Is there an "exists" function for jQuery?
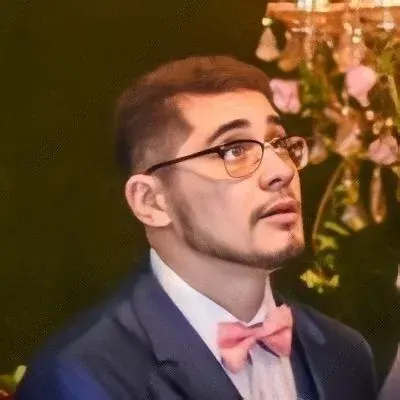
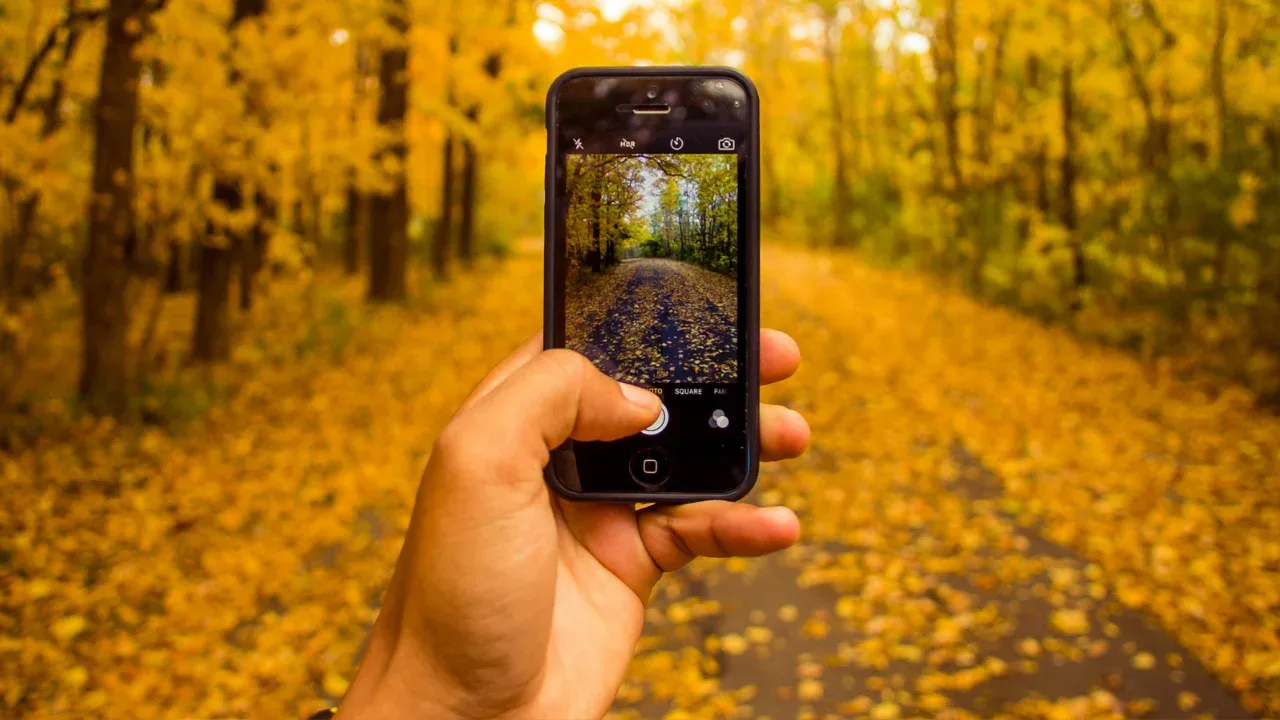
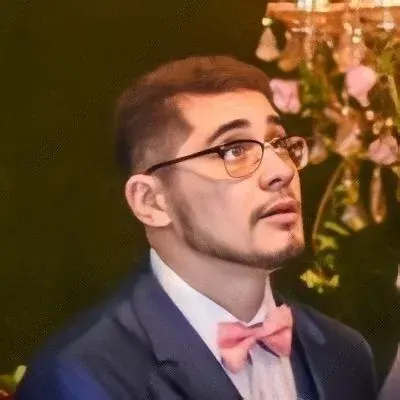
🤔 Is there an "exists" function for jQuery?
As a JavaScript developer, you've most likely encountered a situation where you need to check if an element exists before performing certain actions. Luckily, jQuery offers a simple and elegant solution to this common problem.
So, let's answer the question: Is there an "exists" function for jQuery? 🤔
💡 The Current Code
Before we dive into other solutions, let's take a look at the current code you might be using to check the existence of an element in jQuery:
if ($(selector).length > 0) {
// Do something
}
This code checks the length property of the jQuery object returned by $(selector)
. If the length is greater than zero, it means that the element exists, and you can proceed with your desired actions.
🚀 A More Elegant Approach
While the current code gets the job done, jQuery provides a more elegant and concise approach for checking if an element exists. It involves using the .length
property in a boolean context.
Here's how it works:
if ($(selector).length) {
// Do something
}
In this updated code, instead of explicitly comparing the length to zero, we rely on the fact that a non-zero length is "truthy" in JavaScript. So, if the length is greater than zero, the condition evaluates to true, and you can proceed with your code execution.
🌟 The "exists" Plugin
On top of the elegant solution provided by jQuery, there is also a dedicated plugin called "exists." This plugin extends the jQuery object with a handy exists()
function, which provides a more readable syntax for checking element existence.
To use the "exists" plugin, you first need to include it in your HTML:
<script src="jquery.exists.js"></script>
Then, you can use the exists()
function like this:
if ($(selector).exists()) {
// Do something
}
The result is the same as using the previous approaches, but the code becomes more expressive and self-explanatory.
📣 Call-to-Action: Enhance Your jQuery Skills
Now that you've learned about the different ways to check for element existence in jQuery, it's time to put your knowledge into practice! Experiment with both the concise syntax and the "exists" plugin in your projects.
If you're hungry for more jQuery tips and tricks, head over to my blog and discover additional techniques to supercharge your JavaScript development. Don't forget to bookmark the page and join the discussion in the comments section!
Remember, mastering jQuery opens up a world of possibilities and allows you to create amazing interactive experiences on the web.
Happy coding! 🎉