Is there a RegExp.escape function in JavaScript?
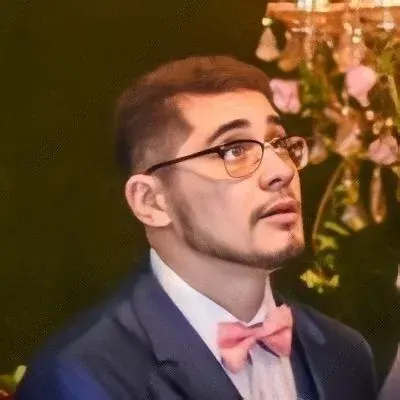
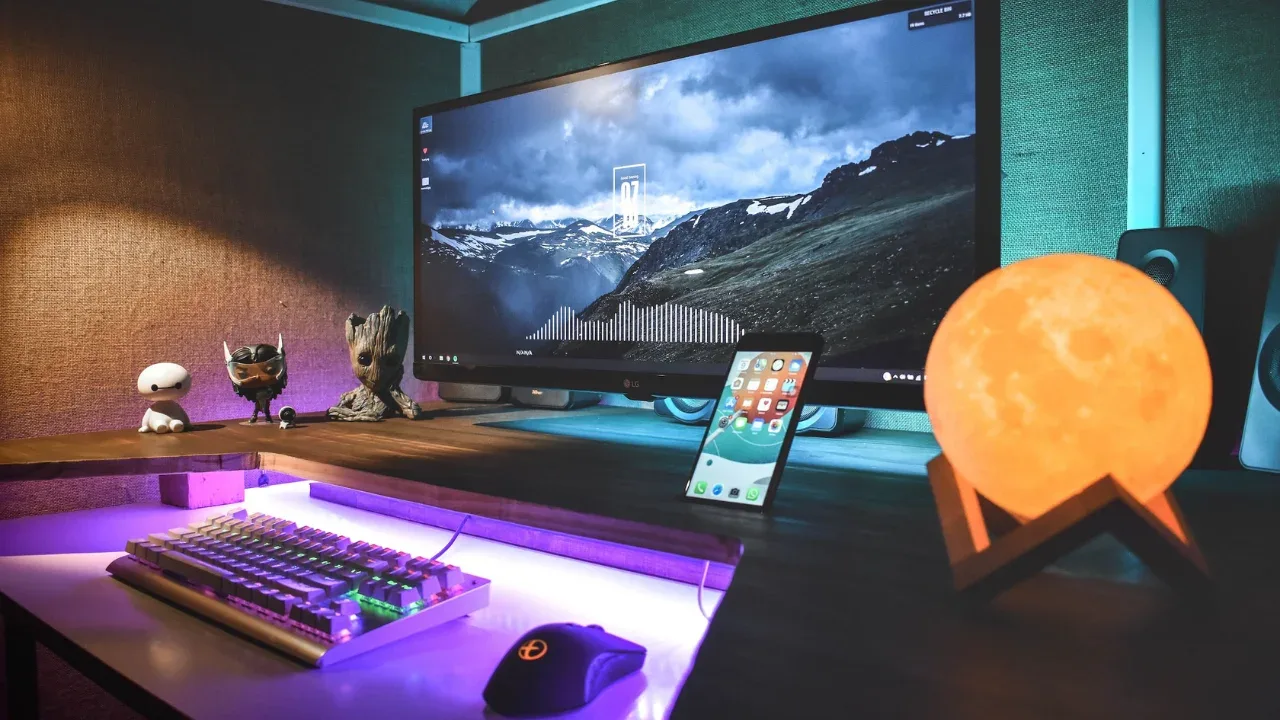
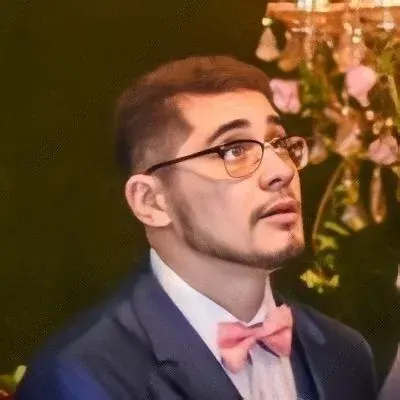
📜 The Quest for RegExp.escape in JavaScript 🧩
Have you ever found yourself embarking on a quest to escape special characters in a string before creating a regular expression out of it in JavaScript? Fear not, for I am here to guide you on this epic journey! 🏰💪
The Problem 🤔
As our fellow adventurer mentioned, they were looking for a RegExp.escape
function in JavaScript. However, unfortunately, JavaScript itself does not provide a built-in RegExp.escape
method 🙅♂️. This means we cannot directly escape special characters in a string to use as a valid regular expression pattern.
The Solution 🎯
But do not despair, dear traveler! Although there isn't a built-in method, we can still overcome this obstacle using a simple workaround. We can create our own escapeRegExp
function to escape those pesky special characters. Here's an example of how you can do it:
function escapeRegExp(string) {
return string.replace(/[.*+?^${}()|[\]\\]/g, '\\$&');
}
var usersString = "Hello?!*`~World()[]";
var expression = new RegExp(escapeRegExp(usersString));
var matches = "Hello".match(expression);
In the escapeRegExp
function, we use the .replace()
method along with a regular expression pattern to match the special characters we want to escape. By replacing them with their escaped counterparts using \\$&
, we ensure that the resulting string can be used as a valid regular expression. 🎉
A Glimpse into Other Realms ✨
While JavaScript lacks a native RegExp.escape
method, other programming languages have already implemented similar functionality. For instance, Ruby, as our fellow traveler mentioned, has the RegExp.escape
method. But in the JavaScript realm, we embrace creativity and build our own path! 💪
Your Journey Begins Here! ⭐️
Now that you're equipped with the knowledge of escaping special characters in JavaScript, it's time for you to embark on your own coding quests! 🚀
If you've encountered this challenge before and have your own unique solutions, we'd love to hear from you in the comments section below! Share your experiences and help fellow adventurers on their journey. Let's build a community of problem solvers! 🌟💬
Safe travels and happy coding! 🧙♀️💻