Iterate through object properties
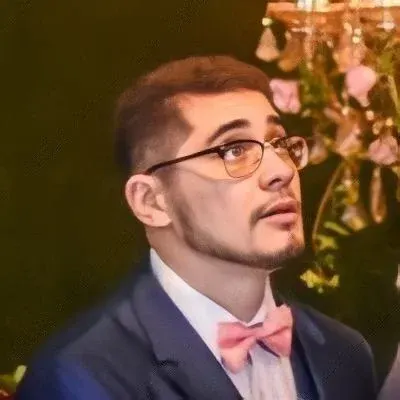
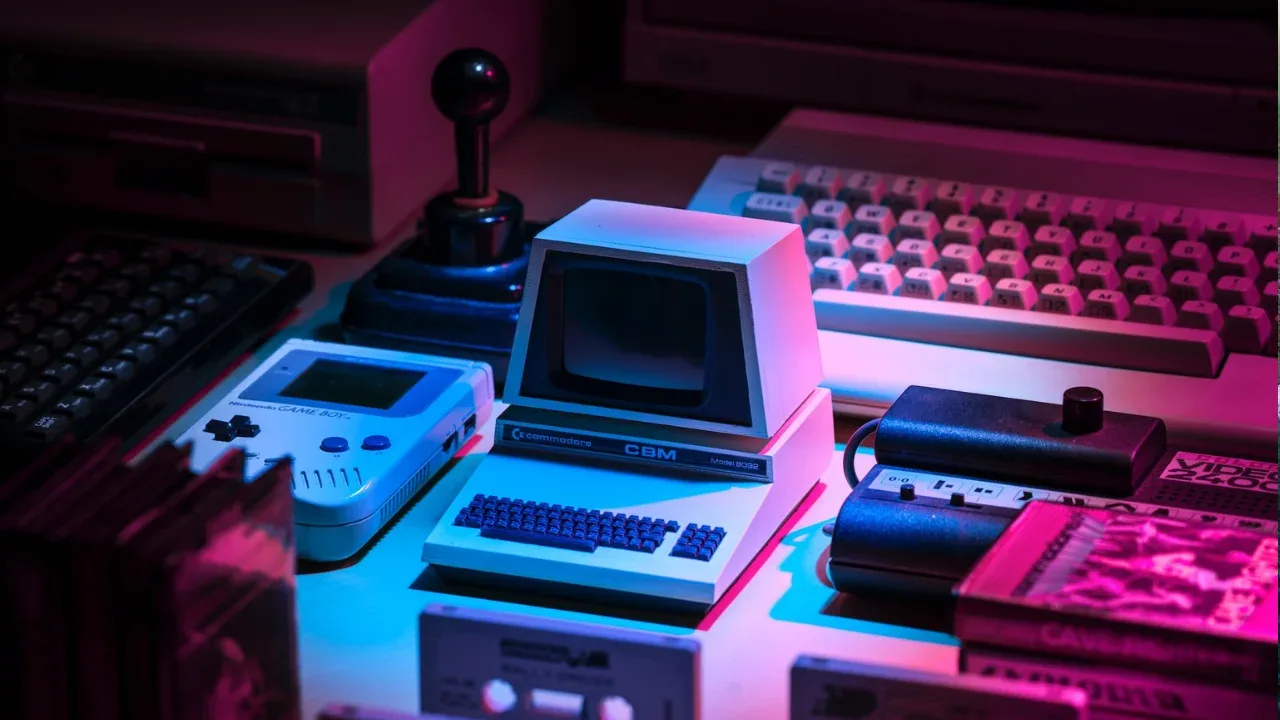
🚀 Iterating Through Object Properties: A Beginner's Guide
Are you struggling to iterate through object properties in JavaScript? 🤔 Don't worry, you're not alone! Many developers find this concept confusing at first. But fear not, because in this blog post, we'll demystify the process and provide you with easy solutions to common issues. Let's dive in! 💪
Understanding the Code
First, let's take a look at the code snippet you shared:
var obj = {
name: "Simon",
age: "20",
clothing: {
style: "simple",
hipster: false
}
}
for(var propt in obj){
console.log(propt + ': ' + obj[propt]);
}
Here, we have an object obj
that contains various properties such as name
, age
, and clothing
. The clothing
property is itself an object with its own properties. The for...in
loop is used to iterate through each property of the object and log it to the console.
Understanding the for...in
Loop
Now, let's focus on the line of code that might be causing confusion:
for(var propt in obj){
console.log(propt + ': ' + obj[propt]);
}
In this loop, the variable propt
represents each property of the object obj
as the loop iterates through them. It's important to note that propt
is not a built-in method or property – it's simply a variable name chosen by the developer.
During each iteration of the loop, propt
takes on the value of the current property name. For example, in the first iteration, propt
would be "name"
, in the second iteration, it would be "age"
, and so on.
By using propt
as the key to access the corresponding property value in obj
(e.g., obj[propt]
), we can log both the property name and its value to the console.
Easy Solutions
If you're looking for an alternative way to iterate through object properties, you can use the Object.keys()
method:
Object.keys(obj).forEach(function(propt) {
console.log(propt + ': ' + obj[propt]);
});
Here, Object.keys(obj)
returns an array of property names from the obj
object. We then use the forEach()
method to iterate through each property name and perform the desired actions.
The Wrap-up
Now that you understand how to iterate through object properties, you can confidently tackle similar challenges in your JavaScript projects. To summarize:
The
for...in
loop enables you to iterate through object properties.The variable name (
propt
in our example) represents the current property name during each iteration.You can also use the
Object.keys()
method to achieve the same result.
Feel free to experiment with these techniques and adapt them to your specific needs. Remember, practice makes perfect! 💪
Share Your Thoughts!
We hope this guide has been helpful in clarifying the process of iterating through object properties. If you have any questions, suggestions, or additional tips, please feel free to share them in the comments below. Let's learn and grow together! 🌱
Don't forget to share this blog post with your fellow developers who might need a little help with object property iteration! Sharing is caring. 😄💙
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
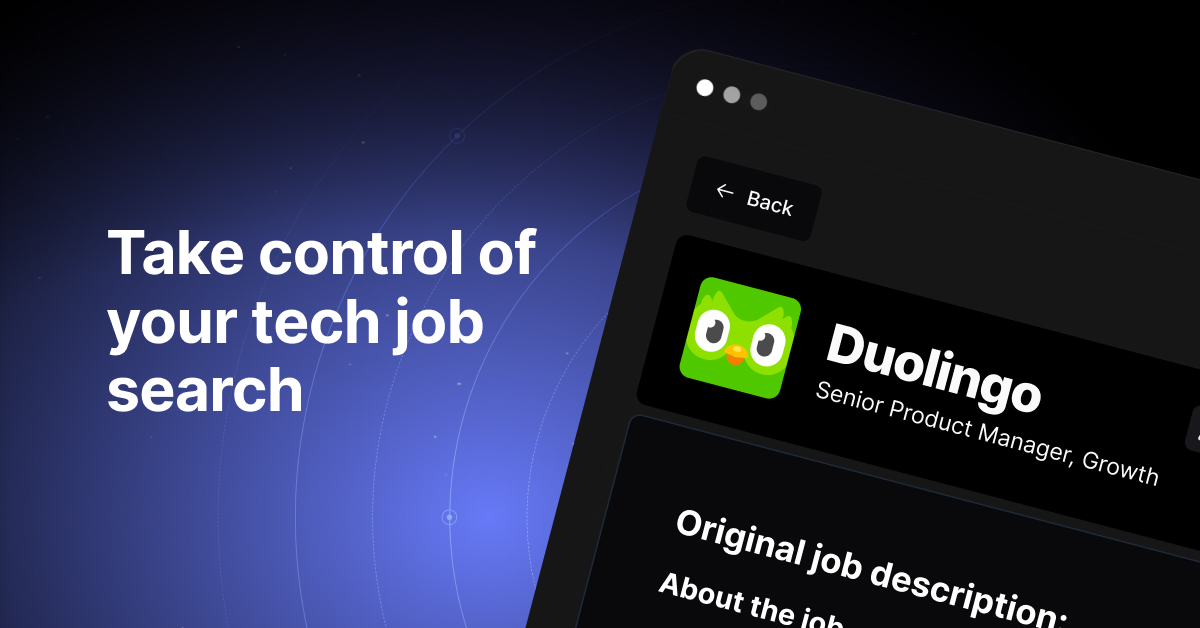