Ignore Typescript Errors "property does not exist on value of type"
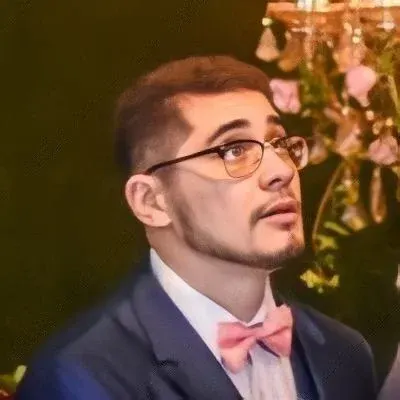
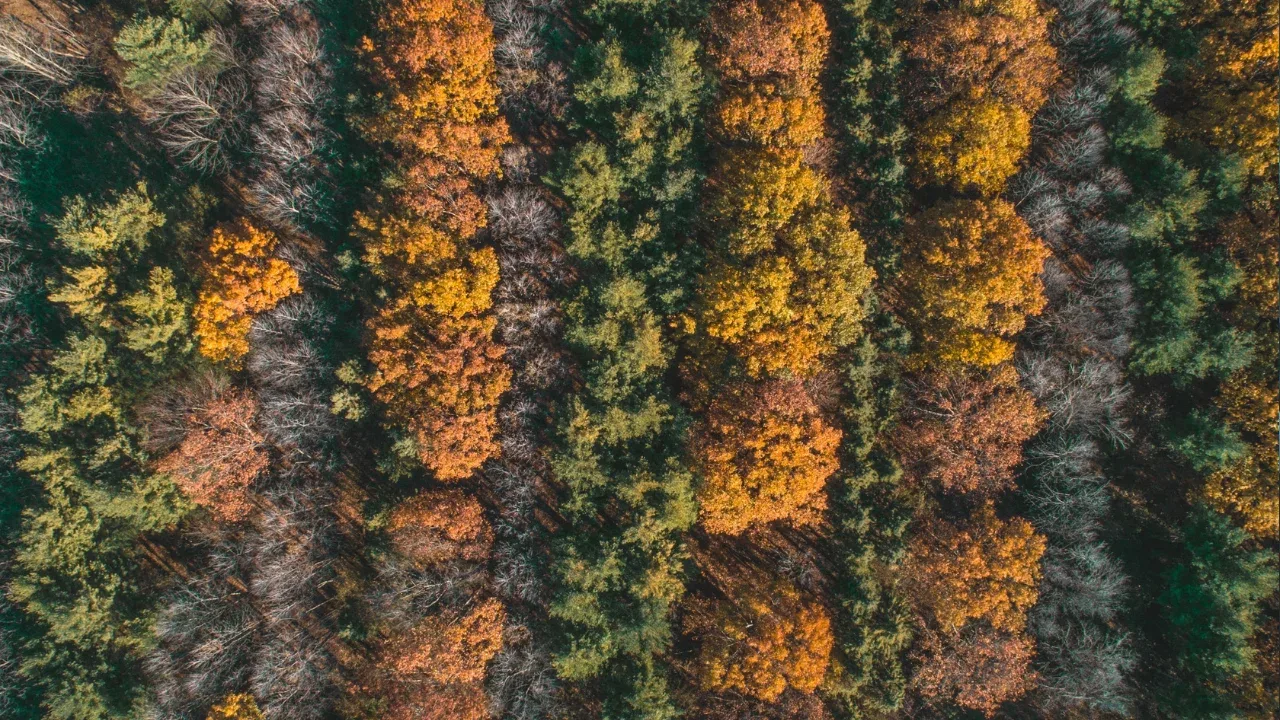
Ignoring Typescript Errors "property does not exist on value of type"
Are you tired of seeing those pesky Typescript errors about properties not existing on certain value types? 🤔 Don't worry, you're not alone! This is a common issue that many developers face when working with Typescript. But fear not, because in this blog post, we'll address this problem head-on and provide you with easy solutions to ignore those errors and keep your development flow going smoothly. 💪
Understanding the Context
Before we dive into the solutions, let's quickly understand the context behind this question. In Visual Studio 2013, building your project stops whenever the Typescript compiler (tsc.exe) exits with code 1. This behavior was not present in Visual Studio 2012. So, if you're using VS2013 or a newer version and encountering this error, keep reading! 👓
Handling the "property does not exist on value of type" Errors
Now, let's tackle those pesky "property does not exist on value of type" errors. These errors usually occur when working with Typescript interfaces or complex types. Here's how you can handle them:
Solution #1: Use the "as" Assertion
One way to bypass these errors is by using the "as" assertion in Typescript. This assertion is used to tell the compiler that you know more about the type than it does. Here's an example:
const myObject: any = { x: 1, y: 2 };
const result = (myObject as any).z; // Ignoring the error
console.log(result); // Output: undefined
By using the "as any" assertion, we're essentially telling the compiler to treat the myObject
variable as any type, allowing us to access properties that don't exist.
Solution #2: Use the "!" Non-null Assertion Operator
Another way to handle these errors is by using the non-null assertion operator "!". This operator is used to inform the compiler that a specific property won't be null or undefined. Here's an example:
const myObject: { x?: number } = { y: 2 };
const result = myObject.x!; // Ignoring the error
console.log(result); // Output: undefined
In this example, we're asserting that the x
property won't be null or undefined, effectively bypassing the error. However, be cautious when using this approach, as it might introduce runtime errors if the property does turn out to be null or undefined.
Solution #3: Disable Strict Null Checking
If you're consistently encountering these errors throughout your project, you can disable strict null checking in your Typescript configuration. However, this approach should be used with caution, as it might lead to potential runtime errors. Here's how you can disable strict null checking:
Locate your
tsconfig.json
file in the root of your project.Add
"strictNullChecks": false
to thecompilerOptions
section.Save the file and restart your build process.
Remember to weigh the trade-offs of disabling strict null checking before implementing this solution.
Let's Keep the Conversation Going! 💬
Now that you have some handy solutions to handle the "property does not exist on value of type" errors, it's time to put them into action! Have you encountered other Typescript errors that you'd like to know how to handle? Share your experiences and let's help each other out! Leave a comment below and let's keep the conversation going. 👇
Happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
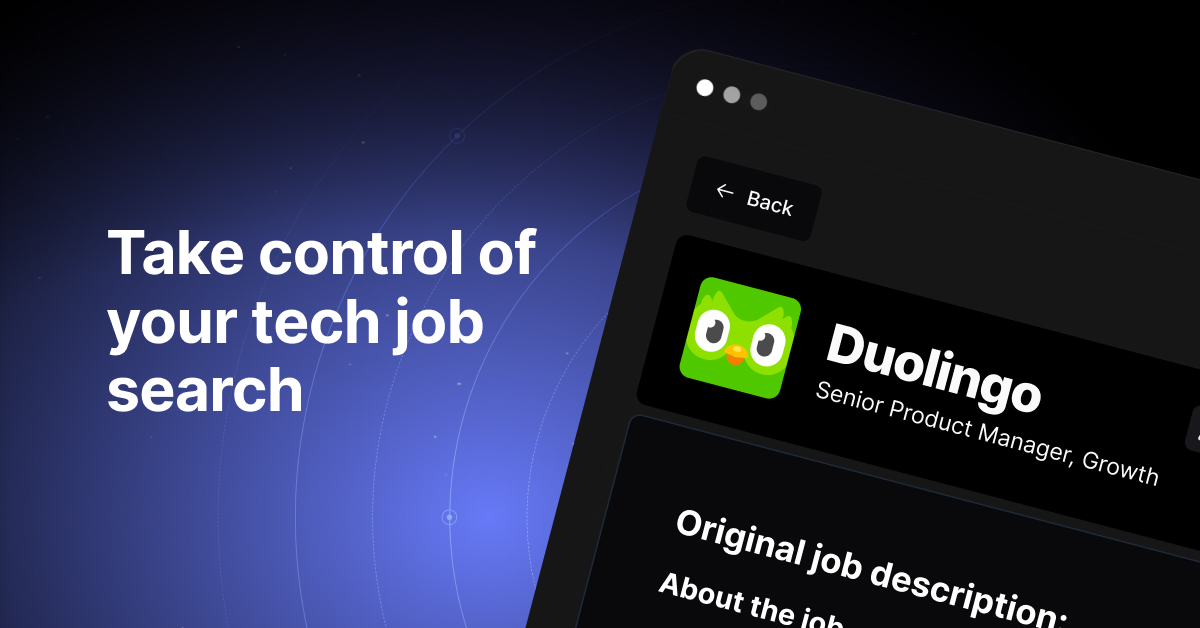