Is there a "null coalescing" operator in JavaScript?
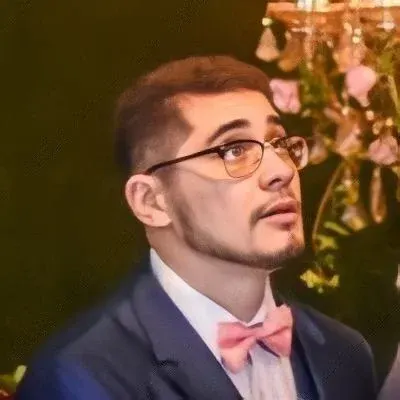
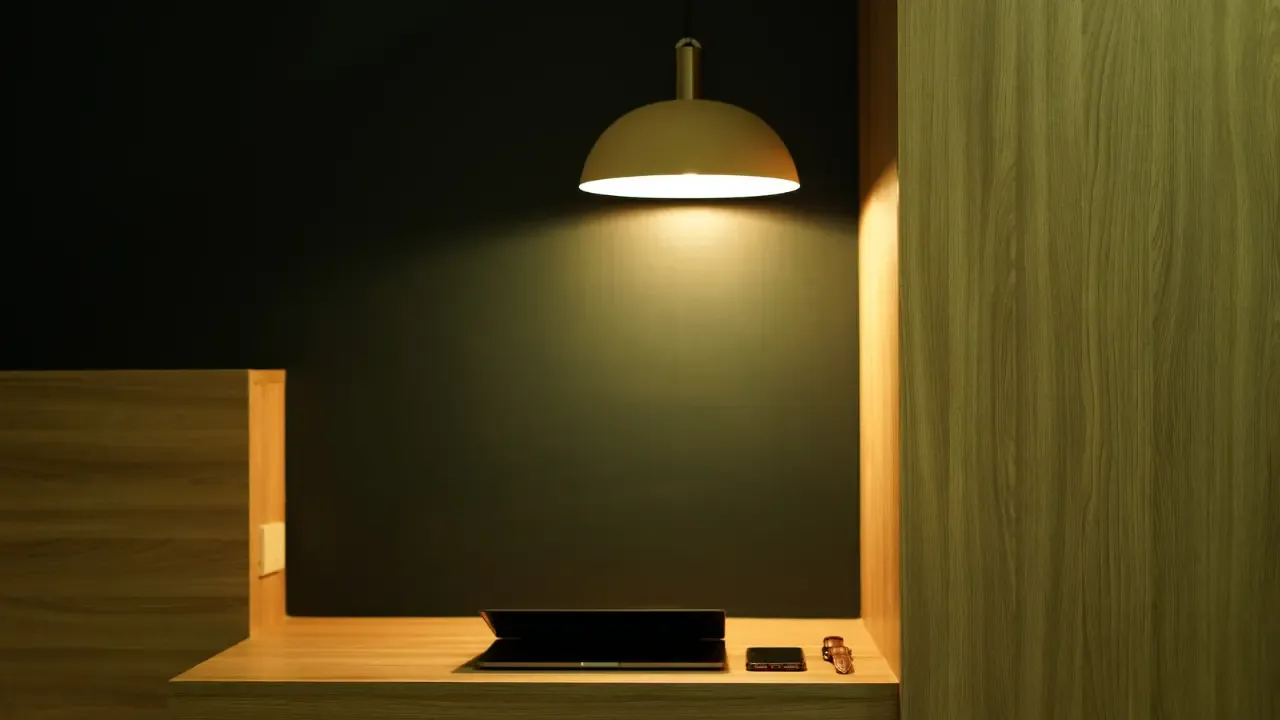
💡 Welcome to my tech blog! Today, we are exploring a much-debated question in the JavaScript community: Is there a "null coalescing" operator in JavaScript? 🤔
Understanding the Problem
Firstly, let's clarify what a null coalescing operator does. In simpler terms, it provides a more elegant way to assign a default value to a variable if it is null or undefined. 🎯
✨ The C# code snippet demonstrates the null coalescing operator in action, where the variable whatIWant
is assigned the value "Cookies!" if someString
is null or undefined. ✨
💡 In JavaScript, we don't have a direct null coalescing operator. However, we can achieve similar functionality using the conditional (ternary) operator. 🤓
JavaScript Alternative Solution To solve the problem using JavaScript, we can rewrite the code snippet like this:
var someString = null;
var whatIWant = someString ? someString : 'Cookies!';
🔍 The conditional operator ?
allows us to check if someString
has a truthy value. If it doesn't, we assign the default value 'Cookies!'
. Although this approach works, many developers find it cumbersome and less readable. 😫
Is There a Better Way? Fortunately, the JavaScript community always finds innovative solutions! 😎 Although there is no dedicated null coalescing operator, we can leverage the concept of short-circuit evaluation to achieve a more concise and readable form. 🌟
We can use the logical OR operator (||
) to provide a fallback value in case the variable is null or undefined. Here's the improved code:
var someString = null;
var whatIWant = someString || 'Cookies!';
🎉 Wow, that's much cleaner, right? The ||
operator checks if someString
has a truthy value. If it doesn't, it will be assigned the default value 'Cookies!'
. This approach is widely accepted and appreciated by JavaScript developers. 🚀
💡 Remember, the logical OR operator returns the first truthy value it encounters, so be cautious when working with boolean values.
Conclusion
In conclusion, while JavaScript doesn't have a dedicated null coalescing operator, we can use the conditional operator ?
or the logical OR operator ||
as alternatives. 😄
🔥 JavaScript has many creative solutions to common problems, allowing developers to write clean and concise code. So, go ahead and start implementing null coalescing-like behavior in your JavaScript projects! 💪
I hope this guide has helped you understand the options available to achieve null coalescing in JavaScript. If you have any questions or alternative suggestions, please leave a comment below. Let's keep pushing the boundaries of JavaScript together! 🌟
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
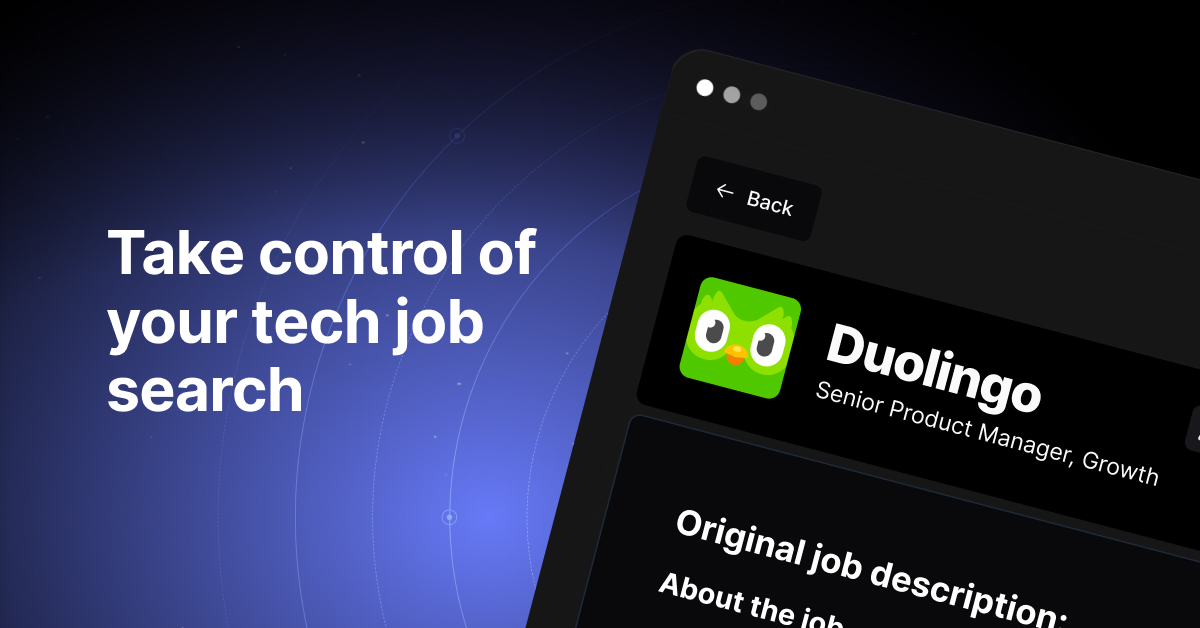