Invariant Violation: Objects are not valid as a React child
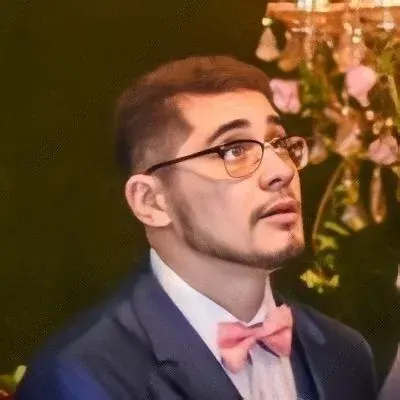
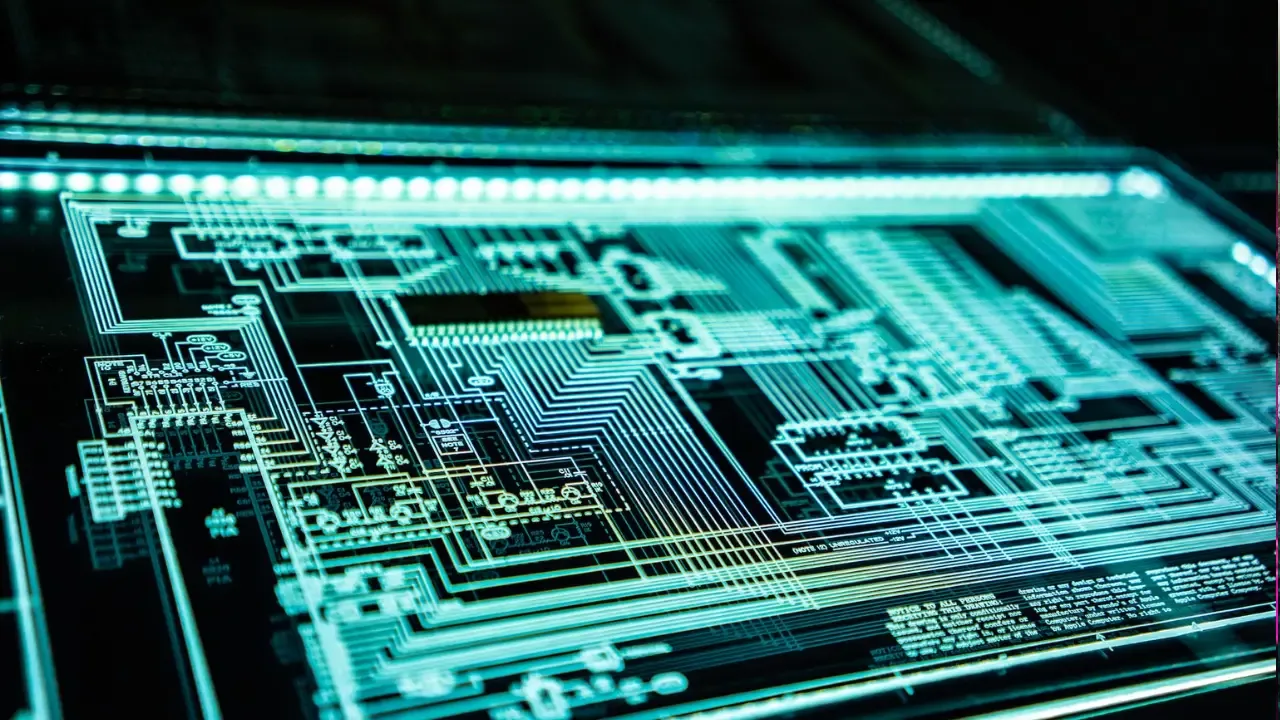
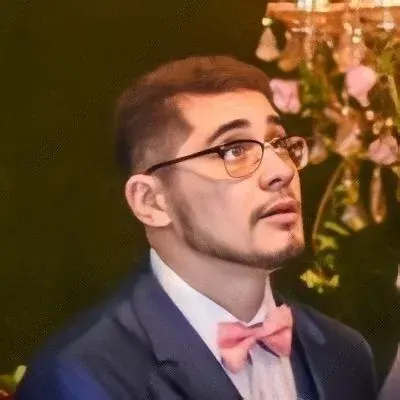
🚨 Error: Invariant Violation: Objects are not valid as a React child 🚨
Have you ever encountered the error "Invariant Violation: Objects are not valid as a React child" while working with React? Don't worry, you're not alone! This error can be quite confusing, but fear not, because I'm here to help you understand and solve it! 😎
🤔 What's the problem?
Let's take a look at the code snippet that triggered the error:
render() {
const items = ['EN', 'IT', 'FR', 'GR', 'RU'].map((item) => {
return (<li onClick={this.onItemClick.bind(this, item)} key={item}>{item}</li>);
});
return (
<div>
...
<ul>
{items}
</ul>
...
</div>
);
}
In this example, we have a render
function within a React component. The render
function is responsible for generating the UI for our component. Inside the render
function, we create a list of <li>
elements using the map
method on an array of languages.
However, when we click on one of the <li>
elements, an error is thrown: "Invariant Violation: Objects are not valid as a React child."
🙄 Why did we get this error?
The error occurs because the onClick
event handler function, this.onItemClick.bind(this, item)
, is being called with an unexpected object as its first argument.
To understand why this is happening, let's take a closer look at the bind
method.
The bind
method creates a new function that, when called, has its this
keyword set to the provided value (this
) and appends any additional arguments to the new function's arguments list.
In our case, this.onItemClick.bind(this, item)
creates a new function that is bound to this
(the component instance) and has item
as an additional argument. However, when the onClick
event is triggered, React passes an event object as the first argument to the event handler function.
Hence, the error occurs because we're trying to render an object (the event object) as a React child, which is not allowed.
🛠️ How to fix it?
There are a couple of ways to fix this error.
The first solution is to update the bind
method and change it to an arrow function within the map
function. Like this:
render() {
const items = ['EN', 'IT', 'FR', 'GR', 'RU'].map((item) => {
return (<li onClick={(e) => this.onItemClick(e, item)} key={item}>{item}</li>);
});
return (
<div>
...
<ul>
{items}
</ul>
...
</div>
);
}
By using an arrow function instead of the bind
method, we explicitly pass the event object e
as the first argument to the onItemClick
function, followed by the item
as the second argument.
Another solution is to update the onItemClick
function to accept only one argument, which is the event object. Then, you can access the item
value from the event target instead of passing it as an argument:
onItemClick(e) {
const item = e.target.innerText;
this.setState({
lang: item,
});
}
With this adjustment, you can remove the item
argument from the bind
or arrow function:
render() {
const items = ['EN', 'IT', 'FR', 'GR', 'RU'].map((item) => {
return (<li onClick={this.onItemClick} key={item}>{item}</li>);
});
return (
<div>
...
<ul>
{items}
</ul>
...
</div>
);
}
Choose the solution that best fits your needs and preferences.
📣 Call to action: Let's engage!
I hope this article helped you understand and solve the "Invariant Violation: Objects are not valid as a React child" error. If you have any questions or suggestions, please leave a comment below. I would love to hear from you!
Additionally, if you found this article helpful, don't hesitate to share it with your friends and colleagues. Together, we can make React development easier for everyone! 💪😄