Is there a version of JavaScript"s String.indexOf() that allows for regular expressions?
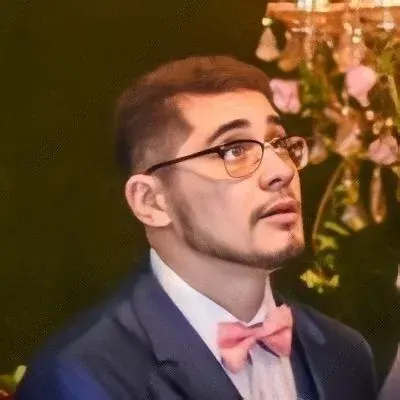
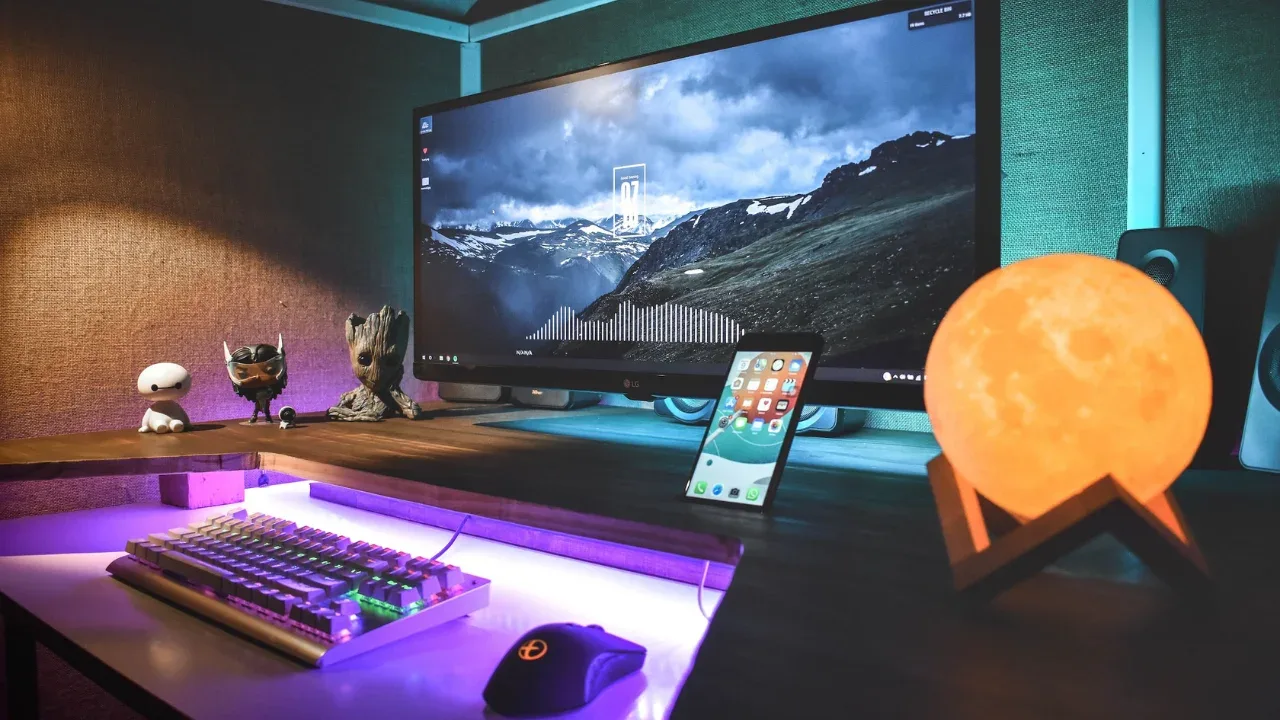
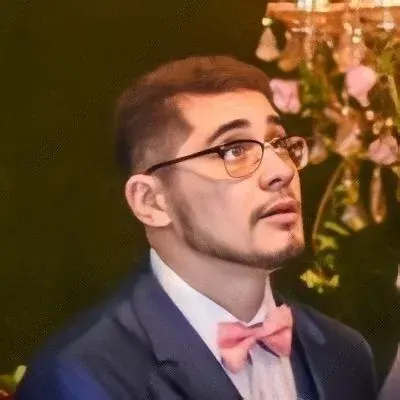
🔎 Finding a Regular Expression-Friendly String.indexOf()
Have you ever wondered if there's a way to use regular expressions with JavaScript's String.indexOf() function? 🤔 It's a common question, and fortunately, there are solutions available. In this blog post, we'll explore the problem, provide easy solutions, and put them to the test. So let's dive in! 💪
The Problem: String.indexOf() and Regular Expressions
JavaScript's String.indexOf() is a commonly used method to search for the position of a substring within a string. However, it only works with plain strings and doesn't accept regular expressions as the search term.
For example, let's say you want to search for the first occurrence of the characters 'a', 'b', or 'c' in a string. Using the conventional String.indexOf(), you might try:
str.indexOf(/[abc]/);
But this won't work because String.indexOf() expects a string, not a regular expression. 😕
The Solution: regexIndexOf()
To overcome this limitation, we can create a custom function that does accept regular expressions. Let's call it regexIndexOf()
.
Here's how you can implement regexIndexOf()
:
String.prototype.regexIndexOf = function (regex, startFrom) {
const substring = this.substring(startFrom);
const match = substring.match(regex);
return match ? match.index + startFrom : -1;
};
With this function, you can now search for the first occurrence of a regular expression pattern in a string:
const index = str.regexIndexOf(/[abc]/);
Putting the Solution to the Test
To ensure our solution works as intended, let's run a few tests. The provided code in the question includes a test()
function that compares the output of regexIndexOf()
with the conventional indexOf()
.
function test(str) {
var i = str.length + 2;
while (i--) {
if (str.indexOf('a', i) !== str.regexIndexOf(/a/, i)) {
alert(['failed regexIndexOf ', str, i, str.indexOf('a', i), str.regexIndexOf(/a/, i)]);
}
if (str.lastIndexOf('a', i) !== str.regexLastIndexOf(/a/, i)) {
alert(['failed regexLastIndexOf ', str, i, str.lastIndexOf('a', i), str.regexLastIndexOf(/a/, i)]);
}
}
}
// Testing the solution
test('xxx');
test('axx');
test('xax');
test('xxa');
test('axa');
test('xaa');
test('aax');
test('aaa');
Run the code above, and if there are no failures reported, congratulations! 🎉 Your regexIndexOf()
function is working correctly.
Time to Level Up Your String Searching!
Now that you have a regex-friendly version of String.indexOf(), you can level up your string searching game! 🚀
In your own code, simply use regexIndexOf()
whenever you need to find the position of a regular expression pattern in a string. Remember to add the regexIndexOf()
function to the String prototype to make it accessible across all your strings.
💡 Pro Tip: You can also create a regexLastIndexOf()
function using the same approach to find the last occurrence of a regular expression pattern in a string.
So go ahead and empower your JavaScript code with the ease and flexibility of regular expressions in your string searching adventures!
If you found this blog post helpful, share it with your fellow developers to make their lives easier too. Have any questions or ideas? Drop a comment below and let's discuss! 😃