Is JavaScript a pass-by-reference or pass-by-value language?
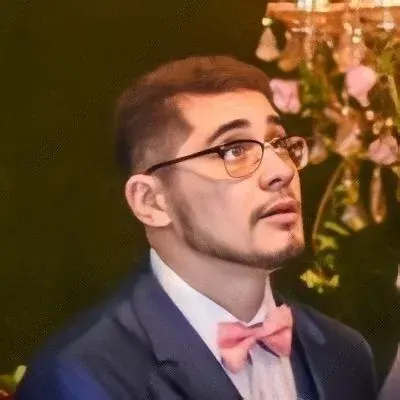
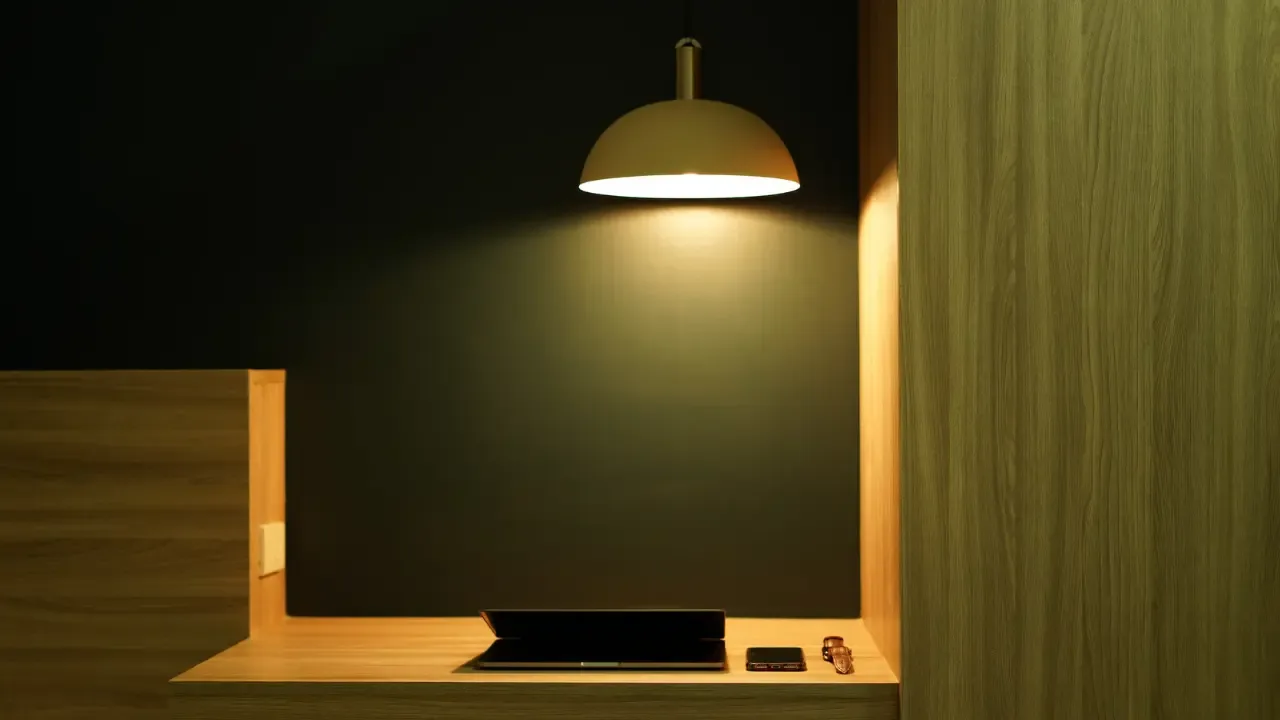
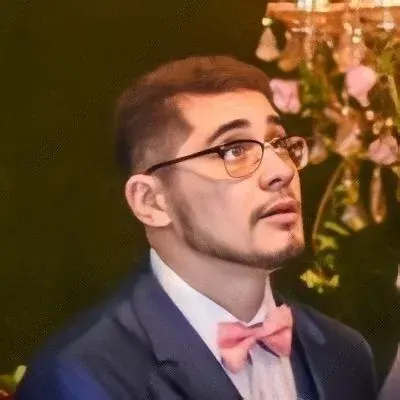
💡 Is JavaScript a pass-by-reference or pass-by-value language?
If you've ever dived into JavaScript, you might have encountered some confusion about whether it is a pass-by-reference or pass-by-value language. 🤔
So, let's clear the air and answer this burning question once and for all! 🧐
Understanding Pass-by-Value and Pass-by-Reference
Before we delve into JavaScript's behavior, let's quickly recap what pass-by-value and pass-by-reference mean.
Pass-by-value: When a value is passed to a function, a copy is made and passed to the function. Any modifications made inside the function do not affect the original value.
Pass-by-reference: When a reference (memory address) is passed to a function, any modifications made inside the function affect the original value.
JavaScript's Approach: A Mix of Both 🤷♀️
In JavaScript, the answer to whether it's pass-by-reference or pass-by-value isn't as straightforward as in some other languages. It's both – to some extent!
Primitive Types: JavaScript treats primitive types, such as numbers and strings, as pass-by-value. This means that when you pass a primitive value to a function, a copy of that value is created and passed to the function. Changes inside the function do not affect the original value.
Objects: Objects in JavaScript behave a bit differently. When you pass an object to a function, it is actually passed by copy of a reference. 🤯 This means that the variable holding the object is essentially a reference to the object itself. Any changes made to the object's properties inside the function will affect the original object.
An Example to Illustrate
Let's see an example to make things crystal clear:
function updateName(person) {
person.name = "John Doe";
}
const personObj = { name: "Jane Doe" };
updateName(personObj);
console.log(personObj.name); // Output: "John Doe"
In the above code, the personObj
is passed to the updateName
function. Since the object is passed by a copy of the reference, any modifications made to the person
parameter inside the function affects the original object.
Conclusion: Pass-by-Value or Pass-by-Reference?
While JavaScript primarily follows the pass-by-value convention for primitive types, its behavior with objects can be misleading. Technically, JavaScript is pass-by-value, but objects are passed by a copy of a reference, giving the illusion of pass-by-reference.
Understanding this distinction is crucial when working with JavaScript. It helps prevent unexpected bugs and makes your code more predictable.
So, next time someone asks you if JavaScript is pass-by-reference or pass-by-value, you can confidently answer, "Both, but objects are passed by a copy of a reference!" 🙌
🔥 Take Action: If you found this post helpful, share it with your fellow developers who might be struggling with the pass-by-value/pass-by-reference concept in JavaScript. Let's spread the knowledge! 💪
Have you ever encountered any tricky situations related to JavaScript's pass-by-value/pass-by-reference behavior? Share your experiences in the comments below! 🗣️