem)
, we're checking if the variable elem
has a value that evaluates to true. If elem
is indeed initialized and holds a truthy value, the code inside the if statement will execute. However, be aware that this method does not differentiate between not being defined and holding a falsy value, which may lead to unexpected results. 🤷♀️
2️⃣ Using typeof and strict inequality:
if (typeof elem !== 'undefined') {
// Code to execute if elem is defined and initialized
}
This method employs the typeof
operator, which returns the type of a variable. Here, we're checking if the type of elem
is not equal to 'undefined'
. If that's the case, the code inside the if statement will run. By using typeof
, we specifically target the defined and initialized variables. It's a more precise way to verify if a variable exists before proceeding further. 🧐
3️⃣ Using the null comparison operator:
if (elem != null) {
// Code to execute if elem is not null or undefined
}
In this approach, we leverage the !=
(loose inequality) operator to check if elem
is neither null
nor undefined
. If this condition holds true, the code inside the if statement gets executed. However, be cautious when using !=
instead of !==
, as it performs type coercion. This means that two values with different types might still be considered equal by JavaScript. So, double-check your comparisons when opting for this method! 😶
Regardless of the method you choose, it's vital to consider the context of your code and the behavior you desire. You may want to ask yourself: do I need precise type checking? Do I only want to ensure the variable exists and is initialized, regardless of its value? 🤔
Now that we've explored these common methods, it's your turn to experiment and incorporate them into your JavaScript endeavors! Remember, understanding variable existence and initialization is fundamental to writing robust code. 😎
But wait, we're not finished just yet! We'd love to hear your thoughts on this topic. Which method do you prefer and why? Have you encountered any additional tips or tricks while exploring this JavaScript conundrum? Share your experiences and ideas in the comments below! Let's keep the conversation going! 💬📢
So, until next time, happy coding and stay curious! 🚀✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
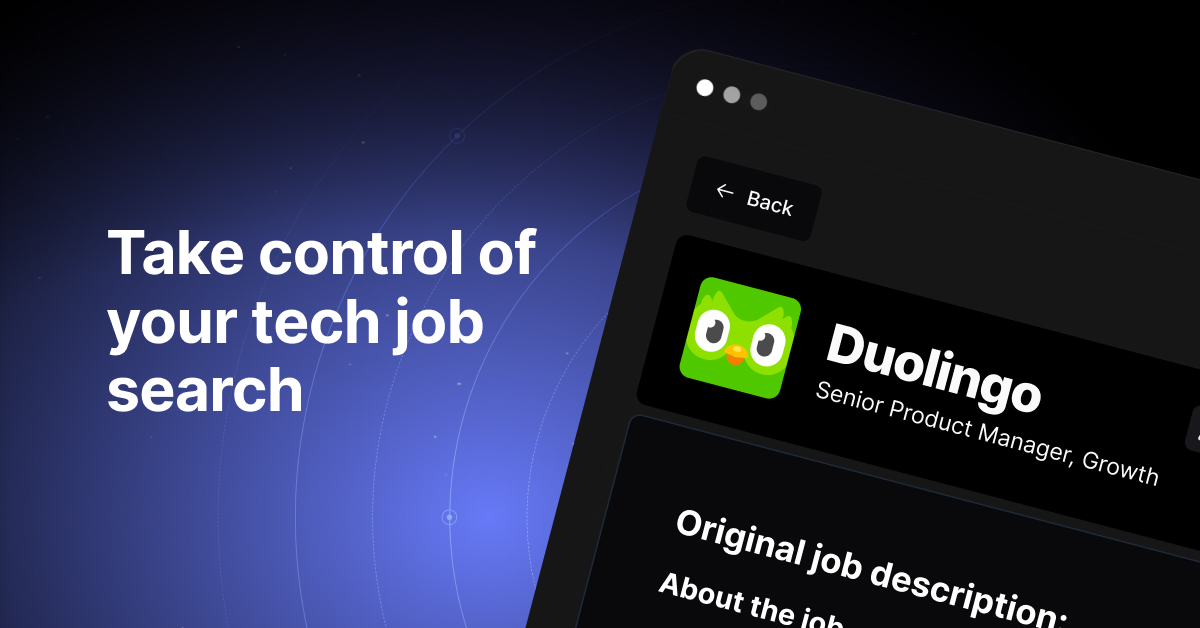