Is there a standard function to check for null, undefined, or blank variables in JavaScript?
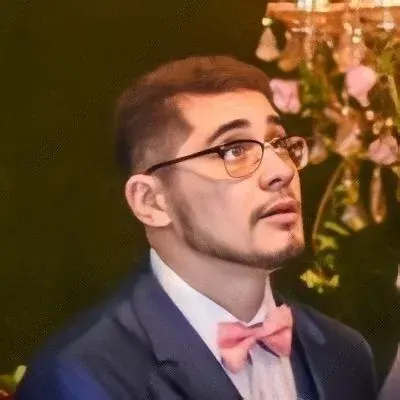
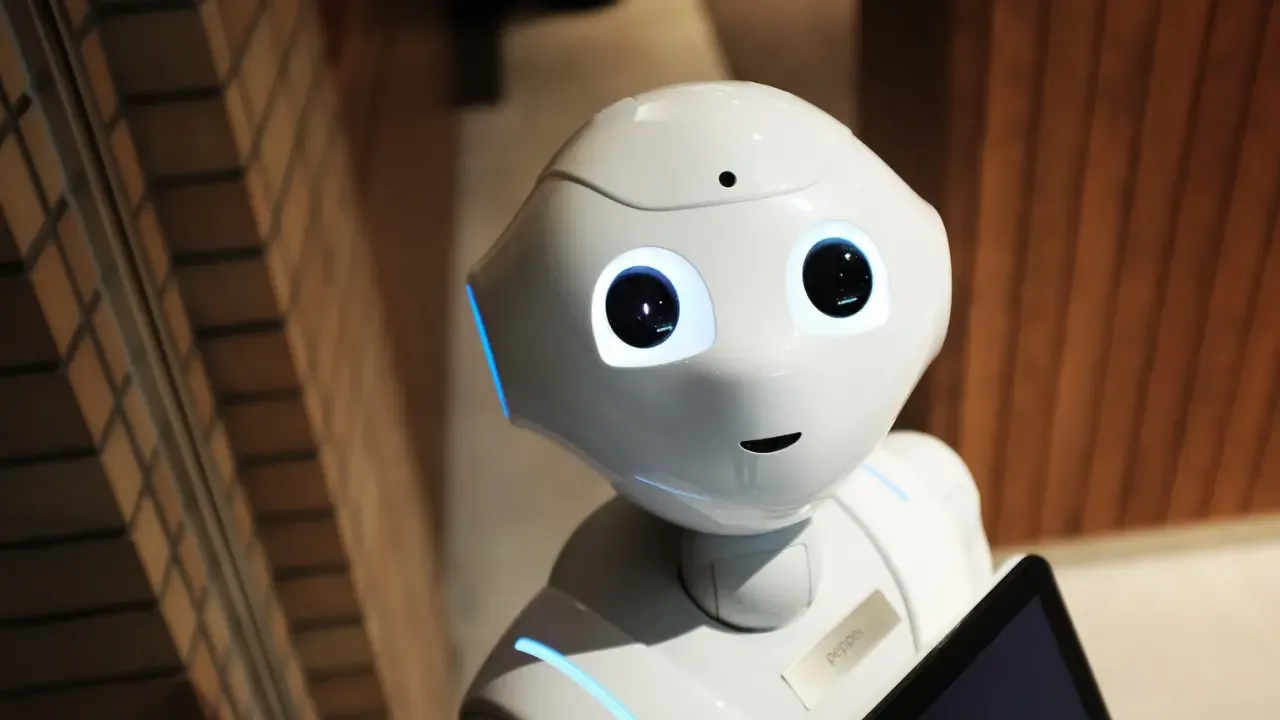
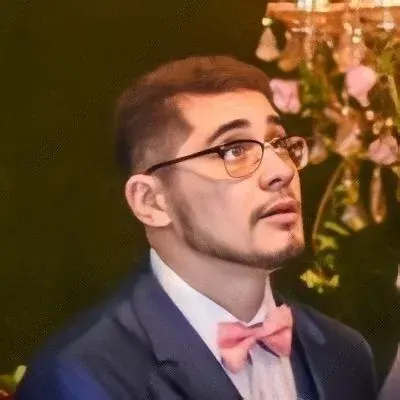
Is there a standard function to check for null, undefined, or blank variables in JavaScript? ๐ค
So, you're wondering if there is a universal JavaScript function that can quickly check if a variable has a value and ensure that it's not undefined
, null
, or blank? You want to make sure your code covers all the cases. Don't worry, I've got you covered! ๐
The Common Issue ๐
One common issue many JavaScript developers face is how to efficiently check if a variable is null, undefined, or empty. It's always a good practice to handle these scenarios gracefully to avoid potential errors and bugs in your code.
The Simple Solution ๐ก
While there isn't a standard function in JavaScript that can do it all for you, you can certainly create a reusable function to check for null, undefined, and blank values. Let's modify the code you shared to make it more concise and effective:
function isEmpty(val) {
return !(val && val.trim().length);
}
How it Works ๐ ๏ธ
In our isEmpty
function, we're making use of a few built-in JavaScript methods:
trim()
- This method removes any leading and trailing white spaces from a string. It's useful to eliminate any extra empty spaces and consider the string as truly empty.length
- By using this property, we can check if a string is empty (length is zero) or not.Logical NOT operator
!
- By negating the final result, we returntrue
if the string is empty, andfalse
otherwise.
With these modifications, our function becomes more powerful and efficient in handling null, undefined, and blank values.
Let's Test it with Some Examples ๐งช
To check if a variable
name
has a value:
const name = 'John';
console.log(isEmpty(name)); // Output: false
To check if a variable
address
is null, undefined, or blank:
const address = null;
console.log(isEmpty(address)); // Output: true
To check if a variable
email
is empty:
const email = ' ';
console.log(isEmpty(email)); // Output: true
Your Turn! โ๏ธ
Now that you know how to create a function to check null, undefined, and blank values in JavaScript, go ahead and implement it in your code. It will ensure your variables are properly handled and save you from unexpected errors.
Remember, clean and error-free code not only impresses your colleagues but also improves future code maintenance.
If you have any questions, suggestions, or alternative solutions, I would love to hear them! Let's continue this discussion in the comments below. Happy coding! ๐ป๐