In reactJS, how to copy text to clipboard?
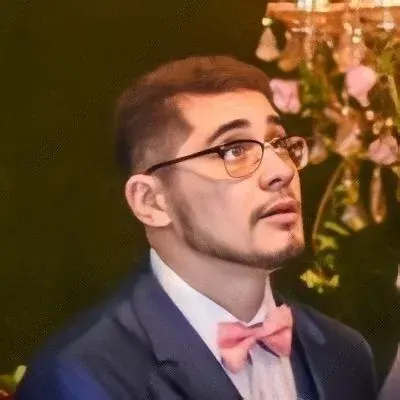
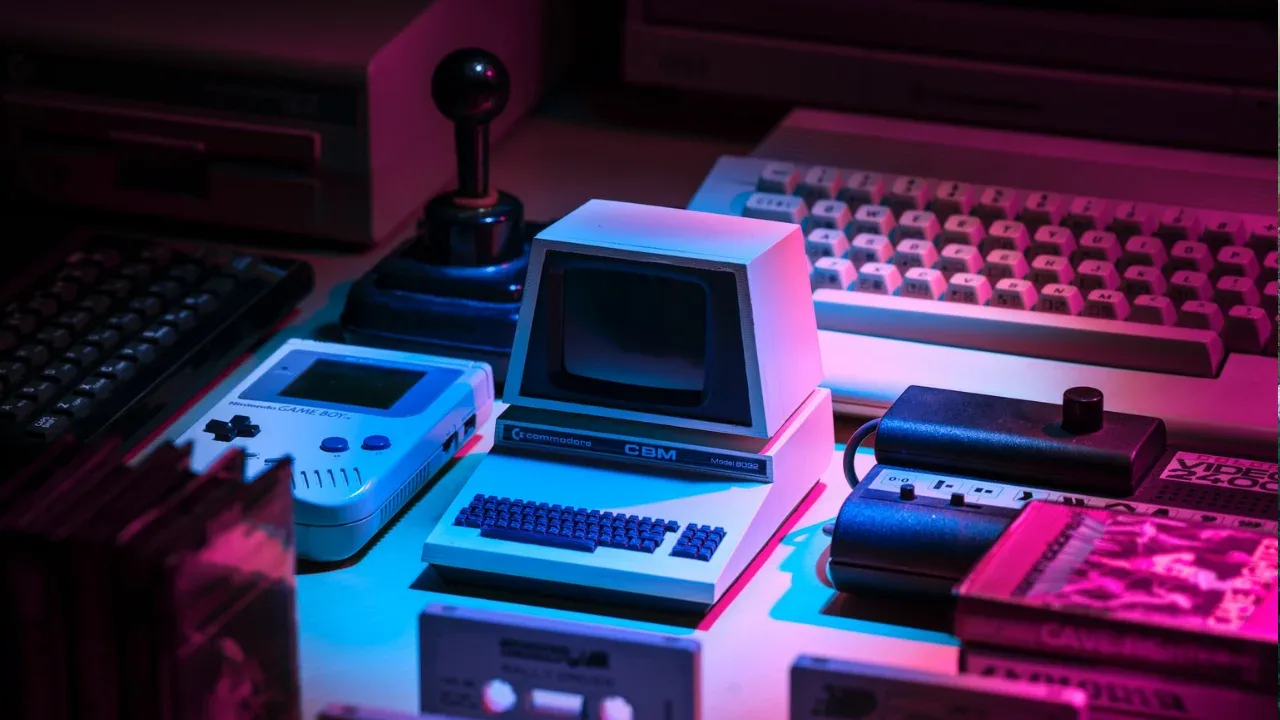
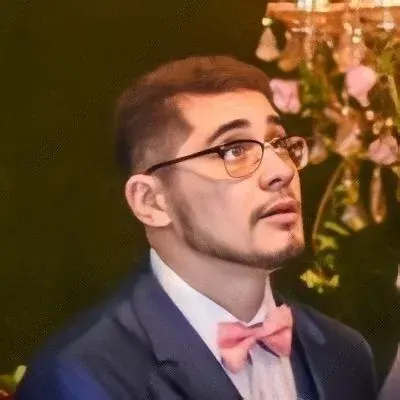
📋 How to Copy Text to Clipboard in ReactJS 📋
Hi there, ReactJS enthusiast! Have you ever wondered how to implement a copy to clipboard functionality in your ReactJS application? Well, you're in luck because I'm here to help you out! 😄
📝 The Problem
In ReactJS, copying text to the clipboard can be a bit tricky, especially considering different browser compatibility issues. But worry not, my friend! I'll guide you through a simple implementation that works perfectly on Chrome 52, as mentioned in your context.
💡 The Solution
To copy text to the clipboard in ReactJS, we need to follow a series of steps. Let's break it down:
Create a function (e.g.,
copyToClipboard
) in your React component.copyToClipboard = (text) => { // Code goes here... }
Inside the
copyToClipboard
function, create atextarea
element dynamically usingdocument.createElement('textarea')
.var textField = document.createElement('textarea');
Set the
innerText
of thetextarea
to the text you want to copy.textField.innerText = text;
Append the
textarea
to the document body usingdocument.body.appendChild(textField)
.Select the text inside the
textarea
usingtextField.select()
.Execute the copy command using
document.execCommand('copy')
.Finally, remove the
textarea
element from the document body usingtextField.remove()
.
Now your copyToClipboard
function should look like this:
copyToClipboard = (text) => {
var textField = document.createElement('textarea');
textField.innerText = text;
document.body.appendChild(textField);
textField.select();
document.execCommand('copy');
textField.remove();
}
With this implementation, the text will be copied to the clipboard when this function is called.
🏆 The Winning Approach
You might wonder why the code snippet you provided isn't working. Well, fear not! The missing piece of the puzzle is that you need to call the copyToClipboard
function with the desired text.
For example, let's say you have a link and want to copy its text when clicked:
<a href="#" onClick={() => this.copyToClipboard('Hello, World!')}>
Copy me to clipboard!
</a>
Here, the copyToClipboard
function is triggered when the link is clicked, and it copies the text 'Hello, World!' to the clipboard. Feel free to modify the text as per your requirements.
🙌 Spread the Word!
Congratulations! You now know how to copy text to the clipboard in ReactJS. Feel free to share this knowledge with fellow ReactJS developers who might find it useful. Spread the love for ReactJS and make copying to the clipboard a breeze!
That's all, folks! If you have any questions or face any issues, don't hesitate to leave a comment below. Happy coding! 😊🚀