Is it not possible to stringify an Error using JSON.stringify?
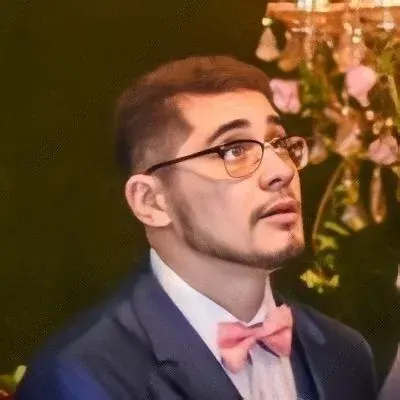
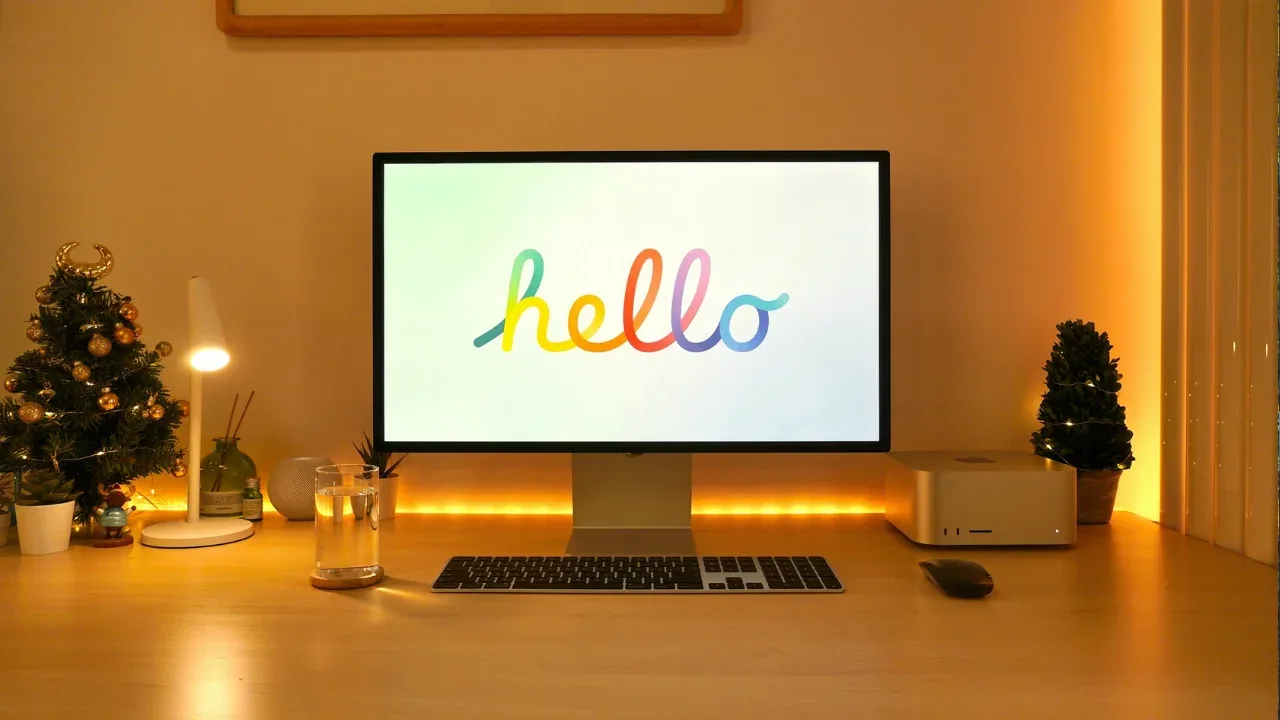
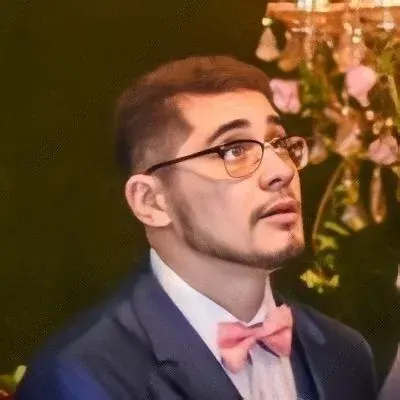
Can I stringify an Error using JSON.stringify? 🤔
If you've ever tried to pass error messages around using web sockets or had the need to stringify an Error object, you might have run into an issue where JSON.stringify
returned an empty object. This can be frustrating and make it difficult to handle errors efficiently.
Let's take a look at how to reproduce the problem and explore some possible solutions.
Reproducing the Problem 🐞
To begin, let's consider a simple example where we try to stringify an Error object using JSON.stringify
. Here's the code:
let error = new Error('simple error message');
console.log(JSON.stringify(error)); // {}
As you can see, the result is an empty object. This behavior can be unexpected and confusing.
However, you might notice that the output of error
when logged to the console is [Error: simple error message]
. This discrepancy raises the question: Why does JSON.stringify
not include the error message?
Understanding the Issue 🤔
When we dig deeper into the behavior of JSON.stringify
with Error objects, we find that the Error.prototype
does not enumerate its properties. This means that the native properties of an Error object, such as message
and stack
, are non-enumerable. Consequently, JSON.stringify
ignores these properties.
Workarounds and Solutions 💡
Stick with simple string-based error messages or create your own custom error objects. Sometimes, using plain strings or custom error objects can be a more straightforward and effective approach.
Pull specific properties manually when stringifying an error object. By selectively choosing the properties you want to include, you can ensure that relevant information is preserved. For example:
JSON.stringify({ message: error.message, stack: error.stack })
With this technique, you can have a more controlled and meaningful representation of your error.
Conclusion 🎉
While it is not possible to stringify an Error object using JSON.stringify
directly, there are alternative approaches that can help you handle error messages effectively. By understanding the nature of the issue and exploring workarounds, you can work with errors in a more structured and manageable way.
Remember, the key is to find the approach that aligns best with your specific use case and requirements.
Have you encountered similar challenges with stringifying Errors? Share your experiences and insights in the comments below. Let's help each other overcome these obstacles! 💪