I want to remove double quotes from a String
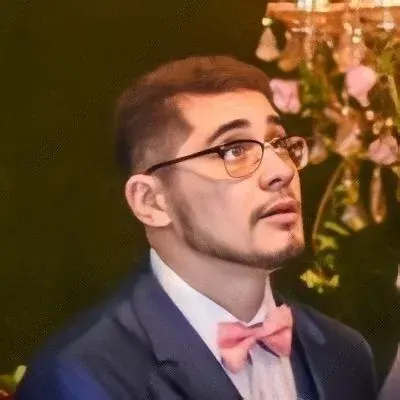
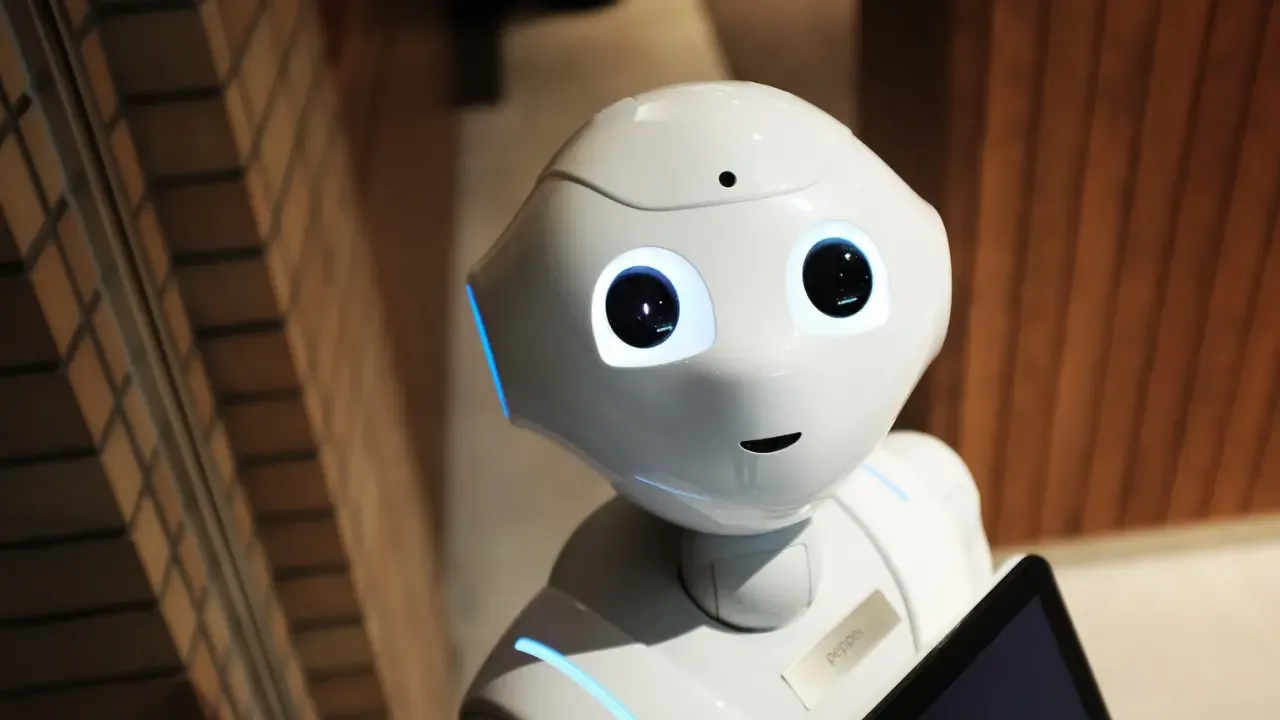
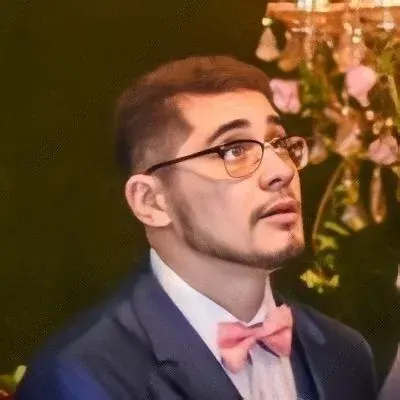
Removing Double Quotes From a String: A Quick Guide! ✨
Have you ever found yourself in a situation where you need to remove those pesky double quotes from a string? Whether you're a seasoned developer or just starting out, dealing with string manipulation can be a bit tricky. But fear not! In this article, we will walk you through some easy solutions to remove those unwanted double quotes from a string. Let's dive in! 💪
The Problem: Removing Double Quotes
So, you have a string surrounded by double quotes, and you want to extract the string without those quotes. For example, if your string is:
"I am here"
You want to output:
I am here
Solution 1: Using String Replace
One simple way to remove the double quotes is by using the String.replace()
method. This method allows you to replace a specific substring with another substring. In this case, we can replace the double quotes with an empty string.
String input = "\"I am here\"";
String output = input.replace("\"", "");
System.out.println(output);
The replace()
method takes two parameters: the substring to be replaced (in our case, the double quotes represented by \"
) and the replacement substring (an empty string in our case). Running this code will produce the desired output: I am here
.
Solution 2: Using Regular Expressions
If you're dealing with more complex cases where the double quotes are present at different locations within the string, regular expressions can be a handy tool. Regular expressions allow us to pattern match and replace specific elements within a string.
String input = "\"I am here\"";
String output = input.replaceAll("\"", "");
System.out.println(output);
The replaceAll()
method takes a regular expression as the first parameter. In our case, we replace all occurrences of double quotes with an empty string. This will give us the same output as Solution 1: I am here
.
Conclusion
Removing double quotes from a string might seem like a daunting task, but it doesn't have to be. By using the String.replace()
method or regular expressions, you can easily extract the desired string without those pesky quotes.
Feel free to experiment with the provided code examples and explore other string manipulation techniques. And remember, incorporating these techniques into your code will help you write cleaner and more efficient programs.
Now it's your turn! Have you encountered any interesting challenges while manipulating strings? Share your experiences and tips in the comments below. Happy coding! 🚀